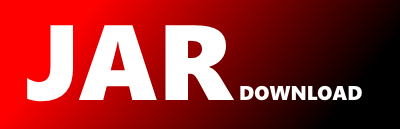
commonMain.aws.sdk.kotlin.services.iotsitewise.model.AssetModelHierarchyDefinition.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains an asset model hierarchy used in asset model creation. An asset model hierarchy determines the kind (or type) of asset that can belong to a hierarchy.
*/
public class AssetModelHierarchyDefinition private constructor(builder: Builder) {
/**
* The ID of an asset model for this hierarchy. This can be either the actual ID in UUID format, or else `externalId:` followed by the external ID, if it has one. For more information, see [Referencing objects with external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-id-references) in the *IoT SiteWise User Guide*.
*/
public val childAssetModelId: kotlin.String = requireNotNull(builder.childAssetModelId) { "A non-null value must be provided for childAssetModelId" }
/**
* An external ID to assign to the asset model hierarchy. The external ID must be unique among asset model hierarchies within this asset model. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public val externalId: kotlin.String? = builder.externalId
/**
* The ID to assign to the asset model hierarchy, if desired. IoT SiteWise automatically generates a unique ID for you, so this parameter is never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If you specify your own ID, it must be globally unique.
*/
public val id: kotlin.String? = builder.id
/**
* The name of the asset model hierarchy definition (as specified in the [CreateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_CreateAssetModel.html) or [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html) API operation).
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.AssetModelHierarchyDefinition = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AssetModelHierarchyDefinition(")
append("childAssetModelId=$childAssetModelId,")
append("externalId=$externalId,")
append("id=$id,")
append("name=$name")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = childAssetModelId.hashCode()
result = 31 * result + (externalId?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AssetModelHierarchyDefinition
if (childAssetModelId != other.childAssetModelId) return false
if (externalId != other.externalId) return false
if (id != other.id) return false
if (name != other.name) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.AssetModelHierarchyDefinition = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ID of an asset model for this hierarchy. This can be either the actual ID in UUID format, or else `externalId:` followed by the external ID, if it has one. For more information, see [Referencing objects with external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-id-references) in the *IoT SiteWise User Guide*.
*/
public var childAssetModelId: kotlin.String? = null
/**
* An external ID to assign to the asset model hierarchy. The external ID must be unique among asset model hierarchies within this asset model. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public var externalId: kotlin.String? = null
/**
* The ID to assign to the asset model hierarchy, if desired. IoT SiteWise automatically generates a unique ID for you, so this parameter is never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If you specify your own ID, it must be globally unique.
*/
public var id: kotlin.String? = null
/**
* The name of the asset model hierarchy definition (as specified in the [CreateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_CreateAssetModel.html) or [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html) API operation).
*/
public var name: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.AssetModelHierarchyDefinition) : this() {
this.childAssetModelId = x.childAssetModelId
this.externalId = x.externalId
this.id = x.id
this.name = x.name
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.AssetModelHierarchyDefinition = AssetModelHierarchyDefinition(this)
internal fun correctErrors(): Builder {
if (childAssetModelId == null) childAssetModelId = ""
if (name == null) name = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy