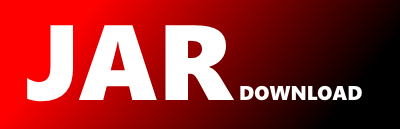
commonMain.aws.sdk.kotlin.services.iotsitewise.model.AssetModelCompositeModelDefinition.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains a composite model definition in an asset model. This composite model definition is applied to all assets created from the asset model.
*/
public class AssetModelCompositeModelDefinition private constructor(builder: Builder) {
/**
* The description of the composite model.
*/
public val description: kotlin.String? = builder.description
/**
* An external ID to assign to the composite model. The external ID must be unique among composite models within this asset model. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public val externalId: kotlin.String? = builder.externalId
/**
* The ID to assign to the composite model, if desired. IoT SiteWise automatically generates a unique ID for you, so this parameter is never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If you specify your own ID, it must be globally unique.
*/
public val id: kotlin.String? = builder.id
/**
* The name of the composite model.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* The asset property definitions for this composite model.
*/
public val properties: List? = builder.properties
/**
* The type of the composite model. For alarm composite models, this type is `AWS/ALARM`.
*/
public val type: kotlin.String = requireNotNull(builder.type) { "A non-null value must be provided for type" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.AssetModelCompositeModelDefinition = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AssetModelCompositeModelDefinition(")
append("description=$description,")
append("externalId=$externalId,")
append("id=$id,")
append("name=$name,")
append("properties=$properties,")
append("type=$type")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (externalId?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (properties?.hashCode() ?: 0)
result = 31 * result + (type.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AssetModelCompositeModelDefinition
if (description != other.description) return false
if (externalId != other.externalId) return false
if (id != other.id) return false
if (name != other.name) return false
if (properties != other.properties) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.AssetModelCompositeModelDefinition = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The description of the composite model.
*/
public var description: kotlin.String? = null
/**
* An external ID to assign to the composite model. The external ID must be unique among composite models within this asset model. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public var externalId: kotlin.String? = null
/**
* The ID to assign to the composite model, if desired. IoT SiteWise automatically generates a unique ID for you, so this parameter is never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If you specify your own ID, it must be globally unique.
*/
public var id: kotlin.String? = null
/**
* The name of the composite model.
*/
public var name: kotlin.String? = null
/**
* The asset property definitions for this composite model.
*/
public var properties: List? = null
/**
* The type of the composite model. For alarm composite models, this type is `AWS/ALARM`.
*/
public var type: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.AssetModelCompositeModelDefinition) : this() {
this.description = x.description
this.externalId = x.externalId
this.id = x.id
this.name = x.name
this.properties = x.properties
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.AssetModelCompositeModelDefinition = AssetModelCompositeModelDefinition(this)
internal fun correctErrors(): Builder {
if (name == null) name = ""
if (type == null) type = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy