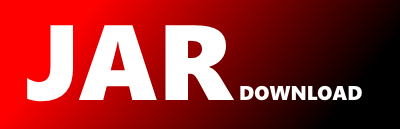
commonMain.aws.sdk.kotlin.services.iotsitewise.model.AssetModelProperty.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains information about an asset model property.
*/
public class AssetModelProperty private constructor(builder: Builder) {
/**
* The data type of the asset model property.
*/
public val dataType: aws.sdk.kotlin.services.iotsitewise.model.PropertyDataType = requireNotNull(builder.dataType) { "A non-null value must be provided for dataType" }
/**
* The data type of the structure for this property. This parameter exists on properties that have the `STRUCT` data type.
*/
public val dataTypeSpec: kotlin.String? = builder.dataTypeSpec
/**
* The external ID (if any) provided in the [CreateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_CreateAssetModel.html) or [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html) operation. You can assign an external ID by specifying this value as part of a call to [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html). However, you can't change the external ID if one is already assigned. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public val externalId: kotlin.String? = builder.externalId
/**
* The ID of the asset model property.
* + If you are callling [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html) to create a *new* property: You can specify its ID here, if desired. IoT SiteWise automatically generates a unique ID for you, so this parameter is never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If you specify your own ID, it must be globally unique.
* + If you are calling UpdateAssetModel to modify an *existing* property: This can be either the actual ID in UUID format, or else `externalId:` followed by the external ID, if it has one. For more information, see [Referencing objects with external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-id-references) in the *IoT SiteWise User Guide*.
*/
public val id: kotlin.String? = builder.id
/**
* The name of the asset model property.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* The structured path to the property from the root of the asset model.
*/
public val path: List? = builder.path
/**
* The property type (see `PropertyType`).
*/
public val type: aws.sdk.kotlin.services.iotsitewise.model.PropertyType? = builder.type
/**
* The unit of the asset model property, such as `Newtons` or `RPM`.
*/
public val unit: kotlin.String? = builder.unit
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.AssetModelProperty = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AssetModelProperty(")
append("dataType=$dataType,")
append("dataTypeSpec=$dataTypeSpec,")
append("externalId=$externalId,")
append("id=$id,")
append("name=$name,")
append("path=$path,")
append("type=$type,")
append("unit=$unit")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = dataType.hashCode()
result = 31 * result + (dataTypeSpec?.hashCode() ?: 0)
result = 31 * result + (externalId?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (path?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
result = 31 * result + (unit?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AssetModelProperty
if (dataType != other.dataType) return false
if (dataTypeSpec != other.dataTypeSpec) return false
if (externalId != other.externalId) return false
if (id != other.id) return false
if (name != other.name) return false
if (path != other.path) return false
if (type != other.type) return false
if (unit != other.unit) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.AssetModelProperty = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The data type of the asset model property.
*/
public var dataType: aws.sdk.kotlin.services.iotsitewise.model.PropertyDataType? = null
/**
* The data type of the structure for this property. This parameter exists on properties that have the `STRUCT` data type.
*/
public var dataTypeSpec: kotlin.String? = null
/**
* The external ID (if any) provided in the [CreateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_CreateAssetModel.html) or [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html) operation. You can assign an external ID by specifying this value as part of a call to [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html). However, you can't change the external ID if one is already assigned. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public var externalId: kotlin.String? = null
/**
* The ID of the asset model property.
* + If you are callling [UpdateAssetModel](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_UpdateAssetModel.html) to create a *new* property: You can specify its ID here, if desired. IoT SiteWise automatically generates a unique ID for you, so this parameter is never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If you specify your own ID, it must be globally unique.
* + If you are calling UpdateAssetModel to modify an *existing* property: This can be either the actual ID in UUID format, or else `externalId:` followed by the external ID, if it has one. For more information, see [Referencing objects with external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-id-references) in the *IoT SiteWise User Guide*.
*/
public var id: kotlin.String? = null
/**
* The name of the asset model property.
*/
public var name: kotlin.String? = null
/**
* The structured path to the property from the root of the asset model.
*/
public var path: List? = null
/**
* The property type (see `PropertyType`).
*/
public var type: aws.sdk.kotlin.services.iotsitewise.model.PropertyType? = null
/**
* The unit of the asset model property, such as `Newtons` or `RPM`.
*/
public var unit: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.AssetModelProperty) : this() {
this.dataType = x.dataType
this.dataTypeSpec = x.dataTypeSpec
this.externalId = x.externalId
this.id = x.id
this.name = x.name
this.path = x.path
this.type = x.type
this.unit = x.unit
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.AssetModelProperty = AssetModelProperty(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.PropertyType] inside the given [block]
*/
public fun type(block: aws.sdk.kotlin.services.iotsitewise.model.PropertyType.Builder.() -> kotlin.Unit) {
this.type = aws.sdk.kotlin.services.iotsitewise.model.PropertyType.invoke(block)
}
internal fun correctErrors(): Builder {
if (dataType == null) dataType = PropertyDataType.SdkUnknown("no value provided")
if (name == null) name = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy