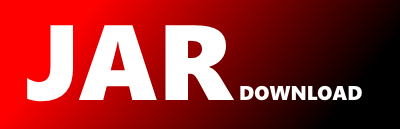
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.CreateCampaignRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class CreateCampaignRequest private constructor(builder: Builder) {
/**
* The data collection scheme associated with the campaign. You can specify a scheme that collects data based on time or an event.
*/
public val collectionScheme: aws.sdk.kotlin.services.iotfleetwise.model.CollectionScheme? = builder.collectionScheme
/**
* (Optional) Whether to compress signals before transmitting data to Amazon Web Services IoT FleetWise. If you don't want to compress the signals, use `OFF`. If it's not specified, `SNAPPY` is used.
*
* Default: `SNAPPY`
*/
public val compression: aws.sdk.kotlin.services.iotfleetwise.model.Compression? = builder.compression
/**
* The destination where the campaign sends data. You can choose to send data to be stored in Amazon S3 or Amazon Timestream.
*
* Amazon S3 optimizes the cost of data storage and provides additional mechanisms to use vehicle data, such as data lakes, centralized data storage, data processing pipelines, and analytics. Amazon Web Services IoT FleetWise supports at-least-once file delivery to S3. Your vehicle data is stored on multiple Amazon Web Services IoT FleetWise servers for redundancy and high availability.
*
* You can use Amazon Timestream to access and analyze time series data, and Timestream to query vehicle data so that you can identify trends and patterns.
*/
public val dataDestinationConfigs: List? = builder.dataDestinationConfigs
/**
* (Optional) A list of vehicle attributes to associate with a campaign.
*
* Enrich the data with specified vehicle attributes. For example, add `make` and `model` to the campaign, and Amazon Web Services IoT FleetWise will associate the data with those attributes as dimensions in Amazon Timestream. You can then query the data against `make` and `model`.
*
* Default: An empty array
*/
public val dataExtraDimensions: List? = builder.dataExtraDimensions
/**
* An optional description of the campaign to help identify its purpose.
*/
public val description: kotlin.String? = builder.description
/**
* (Optional) Option for a vehicle to send diagnostic trouble codes to Amazon Web Services IoT FleetWise. If you want to send diagnostic trouble codes, use `SEND_ACTIVE_DTCS`. If it's not specified, `OFF` is used.
*
* Default: `OFF`
*/
public val diagnosticsMode: aws.sdk.kotlin.services.iotfleetwise.model.DiagnosticsMode? = builder.diagnosticsMode
/**
* (Optional) The time the campaign expires, in seconds since epoch (January 1, 1970 at midnight UTC time). Vehicle data isn't collected after the campaign expires.
*
* Default: 253402214400 (December 31, 9999, 00:00:00 UTC)
*/
public val expiryTime: aws.smithy.kotlin.runtime.time.Instant? = builder.expiryTime
/**
* The name of the campaign to create.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* (Optional) How long (in milliseconds) to collect raw data after a triggering event initiates the collection. If it's not specified, `0` is used.
*
* Default: `0`
*/
public val postTriggerCollectionDuration: kotlin.Long? = builder.postTriggerCollectionDuration
/**
* (Optional) A number indicating the priority of one campaign over another campaign for a certain vehicle or fleet. A campaign with the lowest value is deployed to vehicles before any other campaigns. If it's not specified, `0` is used.
*
* Default: `0`
*/
@Deprecated("priority is no longer used or needed as input")
public val priority: kotlin.Int? = builder.priority
/**
* The Amazon Resource Name (ARN) of the signal catalog to associate with the campaign.
*/
public val signalCatalogArn: kotlin.String = requireNotNull(builder.signalCatalogArn) { "A non-null value must be provided for signalCatalogArn" }
/**
* (Optional) A list of information about signals to collect.
*/
public val signalsToCollect: List? = builder.signalsToCollect
/**
* (Optional) Whether to store collected data after a vehicle lost a connection with the cloud. After a connection is re-established, the data is automatically forwarded to Amazon Web Services IoT FleetWise. If you want to store collected data when a vehicle loses connection with the cloud, use `TO_DISK`. If it's not specified, `OFF` is used.
*
* Default: `OFF`
*/
public val spoolingMode: aws.sdk.kotlin.services.iotfleetwise.model.SpoolingMode? = builder.spoolingMode
/**
* (Optional) The time, in milliseconds, to deliver a campaign after it was approved. If it's not specified, `0` is used.
*
* Default: `0`
*/
public val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* Metadata that can be used to manage the campaign.
*/
public val tags: List? = builder.tags
/**
* The ARN of the vehicle or fleet to deploy a campaign to.
*/
public val targetArn: kotlin.String = requireNotNull(builder.targetArn) { "A non-null value must be provided for targetArn" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.CreateCampaignRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateCampaignRequest(")
append("collectionScheme=$collectionScheme,")
append("compression=$compression,")
append("dataDestinationConfigs=$dataDestinationConfigs,")
append("dataExtraDimensions=*** Sensitive Data Redacted ***,")
append("description=$description,")
append("diagnosticsMode=$diagnosticsMode,")
append("expiryTime=$expiryTime,")
append("name=$name,")
append("postTriggerCollectionDuration=$postTriggerCollectionDuration,")
append("priority=$priority,")
append("signalCatalogArn=$signalCatalogArn,")
append("signalsToCollect=*** Sensitive Data Redacted ***,")
append("spoolingMode=$spoolingMode,")
append("startTime=$startTime,")
append("tags=$tags,")
append("targetArn=$targetArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = collectionScheme?.hashCode() ?: 0
result = 31 * result + (compression?.hashCode() ?: 0)
result = 31 * result + (dataDestinationConfigs?.hashCode() ?: 0)
result = 31 * result + (dataExtraDimensions?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (diagnosticsMode?.hashCode() ?: 0)
result = 31 * result + (expiryTime?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (postTriggerCollectionDuration?.hashCode() ?: 0)
result = 31 * result + (priority ?: 0)
result = 31 * result + (signalCatalogArn.hashCode())
result = 31 * result + (signalsToCollect?.hashCode() ?: 0)
result = 31 * result + (spoolingMode?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (targetArn.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateCampaignRequest
if (collectionScheme != other.collectionScheme) return false
if (compression != other.compression) return false
if (dataDestinationConfigs != other.dataDestinationConfigs) return false
if (dataExtraDimensions != other.dataExtraDimensions) return false
if (description != other.description) return false
if (diagnosticsMode != other.diagnosticsMode) return false
if (expiryTime != other.expiryTime) return false
if (name != other.name) return false
if (postTriggerCollectionDuration != other.postTriggerCollectionDuration) return false
if (priority != other.priority) return false
if (signalCatalogArn != other.signalCatalogArn) return false
if (signalsToCollect != other.signalsToCollect) return false
if (spoolingMode != other.spoolingMode) return false
if (startTime != other.startTime) return false
if (tags != other.tags) return false
if (targetArn != other.targetArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.CreateCampaignRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The data collection scheme associated with the campaign. You can specify a scheme that collects data based on time or an event.
*/
public var collectionScheme: aws.sdk.kotlin.services.iotfleetwise.model.CollectionScheme? = null
/**
* (Optional) Whether to compress signals before transmitting data to Amazon Web Services IoT FleetWise. If you don't want to compress the signals, use `OFF`. If it's not specified, `SNAPPY` is used.
*
* Default: `SNAPPY`
*/
public var compression: aws.sdk.kotlin.services.iotfleetwise.model.Compression? = null
/**
* The destination where the campaign sends data. You can choose to send data to be stored in Amazon S3 or Amazon Timestream.
*
* Amazon S3 optimizes the cost of data storage and provides additional mechanisms to use vehicle data, such as data lakes, centralized data storage, data processing pipelines, and analytics. Amazon Web Services IoT FleetWise supports at-least-once file delivery to S3. Your vehicle data is stored on multiple Amazon Web Services IoT FleetWise servers for redundancy and high availability.
*
* You can use Amazon Timestream to access and analyze time series data, and Timestream to query vehicle data so that you can identify trends and patterns.
*/
public var dataDestinationConfigs: List? = null
/**
* (Optional) A list of vehicle attributes to associate with a campaign.
*
* Enrich the data with specified vehicle attributes. For example, add `make` and `model` to the campaign, and Amazon Web Services IoT FleetWise will associate the data with those attributes as dimensions in Amazon Timestream. You can then query the data against `make` and `model`.
*
* Default: An empty array
*/
public var dataExtraDimensions: List? = null
/**
* An optional description of the campaign to help identify its purpose.
*/
public var description: kotlin.String? = null
/**
* (Optional) Option for a vehicle to send diagnostic trouble codes to Amazon Web Services IoT FleetWise. If you want to send diagnostic trouble codes, use `SEND_ACTIVE_DTCS`. If it's not specified, `OFF` is used.
*
* Default: `OFF`
*/
public var diagnosticsMode: aws.sdk.kotlin.services.iotfleetwise.model.DiagnosticsMode? = null
/**
* (Optional) The time the campaign expires, in seconds since epoch (January 1, 1970 at midnight UTC time). Vehicle data isn't collected after the campaign expires.
*
* Default: 253402214400 (December 31, 9999, 00:00:00 UTC)
*/
public var expiryTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the campaign to create.
*/
public var name: kotlin.String? = null
/**
* (Optional) How long (in milliseconds) to collect raw data after a triggering event initiates the collection. If it's not specified, `0` is used.
*
* Default: `0`
*/
public var postTriggerCollectionDuration: kotlin.Long? = null
/**
* (Optional) A number indicating the priority of one campaign over another campaign for a certain vehicle or fleet. A campaign with the lowest value is deployed to vehicles before any other campaigns. If it's not specified, `0` is used.
*
* Default: `0`
*/
@Deprecated("priority is no longer used or needed as input")
public var priority: kotlin.Int? = null
/**
* The Amazon Resource Name (ARN) of the signal catalog to associate with the campaign.
*/
public var signalCatalogArn: kotlin.String? = null
/**
* (Optional) A list of information about signals to collect.
*/
public var signalsToCollect: List? = null
/**
* (Optional) Whether to store collected data after a vehicle lost a connection with the cloud. After a connection is re-established, the data is automatically forwarded to Amazon Web Services IoT FleetWise. If you want to store collected data when a vehicle loses connection with the cloud, use `TO_DISK`. If it's not specified, `OFF` is used.
*
* Default: `OFF`
*/
public var spoolingMode: aws.sdk.kotlin.services.iotfleetwise.model.SpoolingMode? = null
/**
* (Optional) The time, in milliseconds, to deliver a campaign after it was approved. If it's not specified, `0` is used.
*
* Default: `0`
*/
public var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Metadata that can be used to manage the campaign.
*/
public var tags: List? = null
/**
* The ARN of the vehicle or fleet to deploy a campaign to.
*/
public var targetArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.CreateCampaignRequest) : this() {
this.collectionScheme = x.collectionScheme
this.compression = x.compression
this.dataDestinationConfigs = x.dataDestinationConfigs
this.dataExtraDimensions = x.dataExtraDimensions
this.description = x.description
this.diagnosticsMode = x.diagnosticsMode
this.expiryTime = x.expiryTime
this.name = x.name
this.postTriggerCollectionDuration = x.postTriggerCollectionDuration
this.priority = x.priority
this.signalCatalogArn = x.signalCatalogArn
this.signalsToCollect = x.signalsToCollect
this.spoolingMode = x.spoolingMode
this.startTime = x.startTime
this.tags = x.tags
this.targetArn = x.targetArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.CreateCampaignRequest = CreateCampaignRequest(this)
internal fun correctErrors(): Builder {
if (name == null) name = ""
if (signalCatalogArn == null) signalCatalogArn = ""
if (targetArn == null) targetArn = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy