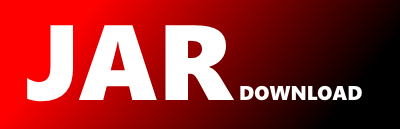
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.CreateVehicleRequestItem.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information about the vehicle to create.
*/
public class CreateVehicleRequestItem private constructor(builder: Builder) {
/**
* An option to create a new Amazon Web Services IoT thing when creating a vehicle, or to validate an existing thing as a vehicle.
*/
public val associationBehavior: aws.sdk.kotlin.services.iotfleetwise.model.VehicleAssociationBehavior? = builder.associationBehavior
/**
* Static information about a vehicle in a key-value pair. For example: `"engine Type"` : `"v6"`
*/
public val attributes: Map? = builder.attributes
/**
* The Amazon Resource Name (ARN) of a decoder manifest associated with the vehicle to create.
*/
public val decoderManifestArn: kotlin.String = requireNotNull(builder.decoderManifestArn) { "A non-null value must be provided for decoderManifestArn" }
/**
* The ARN of the vehicle model (model manifest) to create the vehicle from.
*/
public val modelManifestArn: kotlin.String = requireNotNull(builder.modelManifestArn) { "A non-null value must be provided for modelManifestArn" }
/**
* Metadata which can be used to manage the vehicle.
*/
public val tags: List? = builder.tags
/**
* The unique ID of the vehicle to create.
*/
public val vehicleName: kotlin.String = requireNotNull(builder.vehicleName) { "A non-null value must be provided for vehicleName" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.CreateVehicleRequestItem = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateVehicleRequestItem(")
append("associationBehavior=$associationBehavior,")
append("attributes=$attributes,")
append("decoderManifestArn=$decoderManifestArn,")
append("modelManifestArn=$modelManifestArn,")
append("tags=$tags,")
append("vehicleName=$vehicleName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = associationBehavior?.hashCode() ?: 0
result = 31 * result + (attributes?.hashCode() ?: 0)
result = 31 * result + (decoderManifestArn.hashCode())
result = 31 * result + (modelManifestArn.hashCode())
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (vehicleName.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateVehicleRequestItem
if (associationBehavior != other.associationBehavior) return false
if (attributes != other.attributes) return false
if (decoderManifestArn != other.decoderManifestArn) return false
if (modelManifestArn != other.modelManifestArn) return false
if (tags != other.tags) return false
if (vehicleName != other.vehicleName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.CreateVehicleRequestItem = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* An option to create a new Amazon Web Services IoT thing when creating a vehicle, or to validate an existing thing as a vehicle.
*/
public var associationBehavior: aws.sdk.kotlin.services.iotfleetwise.model.VehicleAssociationBehavior? = null
/**
* Static information about a vehicle in a key-value pair. For example: `"engine Type"` : `"v6"`
*/
public var attributes: Map? = null
/**
* The Amazon Resource Name (ARN) of a decoder manifest associated with the vehicle to create.
*/
public var decoderManifestArn: kotlin.String? = null
/**
* The ARN of the vehicle model (model manifest) to create the vehicle from.
*/
public var modelManifestArn: kotlin.String? = null
/**
* Metadata which can be used to manage the vehicle.
*/
public var tags: List? = null
/**
* The unique ID of the vehicle to create.
*/
public var vehicleName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.CreateVehicleRequestItem) : this() {
this.associationBehavior = x.associationBehavior
this.attributes = x.attributes
this.decoderManifestArn = x.decoderManifestArn
this.modelManifestArn = x.modelManifestArn
this.tags = x.tags
this.vehicleName = x.vehicleName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.CreateVehicleRequestItem = CreateVehicleRequestItem(this)
internal fun correctErrors(): Builder {
if (decoderManifestArn == null) decoderManifestArn = ""
if (modelManifestArn == null) modelManifestArn = ""
if (vehicleName == null) vehicleName = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy