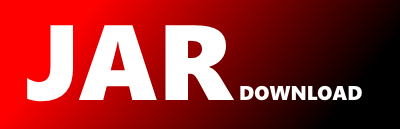
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.S3Config.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The Amazon S3 bucket where the Amazon Web Services IoT FleetWise campaign sends data. Amazon S3 is an object storage service that stores data as objects within buckets. For more information, see [Creating, configuring, and working with Amazon S3 buckets](https://docs.aws.amazon.com/AmazonS3/latest/userguide/creating-buckets-s3.html) in the *Amazon Simple Storage Service User Guide*.
*/
public class S3Config private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the Amazon S3 bucket.
*/
public val bucketArn: kotlin.String = requireNotNull(builder.bucketArn) { "A non-null value must be provided for bucketArn" }
/**
* Specify the format that files are saved in the Amazon S3 bucket. You can save files in an Apache Parquet or JSON format.
* + Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and can reduce costs. This option is selected by default.
* + JSON - Store data in a standard text-based JSON file format.
*/
public val dataFormat: aws.sdk.kotlin.services.iotfleetwise.model.DataFormat? = builder.dataFormat
/**
* (Optional) Enter an S3 bucket prefix. The prefix is the string of characters after the bucket name and before the object name. You can use the prefix to organize data stored in Amazon S3 buckets. For more information, see [Organizing objects using prefixes](https://docs.aws.amazon.com/AmazonS3/latest/userguide/using-prefixes.html) in the *Amazon Simple Storage Service User Guide*.
*
* By default, Amazon Web Services IoT FleetWise sets the prefix `processed-data/year=YY/month=MM/date=DD/hour=HH/` (in UTC) to data it delivers to Amazon S3. You can enter a prefix to append it to this default prefix. For example, if you enter the prefix `vehicles`, the prefix will be `vehicles/processed-data/year=YY/month=MM/date=DD/hour=HH/`.
*/
public val prefix: kotlin.String? = builder.prefix
/**
* By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size, which can optimize the cost of data storage.
*/
public val storageCompressionFormat: aws.sdk.kotlin.services.iotfleetwise.model.StorageCompressionFormat? = builder.storageCompressionFormat
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.S3Config = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("S3Config(")
append("bucketArn=$bucketArn,")
append("dataFormat=$dataFormat,")
append("prefix=$prefix,")
append("storageCompressionFormat=$storageCompressionFormat")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = bucketArn.hashCode()
result = 31 * result + (dataFormat?.hashCode() ?: 0)
result = 31 * result + (prefix?.hashCode() ?: 0)
result = 31 * result + (storageCompressionFormat?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as S3Config
if (bucketArn != other.bucketArn) return false
if (dataFormat != other.dataFormat) return false
if (prefix != other.prefix) return false
if (storageCompressionFormat != other.storageCompressionFormat) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.S3Config = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the Amazon S3 bucket.
*/
public var bucketArn: kotlin.String? = null
/**
* Specify the format that files are saved in the Amazon S3 bucket. You can save files in an Apache Parquet or JSON format.
* + Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and can reduce costs. This option is selected by default.
* + JSON - Store data in a standard text-based JSON file format.
*/
public var dataFormat: aws.sdk.kotlin.services.iotfleetwise.model.DataFormat? = null
/**
* (Optional) Enter an S3 bucket prefix. The prefix is the string of characters after the bucket name and before the object name. You can use the prefix to organize data stored in Amazon S3 buckets. For more information, see [Organizing objects using prefixes](https://docs.aws.amazon.com/AmazonS3/latest/userguide/using-prefixes.html) in the *Amazon Simple Storage Service User Guide*.
*
* By default, Amazon Web Services IoT FleetWise sets the prefix `processed-data/year=YY/month=MM/date=DD/hour=HH/` (in UTC) to data it delivers to Amazon S3. You can enter a prefix to append it to this default prefix. For example, if you enter the prefix `vehicles`, the prefix will be `vehicles/processed-data/year=YY/month=MM/date=DD/hour=HH/`.
*/
public var prefix: kotlin.String? = null
/**
* By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size, which can optimize the cost of data storage.
*/
public var storageCompressionFormat: aws.sdk.kotlin.services.iotfleetwise.model.StorageCompressionFormat? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.S3Config) : this() {
this.bucketArn = x.bucketArn
this.dataFormat = x.dataFormat
this.prefix = x.prefix
this.storageCompressionFormat = x.storageCompressionFormat
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.S3Config = S3Config(this)
internal fun correctErrors(): Builder {
if (bucketArn == null) bucketArn = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy