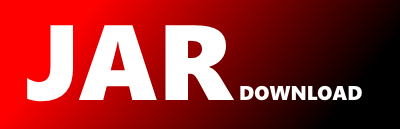
commonMain.aws.sdk.kotlin.services.iotsitewise.model.AssetHierarchy.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Describes an asset hierarchy that contains a hierarchy's name and ID.
*/
public class AssetHierarchy private constructor(builder: Builder) {
/**
* The external ID of the hierarchy, if it has one. When you update an asset hierarchy, you may assign an external ID if it doesn't already have one. You can't change the external ID of an asset hierarchy that already has one. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public val externalId: kotlin.String? = builder.externalId
/**
* The ID of the hierarchy. This ID is a `hierarchyId`.
*/
public val id: kotlin.String? = builder.id
/**
* The hierarchy name provided in the [CreateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_CreateAssetModel.html) or [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html) API operation.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.AssetHierarchy = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AssetHierarchy(")
append("externalId=$externalId,")
append("id=$id,")
append("name=$name")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = externalId?.hashCode() ?: 0
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AssetHierarchy
if (externalId != other.externalId) return false
if (id != other.id) return false
if (name != other.name) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.AssetHierarchy = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The external ID of the hierarchy, if it has one. When you update an asset hierarchy, you may assign an external ID if it doesn't already have one. You can't change the external ID of an asset hierarchy that already has one. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public var externalId: kotlin.String? = null
/**
* The ID of the hierarchy. This ID is a `hierarchyId`.
*/
public var id: kotlin.String? = null
/**
* The hierarchy name provided in the [CreateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_CreateAssetModel.html) or [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html) API operation.
*/
public var name: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.AssetHierarchy) : this() {
this.externalId = x.externalId
this.id = x.id
this.name = x.name
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.AssetHierarchy = AssetHierarchy(this)
internal fun correctErrors(): Builder {
if (name == null) name = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy