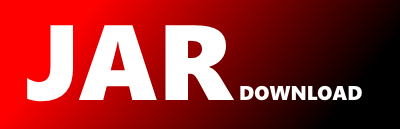
commonMain.aws.sdk.kotlin.services.iotsitewise.model.AssetModelPropertyDefinition.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains an asset model property definition. This property definition is applied to all assets created from the asset model.
*/
public class AssetModelPropertyDefinition private constructor(builder: Builder) {
/**
* The data type of the property definition.
*
* If you specify `STRUCT`, you must also specify `dataTypeSpec` to identify the type of the structure for this property.
*/
public val dataType: aws.sdk.kotlin.services.iotsitewise.model.PropertyDataType = requireNotNull(builder.dataType) { "A non-null value must be provided for dataType" }
/**
* The data type of the structure for this property. This parameter is required on properties that have the `STRUCT` data type.
*
* The options for this parameter depend on the type of the composite model in which you define this property. Use `AWS/ALARM_STATE` for alarm state in alarm composite models.
*/
public val dataTypeSpec: kotlin.String? = builder.dataTypeSpec
/**
* An external ID to assign to the property definition. The external ID must be unique among property definitions within this asset model. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public val externalId: kotlin.String? = builder.externalId
/**
* The ID to assign to the asset model property, if desired. IoT SiteWise automatically generates a unique ID for you, so this parameter is never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If you specify your own ID, it must be globally unique.
*/
public val id: kotlin.String? = builder.id
/**
* The name of the property definition.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* The property definition type (see `PropertyType`). You can only specify one type in a property definition.
*/
public val type: aws.sdk.kotlin.services.iotsitewise.model.PropertyType? = builder.type
/**
* The unit of the property definition, such as `Newtons` or `RPM`.
*/
public val unit: kotlin.String? = builder.unit
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.AssetModelPropertyDefinition = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AssetModelPropertyDefinition(")
append("dataType=$dataType,")
append("dataTypeSpec=$dataTypeSpec,")
append("externalId=$externalId,")
append("id=$id,")
append("name=$name,")
append("type=$type,")
append("unit=$unit")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = dataType.hashCode()
result = 31 * result + (dataTypeSpec?.hashCode() ?: 0)
result = 31 * result + (externalId?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (type?.hashCode() ?: 0)
result = 31 * result + (unit?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AssetModelPropertyDefinition
if (dataType != other.dataType) return false
if (dataTypeSpec != other.dataTypeSpec) return false
if (externalId != other.externalId) return false
if (id != other.id) return false
if (name != other.name) return false
if (type != other.type) return false
if (unit != other.unit) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.AssetModelPropertyDefinition = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The data type of the property definition.
*
* If you specify `STRUCT`, you must also specify `dataTypeSpec` to identify the type of the structure for this property.
*/
public var dataType: aws.sdk.kotlin.services.iotsitewise.model.PropertyDataType? = null
/**
* The data type of the structure for this property. This parameter is required on properties that have the `STRUCT` data type.
*
* The options for this parameter depend on the type of the composite model in which you define this property. Use `AWS/ALARM_STATE` for alarm state in alarm composite models.
*/
public var dataTypeSpec: kotlin.String? = null
/**
* An external ID to assign to the property definition. The external ID must be unique among property definitions within this asset model. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public var externalId: kotlin.String? = null
/**
* The ID to assign to the asset model property, if desired. IoT SiteWise automatically generates a unique ID for you, so this parameter is never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If you specify your own ID, it must be globally unique.
*/
public var id: kotlin.String? = null
/**
* The name of the property definition.
*/
public var name: kotlin.String? = null
/**
* The property definition type (see `PropertyType`). You can only specify one type in a property definition.
*/
public var type: aws.sdk.kotlin.services.iotsitewise.model.PropertyType? = null
/**
* The unit of the property definition, such as `Newtons` or `RPM`.
*/
public var unit: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.AssetModelPropertyDefinition) : this() {
this.dataType = x.dataType
this.dataTypeSpec = x.dataTypeSpec
this.externalId = x.externalId
this.id = x.id
this.name = x.name
this.type = x.type
this.unit = x.unit
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.AssetModelPropertyDefinition = AssetModelPropertyDefinition(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.PropertyType] inside the given [block]
*/
public fun type(block: aws.sdk.kotlin.services.iotsitewise.model.PropertyType.Builder.() -> kotlin.Unit) {
this.type = aws.sdk.kotlin.services.iotsitewise.model.PropertyType.invoke(block)
}
internal fun correctErrors(): Builder {
if (dataType == null) dataType = PropertyDataType.SdkUnknown("no value provided")
if (name == null) name = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy