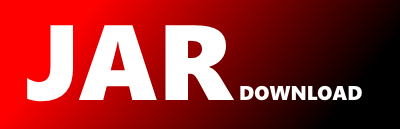
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.TimestreamConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The Amazon Timestream table where the Amazon Web Services IoT FleetWise campaign sends data. Timestream stores and organizes data to optimize query processing time and to reduce storage costs. For more information, see [Data modeling](https://docs.aws.amazon.com/timestream/latest/developerguide/data-modeling.html) in the *Amazon Timestream Developer Guide*.
*/
public class TimestreamConfig private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the task execution role that grants Amazon Web Services IoT FleetWise permission to deliver data to the Amazon Timestream table.
*/
public val executionRoleArn: kotlin.String = requireNotNull(builder.executionRoleArn) { "A non-null value must be provided for executionRoleArn" }
/**
* The Amazon Resource Name (ARN) of the Amazon Timestream table.
*/
public val timestreamTableArn: kotlin.String = requireNotNull(builder.timestreamTableArn) { "A non-null value must be provided for timestreamTableArn" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.TimestreamConfig = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TimestreamConfig(")
append("executionRoleArn=$executionRoleArn,")
append("timestreamTableArn=$timestreamTableArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = executionRoleArn.hashCode()
result = 31 * result + (timestreamTableArn.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TimestreamConfig
if (executionRoleArn != other.executionRoleArn) return false
if (timestreamTableArn != other.timestreamTableArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.TimestreamConfig = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the task execution role that grants Amazon Web Services IoT FleetWise permission to deliver data to the Amazon Timestream table.
*/
public var executionRoleArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the Amazon Timestream table.
*/
public var timestreamTableArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.TimestreamConfig) : this() {
this.executionRoleArn = x.executionRoleArn
this.timestreamTableArn = x.timestreamTableArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.TimestreamConfig = TimestreamConfig(this)
internal fun correctErrors(): Builder {
if (executionRoleArn == null) executionRoleArn = ""
if (timestreamTableArn == null) timestreamTableArn = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy