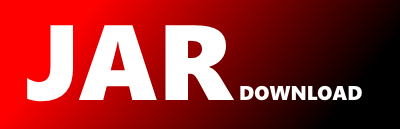
commonMain.aws.sdk.kotlin.services.iotfleetwise.model.UpdateDecoderManifestRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise-jvm Show documentation
Show all versions of iotfleetwise-jvm Show documentation
The AWS SDK for Kotlin client for IoTFleetWise
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotfleetwise.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateDecoderManifestRequest private constructor(builder: Builder) {
/**
* A brief description of the decoder manifest to update.
*/
public val description: kotlin.String? = builder.description
/**
* The name of the decoder manifest to update.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* A list of information about the network interfaces to add to the decoder manifest.
*/
public val networkInterfacesToAdd: List? = builder.networkInterfacesToAdd
/**
* A list of network interfaces to remove from the decoder manifest.
*/
public val networkInterfacesToRemove: List? = builder.networkInterfacesToRemove
/**
* A list of information about the network interfaces to update in the decoder manifest.
*/
public val networkInterfacesToUpdate: List? = builder.networkInterfacesToUpdate
/**
* A list of information about decoding additional signals to add to the decoder manifest.
*/
public val signalDecodersToAdd: List? = builder.signalDecodersToAdd
/**
* A list of signal decoders to remove from the decoder manifest.
*/
public val signalDecodersToRemove: List? = builder.signalDecodersToRemove
/**
* A list of updated information about decoding signals to update in the decoder manifest.
*/
public val signalDecodersToUpdate: List? = builder.signalDecodersToUpdate
/**
* The state of the decoder manifest. If the status is `ACTIVE`, the decoder manifest can't be edited. If the status is `DRAFT`, you can edit the decoder manifest.
*/
public val status: aws.sdk.kotlin.services.iotfleetwise.model.ManifestStatus? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotfleetwise.model.UpdateDecoderManifestRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateDecoderManifestRequest(")
append("description=$description,")
append("name=$name,")
append("networkInterfacesToAdd=$networkInterfacesToAdd,")
append("networkInterfacesToRemove=$networkInterfacesToRemove,")
append("networkInterfacesToUpdate=$networkInterfacesToUpdate,")
append("signalDecodersToAdd=$signalDecodersToAdd,")
append("signalDecodersToRemove=$signalDecodersToRemove,")
append("signalDecodersToUpdate=$signalDecodersToUpdate,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (name.hashCode())
result = 31 * result + (networkInterfacesToAdd?.hashCode() ?: 0)
result = 31 * result + (networkInterfacesToRemove?.hashCode() ?: 0)
result = 31 * result + (networkInterfacesToUpdate?.hashCode() ?: 0)
result = 31 * result + (signalDecodersToAdd?.hashCode() ?: 0)
result = 31 * result + (signalDecodersToRemove?.hashCode() ?: 0)
result = 31 * result + (signalDecodersToUpdate?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateDecoderManifestRequest
if (description != other.description) return false
if (name != other.name) return false
if (networkInterfacesToAdd != other.networkInterfacesToAdd) return false
if (networkInterfacesToRemove != other.networkInterfacesToRemove) return false
if (networkInterfacesToUpdate != other.networkInterfacesToUpdate) return false
if (signalDecodersToAdd != other.signalDecodersToAdd) return false
if (signalDecodersToRemove != other.signalDecodersToRemove) return false
if (signalDecodersToUpdate != other.signalDecodersToUpdate) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotfleetwise.model.UpdateDecoderManifestRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A brief description of the decoder manifest to update.
*/
public var description: kotlin.String? = null
/**
* The name of the decoder manifest to update.
*/
public var name: kotlin.String? = null
/**
* A list of information about the network interfaces to add to the decoder manifest.
*/
public var networkInterfacesToAdd: List? = null
/**
* A list of network interfaces to remove from the decoder manifest.
*/
public var networkInterfacesToRemove: List? = null
/**
* A list of information about the network interfaces to update in the decoder manifest.
*/
public var networkInterfacesToUpdate: List? = null
/**
* A list of information about decoding additional signals to add to the decoder manifest.
*/
public var signalDecodersToAdd: List? = null
/**
* A list of signal decoders to remove from the decoder manifest.
*/
public var signalDecodersToRemove: List? = null
/**
* A list of updated information about decoding signals to update in the decoder manifest.
*/
public var signalDecodersToUpdate: List? = null
/**
* The state of the decoder manifest. If the status is `ACTIVE`, the decoder manifest can't be edited. If the status is `DRAFT`, you can edit the decoder manifest.
*/
public var status: aws.sdk.kotlin.services.iotfleetwise.model.ManifestStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotfleetwise.model.UpdateDecoderManifestRequest) : this() {
this.description = x.description
this.name = x.name
this.networkInterfacesToAdd = x.networkInterfacesToAdd
this.networkInterfacesToRemove = x.networkInterfacesToRemove
this.networkInterfacesToUpdate = x.networkInterfacesToUpdate
this.signalDecodersToAdd = x.signalDecodersToAdd
this.signalDecodersToRemove = x.signalDecodersToRemove
this.signalDecodersToUpdate = x.signalDecodersToUpdate
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotfleetwise.model.UpdateDecoderManifestRequest = UpdateDecoderManifestRequest(this)
internal fun correctErrors(): Builder {
if (name == null) name = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy