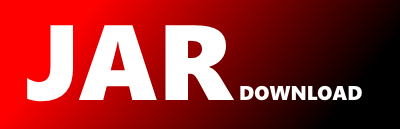
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.GetVehicleStatusRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
public class GetVehicleStatusRequest private constructor(builder: Builder) {
/**
* The maximum number of items to return, between 1 and 100, inclusive.
*/
public val maxResults: kotlin.Int? = builder.maxResults
/**
* A pagination token for the next set of results.
*
* If the results of a search are large, only a portion of the results are returned, and a `nextToken` pagination token is returned in the response. To retrieve the next set of results, reissue the search request and include the returned token. When all results have been returned, the response does not contain a pagination token value.
*/
public val nextToken: kotlin.String? = builder.nextToken
/**
* The ID of the vehicle to retrieve information about.
*/
public val vehicleName: kotlin.String = requireNotNull(builder.vehicleName) { "A non-null value must be provided for vehicleName" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.GetVehicleStatusRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetVehicleStatusRequest(")
append("maxResults=$maxResults,")
append("nextToken=$nextToken,")
append("vehicleName=$vehicleName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = maxResults ?: 0
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (vehicleName.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetVehicleStatusRequest
if (maxResults != other.maxResults) return false
if (nextToken != other.nextToken) return false
if (vehicleName != other.vehicleName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.GetVehicleStatusRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The maximum number of items to return, between 1 and 100, inclusive.
*/
public var maxResults: kotlin.Int? = null
/**
* A pagination token for the next set of results.
*
* If the results of a search are large, only a portion of the results are returned, and a `nextToken` pagination token is returned in the response. To retrieve the next set of results, reissue the search request and include the returned token. When all results have been returned, the response does not contain a pagination token value.
*/
public var nextToken: kotlin.String? = null
/**
* The ID of the vehicle to retrieve information about.
*/
public var vehicleName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.GetVehicleStatusRequest) : this() {
this.maxResults = x.maxResults
this.nextToken = x.nextToken
this.vehicleName = x.vehicleName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.GetVehicleStatusRequest = GetVehicleStatusRequest(this)
internal fun correctErrors(): Builder {
if (vehicleName == null) vehicleName = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy