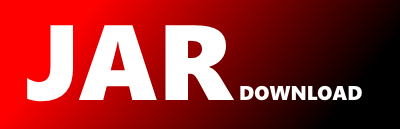
commonMain.aws.sdk.kotlin.services.iotsitewise.model.DescribeAccessPolicyResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeAccessPolicyResponse private constructor(builder: Builder) {
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the access policy, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:access-policy/${AccessPolicyId}`
*/
public val accessPolicyArn: kotlin.String = requireNotNull(builder.accessPolicyArn) { "A non-null value must be provided for accessPolicyArn" }
/**
* The date the access policy was created, in Unix epoch time.
*/
public val accessPolicyCreationDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.accessPolicyCreationDate) { "A non-null value must be provided for accessPolicyCreationDate" }
/**
* The ID of the access policy.
*/
public val accessPolicyId: kotlin.String = requireNotNull(builder.accessPolicyId) { "A non-null value must be provided for accessPolicyId" }
/**
* The identity (IAM Identity Center user, IAM Identity Center group, or IAM user) to which this access policy applies.
*/
public val accessPolicyIdentity: aws.sdk.kotlin.services.iotsitewise.model.Identity? = builder.accessPolicyIdentity
/**
* The date the access policy was last updated, in Unix epoch time.
*/
public val accessPolicyLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.accessPolicyLastUpdateDate) { "A non-null value must be provided for accessPolicyLastUpdateDate" }
/**
* The access policy permission. Note that a project `ADMINISTRATOR` is also known as a project owner.
*/
public val accessPolicyPermission: aws.sdk.kotlin.services.iotsitewise.model.Permission = requireNotNull(builder.accessPolicyPermission) { "A non-null value must be provided for accessPolicyPermission" }
/**
* The IoT SiteWise Monitor resource (portal or project) to which this access policy provides access.
*/
public val accessPolicyResource: aws.sdk.kotlin.services.iotsitewise.model.Resource? = builder.accessPolicyResource
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.DescribeAccessPolicyResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeAccessPolicyResponse(")
append("accessPolicyArn=$accessPolicyArn,")
append("accessPolicyCreationDate=$accessPolicyCreationDate,")
append("accessPolicyId=$accessPolicyId,")
append("accessPolicyIdentity=$accessPolicyIdentity,")
append("accessPolicyLastUpdateDate=$accessPolicyLastUpdateDate,")
append("accessPolicyPermission=$accessPolicyPermission,")
append("accessPolicyResource=$accessPolicyResource")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accessPolicyArn.hashCode()
result = 31 * result + (accessPolicyCreationDate.hashCode())
result = 31 * result + (accessPolicyId.hashCode())
result = 31 * result + (accessPolicyIdentity?.hashCode() ?: 0)
result = 31 * result + (accessPolicyLastUpdateDate.hashCode())
result = 31 * result + (accessPolicyPermission.hashCode())
result = 31 * result + (accessPolicyResource?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeAccessPolicyResponse
if (accessPolicyArn != other.accessPolicyArn) return false
if (accessPolicyCreationDate != other.accessPolicyCreationDate) return false
if (accessPolicyId != other.accessPolicyId) return false
if (accessPolicyIdentity != other.accessPolicyIdentity) return false
if (accessPolicyLastUpdateDate != other.accessPolicyLastUpdateDate) return false
if (accessPolicyPermission != other.accessPolicyPermission) return false
if (accessPolicyResource != other.accessPolicyResource) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.DescribeAccessPolicyResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the access policy, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:access-policy/${AccessPolicyId}`
*/
public var accessPolicyArn: kotlin.String? = null
/**
* The date the access policy was created, in Unix epoch time.
*/
public var accessPolicyCreationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the access policy.
*/
public var accessPolicyId: kotlin.String? = null
/**
* The identity (IAM Identity Center user, IAM Identity Center group, or IAM user) to which this access policy applies.
*/
public var accessPolicyIdentity: aws.sdk.kotlin.services.iotsitewise.model.Identity? = null
/**
* The date the access policy was last updated, in Unix epoch time.
*/
public var accessPolicyLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The access policy permission. Note that a project `ADMINISTRATOR` is also known as a project owner.
*/
public var accessPolicyPermission: aws.sdk.kotlin.services.iotsitewise.model.Permission? = null
/**
* The IoT SiteWise Monitor resource (portal or project) to which this access policy provides access.
*/
public var accessPolicyResource: aws.sdk.kotlin.services.iotsitewise.model.Resource? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.DescribeAccessPolicyResponse) : this() {
this.accessPolicyArn = x.accessPolicyArn
this.accessPolicyCreationDate = x.accessPolicyCreationDate
this.accessPolicyId = x.accessPolicyId
this.accessPolicyIdentity = x.accessPolicyIdentity
this.accessPolicyLastUpdateDate = x.accessPolicyLastUpdateDate
this.accessPolicyPermission = x.accessPolicyPermission
this.accessPolicyResource = x.accessPolicyResource
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.DescribeAccessPolicyResponse = DescribeAccessPolicyResponse(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.Identity] inside the given [block]
*/
public fun accessPolicyIdentity(block: aws.sdk.kotlin.services.iotsitewise.model.Identity.Builder.() -> kotlin.Unit) {
this.accessPolicyIdentity = aws.sdk.kotlin.services.iotsitewise.model.Identity.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.Resource] inside the given [block]
*/
public fun accessPolicyResource(block: aws.sdk.kotlin.services.iotsitewise.model.Resource.Builder.() -> kotlin.Unit) {
this.accessPolicyResource = aws.sdk.kotlin.services.iotsitewise.model.Resource.invoke(block)
}
internal fun correctErrors(): Builder {
if (accessPolicyArn == null) accessPolicyArn = ""
if (accessPolicyCreationDate == null) accessPolicyCreationDate = Instant.fromEpochSeconds(0)
if (accessPolicyId == null) accessPolicyId = ""
if (accessPolicyLastUpdateDate == null) accessPolicyLastUpdateDate = Instant.fromEpochSeconds(0)
if (accessPolicyPermission == null) accessPolicyPermission = Permission.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy