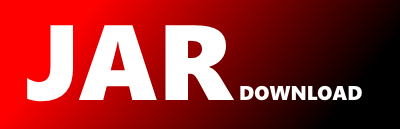
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.Node.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
/**
* A general abstraction of a signal. A node can be specified as an actuator, attribute, branch, or sensor.
*/
public sealed class Node {
/**
* Information about a node specified as an actuator.
*
* An actuator is a digital representation of a vehicle device.
*/
public data class Actuator(val value: aws.sdk.kotlin.services.iotfleetwise.model.Actuator) : aws.sdk.kotlin.services.iotfleetwise.model.Node() {
}
/**
* Information about a node specified as an attribute.
*
* An attribute represents static information about a vehicle.
*/
public data class Attribute(val value: aws.sdk.kotlin.services.iotfleetwise.model.Attribute) : aws.sdk.kotlin.services.iotfleetwise.model.Node() {
}
/**
* Information about a node specified as a branch.
*
* A group of signals that are defined in a hierarchical structure.
*/
public data class Branch(val value: aws.sdk.kotlin.services.iotfleetwise.model.Branch) : aws.sdk.kotlin.services.iotfleetwise.model.Node() {
}
/**
* Represents a member of the complex data structure. The `datatype` of the property can be either primitive or another `struct`.
*/
public data class Property(val value: aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty) : aws.sdk.kotlin.services.iotfleetwise.model.Node() {
}
/**
* An input component that reports the environmental condition of a vehicle.
*
* You can collect data about fluid levels, temperatures, vibrations, or battery voltage from sensors.
*/
public data class Sensor(val value: aws.sdk.kotlin.services.iotfleetwise.model.Sensor) : aws.sdk.kotlin.services.iotfleetwise.model.Node() {
}
/**
* Represents a complex or higher-order data structure.
*/
public data class Struct(val value: aws.sdk.kotlin.services.iotfleetwise.model.CustomStruct) : aws.sdk.kotlin.services.iotfleetwise.model.Node() {
}
public object SdkUnknown : aws.sdk.kotlin.services.iotfleetwise.model.Node() {
}
/**
* Casts this [Node] as a [Actuator] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.Actuator] value. Throws an exception if the [Node] is not a
* [Actuator].
*/
public fun asActuator(): aws.sdk.kotlin.services.iotfleetwise.model.Actuator = (this as Node.Actuator).value
/**
* Casts this [Node] as a [Actuator] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.Actuator] value. Returns null if the [Node] is not a [Actuator].
*/
public fun asActuatorOrNull(): aws.sdk.kotlin.services.iotfleetwise.model.Actuator? = (this as? Node.Actuator)?.value
/**
* Casts this [Node] as a [Attribute] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.Attribute] value. Throws an exception if the [Node] is not a
* [Attribute].
*/
public fun asAttribute(): aws.sdk.kotlin.services.iotfleetwise.model.Attribute = (this as Node.Attribute).value
/**
* Casts this [Node] as a [Attribute] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.Attribute] value. Returns null if the [Node] is not a [Attribute].
*/
public fun asAttributeOrNull(): aws.sdk.kotlin.services.iotfleetwise.model.Attribute? = (this as? Node.Attribute)?.value
/**
* Casts this [Node] as a [Branch] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.Branch] value. Throws an exception if the [Node] is not a
* [Branch].
*/
public fun asBranch(): aws.sdk.kotlin.services.iotfleetwise.model.Branch = (this as Node.Branch).value
/**
* Casts this [Node] as a [Branch] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.Branch] value. Returns null if the [Node] is not a [Branch].
*/
public fun asBranchOrNull(): aws.sdk.kotlin.services.iotfleetwise.model.Branch? = (this as? Node.Branch)?.value
/**
* Casts this [Node] as a [Property] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty] value. Throws an exception if the [Node] is not a
* [Property].
*/
public fun asProperty(): aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty = (this as Node.Property).value
/**
* Casts this [Node] as a [Property] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty] value. Returns null if the [Node] is not a [Property].
*/
public fun asPropertyOrNull(): aws.sdk.kotlin.services.iotfleetwise.model.CustomProperty? = (this as? Node.Property)?.value
/**
* Casts this [Node] as a [Sensor] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.Sensor] value. Throws an exception if the [Node] is not a
* [Sensor].
*/
public fun asSensor(): aws.sdk.kotlin.services.iotfleetwise.model.Sensor = (this as Node.Sensor).value
/**
* Casts this [Node] as a [Sensor] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.Sensor] value. Returns null if the [Node] is not a [Sensor].
*/
public fun asSensorOrNull(): aws.sdk.kotlin.services.iotfleetwise.model.Sensor? = (this as? Node.Sensor)?.value
/**
* Casts this [Node] as a [Struct] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.CustomStruct] value. Throws an exception if the [Node] is not a
* [Struct].
*/
public fun asStruct(): aws.sdk.kotlin.services.iotfleetwise.model.CustomStruct = (this as Node.Struct).value
/**
* Casts this [Node] as a [Struct] and retrieves its [aws.sdk.kotlin.services.iotfleetwise.model.CustomStruct] value. Returns null if the [Node] is not a [Struct].
*/
public fun asStructOrNull(): aws.sdk.kotlin.services.iotfleetwise.model.CustomStruct? = (this as? Node.Struct)?.value
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy