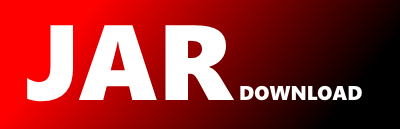
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information about signal messages using the on-board diagnostics (OBD) II protocol in a vehicle.
*/
public class ObdSignal private constructor(builder: Builder) {
/**
* The number of bits to mask in a message.
*/
public val bitMaskLength: kotlin.Int? = builder.bitMaskLength
/**
* The number of positions to shift bits in the message.
*/
public val bitRightShift: kotlin.Int = builder.bitRightShift
/**
* The length of a message.
*/
public val byteLength: kotlin.Int = requireNotNull(builder.byteLength) { "A non-null value must be provided for byteLength" }
/**
* The offset used to calculate the signal value. Combined with scaling, the calculation is `value = raw_value * scaling + offset`.
*/
public val offset: kotlin.Double = requireNotNull(builder.offset) { "A non-null value must be provided for offset" }
/**
* The diagnostic code used to request data from a vehicle for this signal.
*/
public val pid: kotlin.Int = builder.pid
/**
* The length of the requested data.
*/
public val pidResponseLength: kotlin.Int = requireNotNull(builder.pidResponseLength) { "A non-null value must be provided for pidResponseLength" }
/**
* A multiplier used to decode the message.
*/
public val scaling: kotlin.Double = requireNotNull(builder.scaling) { "A non-null value must be provided for scaling" }
/**
* The mode of operation (diagnostic service) in a message.
*/
public val serviceMode: kotlin.Int = builder.serviceMode
/**
* Indicates the beginning of the message.
*/
public val startByte: kotlin.Int = builder.startByte
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ObdSignal(")
append("bitMaskLength=$bitMaskLength,")
append("bitRightShift=$bitRightShift,")
append("byteLength=$byteLength,")
append("offset=$offset,")
append("pid=$pid,")
append("pidResponseLength=$pidResponseLength,")
append("scaling=$scaling,")
append("serviceMode=$serviceMode,")
append("startByte=$startByte")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = bitMaskLength ?: 0
result = 31 * result + (bitRightShift)
result = 31 * result + (byteLength)
result = 31 * result + (offset.hashCode())
result = 31 * result + (pid)
result = 31 * result + (pidResponseLength)
result = 31 * result + (scaling.hashCode())
result = 31 * result + (serviceMode)
result = 31 * result + (startByte)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ObdSignal
if (bitMaskLength != other.bitMaskLength) return false
if (bitRightShift != other.bitRightShift) return false
if (byteLength != other.byteLength) return false
if (!(offset?.equals(other.offset) ?: (other.offset == null))) return false
if (pid != other.pid) return false
if (pidResponseLength != other.pidResponseLength) return false
if (!(scaling?.equals(other.scaling) ?: (other.scaling == null))) return false
if (serviceMode != other.serviceMode) return false
if (startByte != other.startByte) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The number of bits to mask in a message.
*/
public var bitMaskLength: kotlin.Int? = null
/**
* The number of positions to shift bits in the message.
*/
public var bitRightShift: kotlin.Int = 0
/**
* The length of a message.
*/
public var byteLength: kotlin.Int? = null
/**
* The offset used to calculate the signal value. Combined with scaling, the calculation is `value = raw_value * scaling + offset`.
*/
public var offset: kotlin.Double? = null
/**
* The diagnostic code used to request data from a vehicle for this signal.
*/
public var pid: kotlin.Int = 0
/**
* The length of the requested data.
*/
public var pidResponseLength: kotlin.Int? = null
/**
* A multiplier used to decode the message.
*/
public var scaling: kotlin.Double? = null
/**
* The mode of operation (diagnostic service) in a message.
*/
public var serviceMode: kotlin.Int = 0
/**
* Indicates the beginning of the message.
*/
public var startByte: kotlin.Int = 0
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal) : this() {
this.bitMaskLength = x.bitMaskLength
this.bitRightShift = x.bitRightShift
this.byteLength = x.byteLength
this.offset = x.offset
this.pid = x.pid
this.pidResponseLength = x.pidResponseLength
this.scaling = x.scaling
this.serviceMode = x.serviceMode
this.startByte = x.startByte
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal = ObdSignal(this)
internal fun correctErrors(): Builder {
if (byteLength == null) byteLength = 0
if (offset == null) offset = 0.0
if (pidResponseLength == null) pidResponseLength = 0
if (scaling == null) scaling = 0.0
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy