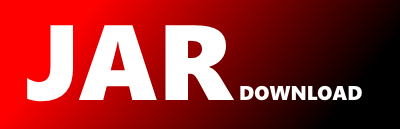
commonMain.aws.sdk.kotlin.services.iotsitewise.model.Alarms.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains the configuration information of an alarm created in an IoT SiteWise Monitor portal. You can use the alarm to monitor an asset property and get notified when the asset property value is outside a specified range. For more information, see [Monitoring with alarms](https://docs.aws.amazon.com/iot-sitewise/latest/appguide/monitor-alarms.html) in the *IoT SiteWise Application Guide*.
*/
public class Alarms private constructor(builder: Builder) {
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the IAM role that allows the alarm to perform actions and access Amazon Web Services resources and services, such as IoT Events.
*/
public val alarmRoleArn: kotlin.String = requireNotNull(builder.alarmRoleArn) { "A non-null value must be provided for alarmRoleArn" }
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the Lambda function that manages alarm notifications. For more information, see [Managing alarm notifications](https://docs.aws.amazon.com/iotevents/latest/developerguide/lambda-support.html) in the *IoT Events Developer Guide*.
*/
public val notificationLambdaArn: kotlin.String? = builder.notificationLambdaArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.Alarms = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Alarms(")
append("alarmRoleArn=$alarmRoleArn,")
append("notificationLambdaArn=$notificationLambdaArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = alarmRoleArn.hashCode()
result = 31 * result + (notificationLambdaArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Alarms
if (alarmRoleArn != other.alarmRoleArn) return false
if (notificationLambdaArn != other.notificationLambdaArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.Alarms = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the IAM role that allows the alarm to perform actions and access Amazon Web Services resources and services, such as IoT Events.
*/
public var alarmRoleArn: kotlin.String? = null
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the Lambda function that manages alarm notifications. For more information, see [Managing alarm notifications](https://docs.aws.amazon.com/iotevents/latest/developerguide/lambda-support.html) in the *IoT Events Developer Guide*.
*/
public var notificationLambdaArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.Alarms) : this() {
this.alarmRoleArn = x.alarmRoleArn
this.notificationLambdaArn = x.notificationLambdaArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.Alarms = Alarms(this)
internal fun correctErrors(): Builder {
if (alarmRoleArn == null) alarmRoleArn = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy