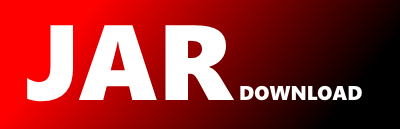
commonMain.aws.sdk.kotlin.services.glue.model.Field.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glue-jvm Show documentation
Show all versions of glue-jvm Show documentation
The AWS SDK for Kotlin client for Glue
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glue.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The `Field` object has information about the different properties associated with a field in the connector.
*/
public class Field private constructor(builder: Builder) {
/**
* Optional map of keys which may be returned.
*/
public val customProperties: Map? = builder.customProperties
/**
* A description of the field.
*/
public val description: kotlin.String? = builder.description
/**
* A unique identifier for the field.
*/
public val fieldName: kotlin.String? = builder.fieldName
/**
* The type of data in the field.
*/
public val fieldType: aws.sdk.kotlin.services.glue.model.FieldDataType? = builder.fieldType
/**
* Indicates whether this field can be created as part of a destination write.
*/
public val isCreateable: kotlin.Boolean? = builder.isCreateable
/**
* Indicates whether this field is populated automatically when the object is created, such as a created at timestamp.
*/
public val isDefaultOnCreate: kotlin.Boolean? = builder.isDefaultOnCreate
/**
* Indicates whether this field can used in a filter clause (`WHERE` clause) of a SQL statement when querying data.
*/
public val isFilterable: kotlin.Boolean? = builder.isFilterable
/**
* Indicates whether this field can be nullable or not.
*/
public val isNullable: kotlin.Boolean? = builder.isNullable
/**
* Indicates whether a given field can be used in partitioning the query made to SaaS.
*/
public val isPartitionable: kotlin.Boolean? = builder.isPartitionable
/**
* Indicates whether this field can used as a primary key for the given entity.
*/
public val isPrimaryKey: kotlin.Boolean? = builder.isPrimaryKey
/**
* Indicates whether this field can be added in Select clause of SQL query or whether it is retrievable or not.
*/
public val isRetrievable: kotlin.Boolean? = builder.isRetrievable
/**
* Indicates whether this field can be updated as part of a destination write.
*/
public val isUpdateable: kotlin.Boolean? = builder.isUpdateable
/**
* Indicates whether this field can be upserted as part of a destination write.
*/
public val isUpsertable: kotlin.Boolean? = builder.isUpsertable
/**
* A readable label used for the field.
*/
public val label: kotlin.String? = builder.label
/**
* The data type returned by the SaaS API, such as “picklist” or “textarea” from Salesforce.
*/
public val nativeDataType: kotlin.String? = builder.nativeDataType
/**
* A parent field name for a nested field.
*/
public val parentField: kotlin.String? = builder.parentField
/**
* Indicates the support filter operators for this field.
*/
public val supportedFilterOperators: List? = builder.supportedFilterOperators
/**
* A list of supported values for the field.
*/
public val supportedValues: List? = builder.supportedValues
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glue.model.Field = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Field(")
append("customProperties=$customProperties,")
append("description=$description,")
append("fieldName=$fieldName,")
append("fieldType=$fieldType,")
append("isCreateable=$isCreateable,")
append("isDefaultOnCreate=$isDefaultOnCreate,")
append("isFilterable=$isFilterable,")
append("isNullable=$isNullable,")
append("isPartitionable=$isPartitionable,")
append("isPrimaryKey=$isPrimaryKey,")
append("isRetrievable=$isRetrievable,")
append("isUpdateable=$isUpdateable,")
append("isUpsertable=$isUpsertable,")
append("label=$label,")
append("nativeDataType=$nativeDataType,")
append("parentField=$parentField,")
append("supportedFilterOperators=$supportedFilterOperators,")
append("supportedValues=$supportedValues")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = customProperties?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (fieldName?.hashCode() ?: 0)
result = 31 * result + (fieldType?.hashCode() ?: 0)
result = 31 * result + (isCreateable?.hashCode() ?: 0)
result = 31 * result + (isDefaultOnCreate?.hashCode() ?: 0)
result = 31 * result + (isFilterable?.hashCode() ?: 0)
result = 31 * result + (isNullable?.hashCode() ?: 0)
result = 31 * result + (isPartitionable?.hashCode() ?: 0)
result = 31 * result + (isPrimaryKey?.hashCode() ?: 0)
result = 31 * result + (isRetrievable?.hashCode() ?: 0)
result = 31 * result + (isUpdateable?.hashCode() ?: 0)
result = 31 * result + (isUpsertable?.hashCode() ?: 0)
result = 31 * result + (label?.hashCode() ?: 0)
result = 31 * result + (nativeDataType?.hashCode() ?: 0)
result = 31 * result + (parentField?.hashCode() ?: 0)
result = 31 * result + (supportedFilterOperators?.hashCode() ?: 0)
result = 31 * result + (supportedValues?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Field
if (customProperties != other.customProperties) return false
if (description != other.description) return false
if (fieldName != other.fieldName) return false
if (fieldType != other.fieldType) return false
if (isCreateable != other.isCreateable) return false
if (isDefaultOnCreate != other.isDefaultOnCreate) return false
if (isFilterable != other.isFilterable) return false
if (isNullable != other.isNullable) return false
if (isPartitionable != other.isPartitionable) return false
if (isPrimaryKey != other.isPrimaryKey) return false
if (isRetrievable != other.isRetrievable) return false
if (isUpdateable != other.isUpdateable) return false
if (isUpsertable != other.isUpsertable) return false
if (label != other.label) return false
if (nativeDataType != other.nativeDataType) return false
if (parentField != other.parentField) return false
if (supportedFilterOperators != other.supportedFilterOperators) return false
if (supportedValues != other.supportedValues) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glue.model.Field = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Optional map of keys which may be returned.
*/
public var customProperties: Map? = null
/**
* A description of the field.
*/
public var description: kotlin.String? = null
/**
* A unique identifier for the field.
*/
public var fieldName: kotlin.String? = null
/**
* The type of data in the field.
*/
public var fieldType: aws.sdk.kotlin.services.glue.model.FieldDataType? = null
/**
* Indicates whether this field can be created as part of a destination write.
*/
public var isCreateable: kotlin.Boolean? = null
/**
* Indicates whether this field is populated automatically when the object is created, such as a created at timestamp.
*/
public var isDefaultOnCreate: kotlin.Boolean? = null
/**
* Indicates whether this field can used in a filter clause (`WHERE` clause) of a SQL statement when querying data.
*/
public var isFilterable: kotlin.Boolean? = null
/**
* Indicates whether this field can be nullable or not.
*/
public var isNullable: kotlin.Boolean? = null
/**
* Indicates whether a given field can be used in partitioning the query made to SaaS.
*/
public var isPartitionable: kotlin.Boolean? = null
/**
* Indicates whether this field can used as a primary key for the given entity.
*/
public var isPrimaryKey: kotlin.Boolean? = null
/**
* Indicates whether this field can be added in Select clause of SQL query or whether it is retrievable or not.
*/
public var isRetrievable: kotlin.Boolean? = null
/**
* Indicates whether this field can be updated as part of a destination write.
*/
public var isUpdateable: kotlin.Boolean? = null
/**
* Indicates whether this field can be upserted as part of a destination write.
*/
public var isUpsertable: kotlin.Boolean? = null
/**
* A readable label used for the field.
*/
public var label: kotlin.String? = null
/**
* The data type returned by the SaaS API, such as “picklist” or “textarea” from Salesforce.
*/
public var nativeDataType: kotlin.String? = null
/**
* A parent field name for a nested field.
*/
public var parentField: kotlin.String? = null
/**
* Indicates the support filter operators for this field.
*/
public var supportedFilterOperators: List? = null
/**
* A list of supported values for the field.
*/
public var supportedValues: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glue.model.Field) : this() {
this.customProperties = x.customProperties
this.description = x.description
this.fieldName = x.fieldName
this.fieldType = x.fieldType
this.isCreateable = x.isCreateable
this.isDefaultOnCreate = x.isDefaultOnCreate
this.isFilterable = x.isFilterable
this.isNullable = x.isNullable
this.isPartitionable = x.isPartitionable
this.isPrimaryKey = x.isPrimaryKey
this.isRetrievable = x.isRetrievable
this.isUpdateable = x.isUpdateable
this.isUpsertable = x.isUpsertable
this.label = x.label
this.nativeDataType = x.nativeDataType
this.parentField = x.parentField
this.supportedFilterOperators = x.supportedFilterOperators
this.supportedValues = x.supportedValues
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glue.model.Field = Field(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy