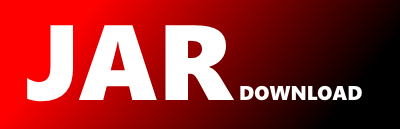
commonMain.aws.sdk.kotlin.services.iotdataplane.DefaultIotDataPlaneClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdataplane-jvm Show documentation
Show all versions of iotdataplane-jvm Show documentation
The AWS SDK for Kotlin client for IoT Data Plane
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotdataplane
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.interceptors.AwsSpanInterceptor
import aws.sdk.kotlin.runtime.http.interceptors.BusinessMetricsInterceptor
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.iotdataplane.auth.IotDataPlaneAuthSchemeProviderAdapter
import aws.sdk.kotlin.services.iotdataplane.auth.IotDataPlaneIdentityProviderConfigAdapter
import aws.sdk.kotlin.services.iotdataplane.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.iotdataplane.model.*
import aws.sdk.kotlin.services.iotdataplane.serde.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.awsprotocol.AwsAttributes
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.collections.attributesOf
import aws.smithy.kotlin.runtime.collections.putIfAbsent
import aws.smithy.kotlin.runtime.collections.putIfAbsentNotNull
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.AuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.OperationMetrics
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.telemetry
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
internal class DefaultIotDataPlaneClient(override val config: IotDataPlaneClient.Config) : IotDataPlaneClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = IotDataPlaneIdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(AuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "iotdata")
}
toMap()
}
private val authSchemeAdapter = IotDataPlaneAuthSchemeProviderAdapter(config)
private val telemetryScope = "aws.sdk.kotlin.services.iotdataplane"
private val opMetrics = OperationMetrics(telemetryScope, config.telemetryProvider)
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion), config.applicationId)
/**
* Deletes the shadow for the specified thing.
*
* Requires permission to access the [DeleteThingShadow](https://docs.aws.amazon.com/service-authorization/latest/reference/list_awsiot.html#awsiot-actions-as-permissions) action.
*
* For more information, see [DeleteThingShadow](http://docs.aws.amazon.com/iot/latest/developerguide/API_DeleteThingShadow.html) in the IoT Developer Guide.
*/
override suspend fun deleteThingShadow(input: DeleteThingShadowRequest): DeleteThingShadowResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteThingShadowOperationSerializer()
deserializeWith = DeleteThingShadowOperationDeserializer()
operationName = "DeleteThingShadow"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Gets the details of a single retained message for the specified topic.
*
* This action returns the message payload of the retained message, which can incur messaging costs. To list only the topic names of the retained messages, call [ListRetainedMessages](https://docs.aws.amazon.com/iot/latest/apireference/API_iotdata_ListRetainedMessages.html).
*
* Requires permission to access the [GetRetainedMessage](https://docs.aws.amazon.com/service-authorization/latest/reference/list_awsiotfleethubfordevicemanagement.html#awsiotfleethubfordevicemanagement-actions-as-permissions) action.
*
* For more information about messaging costs, see [Amazon Web Services IoT Core pricing - Messaging](http://aws.amazon.com/iot-core/pricing/#Messaging).
*/
override suspend fun getRetainedMessage(input: GetRetainedMessageRequest): GetRetainedMessageResponse {
val op = SdkHttpOperation.build {
serializeWith = GetRetainedMessageOperationSerializer()
deserializeWith = GetRetainedMessageOperationDeserializer()
operationName = "GetRetainedMessage"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Gets the shadow for the specified thing.
*
* Requires permission to access the [GetThingShadow](https://docs.aws.amazon.com/service-authorization/latest/reference/list_awsiot.html#awsiot-actions-as-permissions) action.
*
* For more information, see [GetThingShadow](http://docs.aws.amazon.com/iot/latest/developerguide/API_GetThingShadow.html) in the IoT Developer Guide.
*/
override suspend fun getThingShadow(input: GetThingShadowRequest): GetThingShadowResponse {
val op = SdkHttpOperation.build {
serializeWith = GetThingShadowOperationSerializer()
deserializeWith = GetThingShadowOperationDeserializer()
operationName = "GetThingShadow"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists the shadows for the specified thing.
*
* Requires permission to access the [ListNamedShadowsForThing](https://docs.aws.amazon.com/service-authorization/latest/reference/list_awsiot.html#awsiot-actions-as-permissions) action.
*/
override suspend fun listNamedShadowsForThing(input: ListNamedShadowsForThingRequest): ListNamedShadowsForThingResponse {
val op = SdkHttpOperation.build {
serializeWith = ListNamedShadowsForThingOperationSerializer()
deserializeWith = ListNamedShadowsForThingOperationDeserializer()
operationName = "ListNamedShadowsForThing"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists summary information about the retained messages stored for the account.
*
* This action returns only the topic names of the retained messages. It doesn't return any message payloads. Although this action doesn't return a message payload, it can still incur messaging costs.
*
* To get the message payload of a retained message, call [GetRetainedMessage](https://docs.aws.amazon.com/iot/latest/apireference/API_iotdata_GetRetainedMessage.html) with the topic name of the retained message.
*
* Requires permission to access the [ListRetainedMessages](https://docs.aws.amazon.com/service-authorization/latest/reference/list_awsiotfleethubfordevicemanagement.html#awsiotfleethubfordevicemanagement-actions-as-permissions) action.
*
* For more information about messaging costs, see [Amazon Web Services IoT Core pricing - Messaging](http://aws.amazon.com/iot-core/pricing/#Messaging).
*/
override suspend fun listRetainedMessages(input: ListRetainedMessagesRequest): ListRetainedMessagesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListRetainedMessagesOperationSerializer()
deserializeWith = ListRetainedMessagesOperationDeserializer()
operationName = "ListRetainedMessages"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Publishes an MQTT message.
*
* Requires permission to access the [Publish](https://docs.aws.amazon.com/service-authorization/latest/reference/list_awsiot.html#awsiot-actions-as-permissions) action.
*
* For more information about MQTT messages, see [MQTT Protocol](http://docs.aws.amazon.com/iot/latest/developerguide/mqtt.html) in the IoT Developer Guide.
*
* For more information about messaging costs, see [Amazon Web Services IoT Core pricing - Messaging](http://aws.amazon.com/iot-core/pricing/#Messaging).
*/
override suspend fun publish(input: PublishRequest): PublishResponse {
val op = SdkHttpOperation.build {
serializeWith = PublishOperationSerializer()
deserializeWith = PublishOperationDeserializer()
operationName = "Publish"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the shadow for the specified thing.
*
* Requires permission to access the [UpdateThingShadow](https://docs.aws.amazon.com/service-authorization/latest/reference/list_awsiot.html#awsiot-actions-as-permissions) action.
*
* For more information, see [UpdateThingShadow](http://docs.aws.amazon.com/iot/latest/developerguide/API_UpdateThingShadow.html) in the IoT Developer Guide.
*/
override suspend fun updateThingShadow(input: UpdateThingShadowRequest): UpdateThingShadowResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateThingShadowOperationSerializer()
deserializeWith = UpdateThingShadowOperationDeserializer()
operationName = "UpdateThingShadow"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsentNotNull(AwsAttributes.Region, config.region)
ctx.putIfAbsentNotNull(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "iotdata")
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy