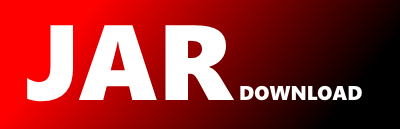
commonMain.aws.sdk.kotlin.services.iot.model.TaskStatistics.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iot.model
/**
* Statistics for the checks performed during the audit.
*/
public class TaskStatistics private constructor(builder: Builder) {
/**
* The number of checks that did not run because the audit was canceled.
*/
public val canceledChecks: kotlin.Int? = builder.canceledChecks
/**
* The number of checks that found compliant resources.
*/
public val compliantChecks: kotlin.Int? = builder.compliantChecks
/**
* The number of checks.
*/
public val failedChecks: kotlin.Int? = builder.failedChecks
/**
* The number of checks in progress.
*/
public val inProgressChecks: kotlin.Int? = builder.inProgressChecks
/**
* The number of checks that found noncompliant resources.
*/
public val nonCompliantChecks: kotlin.Int? = builder.nonCompliantChecks
/**
* The number of checks in this audit.
*/
public val totalChecks: kotlin.Int? = builder.totalChecks
/**
* The number of checks waiting for data collection.
*/
public val waitingForDataCollectionChecks: kotlin.Int? = builder.waitingForDataCollectionChecks
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iot.model.TaskStatistics = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TaskStatistics(")
append("canceledChecks=$canceledChecks,")
append("compliantChecks=$compliantChecks,")
append("failedChecks=$failedChecks,")
append("inProgressChecks=$inProgressChecks,")
append("nonCompliantChecks=$nonCompliantChecks,")
append("totalChecks=$totalChecks,")
append("waitingForDataCollectionChecks=$waitingForDataCollectionChecks")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = canceledChecks ?: 0
result = 31 * result + (compliantChecks ?: 0)
result = 31 * result + (failedChecks ?: 0)
result = 31 * result + (inProgressChecks ?: 0)
result = 31 * result + (nonCompliantChecks ?: 0)
result = 31 * result + (totalChecks ?: 0)
result = 31 * result + (waitingForDataCollectionChecks ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TaskStatistics
if (canceledChecks != other.canceledChecks) return false
if (compliantChecks != other.compliantChecks) return false
if (failedChecks != other.failedChecks) return false
if (inProgressChecks != other.inProgressChecks) return false
if (nonCompliantChecks != other.nonCompliantChecks) return false
if (totalChecks != other.totalChecks) return false
if (waitingForDataCollectionChecks != other.waitingForDataCollectionChecks) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iot.model.TaskStatistics = Builder(this).apply(block).build()
public class Builder {
/**
* The number of checks that did not run because the audit was canceled.
*/
public var canceledChecks: kotlin.Int? = null
/**
* The number of checks that found compliant resources.
*/
public var compliantChecks: kotlin.Int? = null
/**
* The number of checks.
*/
public var failedChecks: kotlin.Int? = null
/**
* The number of checks in progress.
*/
public var inProgressChecks: kotlin.Int? = null
/**
* The number of checks that found noncompliant resources.
*/
public var nonCompliantChecks: kotlin.Int? = null
/**
* The number of checks in this audit.
*/
public var totalChecks: kotlin.Int? = null
/**
* The number of checks waiting for data collection.
*/
public var waitingForDataCollectionChecks: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iot.model.TaskStatistics) : this() {
this.canceledChecks = x.canceledChecks
this.compliantChecks = x.compliantChecks
this.failedChecks = x.failedChecks
this.inProgressChecks = x.inProgressChecks
this.nonCompliantChecks = x.nonCompliantChecks
this.totalChecks = x.totalChecks
this.waitingForDataCollectionChecks = x.waitingForDataCollectionChecks
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iot.model.TaskStatistics = TaskStatistics(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy