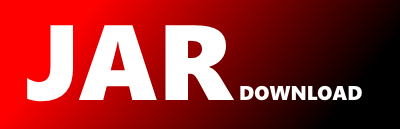
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.CanSignal.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information about a single controller area network (CAN) signal and the messages it receives and transmits.
*/
public class CanSignal private constructor(builder: Builder) {
/**
* A multiplier used to decode the CAN message.
*/
public val factor: kotlin.Double = requireNotNull(builder.factor) { "A non-null value must be provided for factor" }
/**
* Whether the byte ordering of a CAN message is big-endian.
*/
public val isBigEndian: kotlin.Boolean = builder.isBigEndian
/**
* Whether the message data is specified as a signed value.
*/
public val isSigned: kotlin.Boolean = builder.isSigned
/**
* How many bytes of data are in the message.
*/
public val length: kotlin.Int = builder.length
/**
* The ID of the message.
*/
public val messageId: kotlin.Int = builder.messageId
/**
* The name of the signal.
*/
public val name: kotlin.String? = builder.name
/**
* The offset used to calculate the signal value. Combined with factor, the calculation is `value = raw_value * factor + offset`.
*/
public val offset: kotlin.Double = requireNotNull(builder.offset) { "A non-null value must be provided for offset" }
/**
* Indicates the beginning of the CAN signal. This should always be the least significant bit (LSB).
*
* This value might be different from the value in a DBC file. For little endian signals, `startBit` is the same value as in the DBC file. For big endian signals in a DBC file, the start bit is the most significant bit (MSB). You will have to calculate the LSB instead and pass it as the `startBit`.
*/
public val startBit: kotlin.Int = builder.startBit
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.CanSignal = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CanSignal(")
append("factor=$factor,")
append("isBigEndian=$isBigEndian,")
append("isSigned=$isSigned,")
append("length=$length,")
append("messageId=$messageId,")
append("name=$name,")
append("offset=$offset,")
append("startBit=$startBit")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = factor.hashCode()
result = 31 * result + (isBigEndian.hashCode())
result = 31 * result + (isSigned.hashCode())
result = 31 * result + (length)
result = 31 * result + (messageId)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (offset.hashCode())
result = 31 * result + (startBit)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CanSignal
if (!(factor?.equals(other.factor) ?: (other.factor == null))) return false
if (isBigEndian != other.isBigEndian) return false
if (isSigned != other.isSigned) return false
if (length != other.length) return false
if (messageId != other.messageId) return false
if (name != other.name) return false
if (!(offset?.equals(other.offset) ?: (other.offset == null))) return false
if (startBit != other.startBit) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.CanSignal = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A multiplier used to decode the CAN message.
*/
public var factor: kotlin.Double? = null
/**
* Whether the byte ordering of a CAN message is big-endian.
*/
public var isBigEndian: kotlin.Boolean = false
/**
* Whether the message data is specified as a signed value.
*/
public var isSigned: kotlin.Boolean = false
/**
* How many bytes of data are in the message.
*/
public var length: kotlin.Int = 0
/**
* The ID of the message.
*/
public var messageId: kotlin.Int = 0
/**
* The name of the signal.
*/
public var name: kotlin.String? = null
/**
* The offset used to calculate the signal value. Combined with factor, the calculation is `value = raw_value * factor + offset`.
*/
public var offset: kotlin.Double? = null
/**
* Indicates the beginning of the CAN signal. This should always be the least significant bit (LSB).
*
* This value might be different from the value in a DBC file. For little endian signals, `startBit` is the same value as in the DBC file. For big endian signals in a DBC file, the start bit is the most significant bit (MSB). You will have to calculate the LSB instead and pass it as the `startBit`.
*/
public var startBit: kotlin.Int = 0
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.CanSignal) : this() {
this.factor = x.factor
this.isBigEndian = x.isBigEndian
this.isSigned = x.isSigned
this.length = x.length
this.messageId = x.messageId
this.name = x.name
this.offset = x.offset
this.startBit = x.startBit
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.CanSignal = CanSignal(this)
internal fun correctErrors(): Builder {
if (factor == null) factor = 0.0
if (offset == null) offset = 0.0
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy