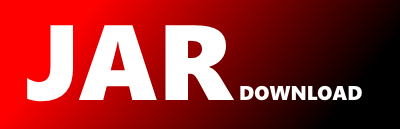
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.SignalDecoder.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information about a signal decoder.
*/
public class SignalDecoder private constructor(builder: Builder) {
/**
* Information about signal decoder using the Controller Area Network (CAN) protocol.
*/
public val canSignal: aws.sdk.kotlin.services.iotfleetwise.model.CanSignal? = builder.canSignal
/**
* The fully qualified name of a signal decoder as defined in a vehicle model.
*/
public val fullyQualifiedName: kotlin.String = requireNotNull(builder.fullyQualifiedName) { "A non-null value must be provided for fullyQualifiedName" }
/**
* The ID of a network interface that specifies what network protocol a vehicle follows.
*/
public val interfaceId: kotlin.String = requireNotNull(builder.interfaceId) { "A non-null value must be provided for interfaceId" }
/**
* The decoding information for a specific message which supports higher order data types.
*/
public val messageSignal: aws.sdk.kotlin.services.iotfleetwise.model.MessageSignal? = builder.messageSignal
/**
* Information about signal decoder using the On-board diagnostic (OBD) II protocol.
*/
public val obdSignal: aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal? = builder.obdSignal
/**
* The network protocol for the vehicle. For example, `CAN_SIGNAL` specifies a protocol that defines how data is communicated between electronic control units (ECUs). `OBD_SIGNAL` specifies a protocol that defines how self-diagnostic data is communicated between ECUs.
*/
public val type: aws.sdk.kotlin.services.iotfleetwise.model.SignalDecoderType = requireNotNull(builder.type) { "A non-null value must be provided for type" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.SignalDecoder = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SignalDecoder(")
append("canSignal=$canSignal,")
append("fullyQualifiedName=$fullyQualifiedName,")
append("interfaceId=$interfaceId,")
append("messageSignal=$messageSignal,")
append("obdSignal=$obdSignal,")
append("type=$type")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = canSignal?.hashCode() ?: 0
result = 31 * result + (fullyQualifiedName.hashCode())
result = 31 * result + (interfaceId.hashCode())
result = 31 * result + (messageSignal?.hashCode() ?: 0)
result = 31 * result + (obdSignal?.hashCode() ?: 0)
result = 31 * result + (type.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SignalDecoder
if (canSignal != other.canSignal) return false
if (fullyQualifiedName != other.fullyQualifiedName) return false
if (interfaceId != other.interfaceId) return false
if (messageSignal != other.messageSignal) return false
if (obdSignal != other.obdSignal) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.SignalDecoder = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Information about signal decoder using the Controller Area Network (CAN) protocol.
*/
public var canSignal: aws.sdk.kotlin.services.iotfleetwise.model.CanSignal? = null
/**
* The fully qualified name of a signal decoder as defined in a vehicle model.
*/
public var fullyQualifiedName: kotlin.String? = null
/**
* The ID of a network interface that specifies what network protocol a vehicle follows.
*/
public var interfaceId: kotlin.String? = null
/**
* The decoding information for a specific message which supports higher order data types.
*/
public var messageSignal: aws.sdk.kotlin.services.iotfleetwise.model.MessageSignal? = null
/**
* Information about signal decoder using the On-board diagnostic (OBD) II protocol.
*/
public var obdSignal: aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal? = null
/**
* The network protocol for the vehicle. For example, `CAN_SIGNAL` specifies a protocol that defines how data is communicated between electronic control units (ECUs). `OBD_SIGNAL` specifies a protocol that defines how self-diagnostic data is communicated between ECUs.
*/
public var type: aws.sdk.kotlin.services.iotfleetwise.model.SignalDecoderType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.SignalDecoder) : this() {
this.canSignal = x.canSignal
this.fullyQualifiedName = x.fullyQualifiedName
this.interfaceId = x.interfaceId
this.messageSignal = x.messageSignal
this.obdSignal = x.obdSignal
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.SignalDecoder = SignalDecoder(this)
/**
* construct an [aws.sdk.kotlin.services.iotfleetwise.model.CanSignal] inside the given [block]
*/
public fun canSignal(block: aws.sdk.kotlin.services.iotfleetwise.model.CanSignal.Builder.() -> kotlin.Unit) {
this.canSignal = aws.sdk.kotlin.services.iotfleetwise.model.CanSignal.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotfleetwise.model.MessageSignal] inside the given [block]
*/
public fun messageSignal(block: aws.sdk.kotlin.services.iotfleetwise.model.MessageSignal.Builder.() -> kotlin.Unit) {
this.messageSignal = aws.sdk.kotlin.services.iotfleetwise.model.MessageSignal.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal] inside the given [block]
*/
public fun obdSignal(block: aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal.Builder.() -> kotlin.Unit) {
this.obdSignal = aws.sdk.kotlin.services.iotfleetwise.model.ObdSignal.invoke(block)
}
internal fun correctErrors(): Builder {
if (fullyQualifiedName == null) fullyQualifiedName = ""
if (interfaceId == null) interfaceId = ""
if (type == null) type = SignalDecoderType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy