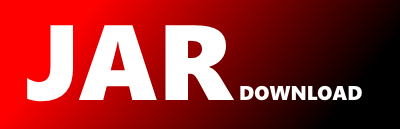
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Represents a member of the complex data structure. The data type of the property can be either primitive or another `struct`.
*/
public class CustomProperty private constructor(builder: Builder) {
/**
* A comment in addition to the description.
*/
public val comment: kotlin.String? = builder.comment
/**
* Indicates whether the property is binary data.
*/
public val dataEncoding: aws.sdk.kotlin.services.iotfleetwise.model.NodeDataEncoding? = builder.dataEncoding
/**
* The data type for the custom property.
*/
public val dataType: aws.sdk.kotlin.services.iotfleetwise.model.NodeDataType = requireNotNull(builder.dataType) { "A non-null value must be provided for dataType" }
/**
* The deprecation message for the node or the branch that was moved or deleted.
*/
public val deprecationMessage: kotlin.String? = builder.deprecationMessage
/**
* A brief description of the custom property.
*/
public val description: kotlin.String? = builder.description
/**
* The fully qualified name of the custom property. For example, the fully qualified name of a custom property might be `ComplexDataTypes.VehicleDataTypes.SVMCamera.FPS`.
*/
public val fullyQualifiedName: kotlin.String = requireNotNull(builder.fullyQualifiedName) { "A non-null value must be provided for fullyQualifiedName" }
/**
* The fully qualified name of the struct node for the custom property if the data type of the custom property is `Struct` or `StructArray`.
*/
public val structFullyQualifiedName: kotlin.String? = builder.structFullyQualifiedName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CustomProperty(")
append("comment=$comment,")
append("dataEncoding=$dataEncoding,")
append("dataType=$dataType,")
append("deprecationMessage=$deprecationMessage,")
append("description=$description,")
append("fullyQualifiedName=$fullyQualifiedName,")
append("structFullyQualifiedName=$structFullyQualifiedName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = comment?.hashCode() ?: 0
result = 31 * result + (dataEncoding?.hashCode() ?: 0)
result = 31 * result + (dataType.hashCode())
result = 31 * result + (deprecationMessage?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (fullyQualifiedName.hashCode())
result = 31 * result + (structFullyQualifiedName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CustomProperty
if (comment != other.comment) return false
if (dataEncoding != other.dataEncoding) return false
if (dataType != other.dataType) return false
if (deprecationMessage != other.deprecationMessage) return false
if (description != other.description) return false
if (fullyQualifiedName != other.fullyQualifiedName) return false
if (structFullyQualifiedName != other.structFullyQualifiedName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A comment in addition to the description.
*/
public var comment: kotlin.String? = null
/**
* Indicates whether the property is binary data.
*/
public var dataEncoding: aws.sdk.kotlin.services.iotfleetwise.model.NodeDataEncoding? = null
/**
* The data type for the custom property.
*/
public var dataType: aws.sdk.kotlin.services.iotfleetwise.model.NodeDataType? = null
/**
* The deprecation message for the node or the branch that was moved or deleted.
*/
public var deprecationMessage: kotlin.String? = null
/**
* A brief description of the custom property.
*/
public var description: kotlin.String? = null
/**
* The fully qualified name of the custom property. For example, the fully qualified name of a custom property might be `ComplexDataTypes.VehicleDataTypes.SVMCamera.FPS`.
*/
public var fullyQualifiedName: kotlin.String? = null
/**
* The fully qualified name of the struct node for the custom property if the data type of the custom property is `Struct` or `StructArray`.
*/
public var structFullyQualifiedName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty) : this() {
this.comment = x.comment
this.dataEncoding = x.dataEncoding
this.dataType = x.dataType
this.deprecationMessage = x.deprecationMessage
this.description = x.description
this.fullyQualifiedName = x.fullyQualifiedName
this.structFullyQualifiedName = x.structFullyQualifiedName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty = CustomProperty(this)
internal fun correctErrors(): Builder {
if (dataType == null) dataType = NodeDataType.SdkUnknown("no value provided")
if (fullyQualifiedName == null) fullyQualifiedName = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy