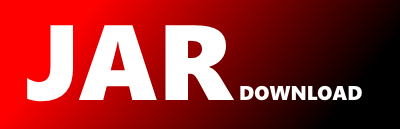
commonMain.aws.sdk.kotlin.services.iot.model.OpenSearchAction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iot Show documentation
Show all versions of iot Show documentation
The AWS SDK for Kotlin client for IoT
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iot.model
/**
* Describes an action that writes data to an Amazon OpenSearch Service domain.
*/
public class OpenSearchAction private constructor(builder: Builder) {
/**
* The endpoint of your OpenSearch domain.
*/
public val endpoint: kotlin.String? = builder.endpoint
/**
* The unique identifier for the document you are storing.
*/
public val id: kotlin.String? = builder.id
/**
* The OpenSearch index where you want to store your data.
*/
public val index: kotlin.String? = builder.index
/**
* The IAM role ARN that has access to OpenSearch.
*/
public val roleArn: kotlin.String? = builder.roleArn
/**
* The type of document you are storing.
*/
public val type: kotlin.String? = builder.type
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iot.model.OpenSearchAction = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("OpenSearchAction(")
append("endpoint=$endpoint,")
append("id=$id,")
append("index=$index,")
append("roleArn=$roleArn,")
append("type=$type")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = endpoint?.hashCode() ?: 0
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (index?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as OpenSearchAction
if (endpoint != other.endpoint) return false
if (id != other.id) return false
if (index != other.index) return false
if (roleArn != other.roleArn) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iot.model.OpenSearchAction = Builder(this).apply(block).build()
public class Builder {
/**
* The endpoint of your OpenSearch domain.
*/
public var endpoint: kotlin.String? = null
/**
* The unique identifier for the document you are storing.
*/
public var id: kotlin.String? = null
/**
* The OpenSearch index where you want to store your data.
*/
public var index: kotlin.String? = null
/**
* The IAM role ARN that has access to OpenSearch.
*/
public var roleArn: kotlin.String? = null
/**
* The type of document you are storing.
*/
public var type: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iot.model.OpenSearchAction) : this() {
this.endpoint = x.endpoint
this.id = x.id
this.index = x.index
this.roleArn = x.roleArn
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iot.model.OpenSearchAction = OpenSearchAction(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy