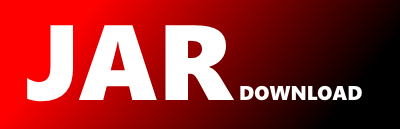
commonMain.aws.sdk.kotlin.services.iotsitewise.model.DescribeAssetResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeAssetResponse private constructor(builder: Builder) {
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the asset, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:asset/${AssetId}`
*/
public val assetArn: kotlin.String = requireNotNull(builder.assetArn) { "A non-null value must be provided for assetArn" }
/**
* The list of the immediate child custom composite model summaries for the asset.
*/
public val assetCompositeModelSummaries: List? = builder.assetCompositeModelSummaries
/**
* The composite models for the asset.
*/
public val assetCompositeModels: List? = builder.assetCompositeModels
/**
* The date the asset was created, in Unix epoch time.
*/
public val assetCreationDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.assetCreationDate) { "A non-null value must be provided for assetCreationDate" }
/**
* A description for the asset.
*/
public val assetDescription: kotlin.String? = builder.assetDescription
/**
* The external ID of the asset, if any.
*/
public val assetExternalId: kotlin.String? = builder.assetExternalId
/**
* A list of asset hierarchies that each contain a `hierarchyId`. A hierarchy specifies allowed parent/child asset relationships.
*/
public val assetHierarchies: List = requireNotNull(builder.assetHierarchies) { "A non-null value must be provided for assetHierarchies" }
/**
* The ID of the asset, in UUID format.
*/
public val assetId: kotlin.String = requireNotNull(builder.assetId) { "A non-null value must be provided for assetId" }
/**
* The date the asset was last updated, in Unix epoch time.
*/
public val assetLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.assetLastUpdateDate) { "A non-null value must be provided for assetLastUpdateDate" }
/**
* The ID of the asset model that was used to create the asset.
*/
public val assetModelId: kotlin.String = requireNotNull(builder.assetModelId) { "A non-null value must be provided for assetModelId" }
/**
* The name of the asset.
*/
public val assetName: kotlin.String = requireNotNull(builder.assetName) { "A non-null value must be provided for assetName" }
/**
* The list of asset properties for the asset.
*
* This object doesn't include properties that you define in composite models. You can find composite model properties in the `assetCompositeModels` object.
*/
public val assetProperties: List = requireNotNull(builder.assetProperties) { "A non-null value must be provided for assetProperties" }
/**
* The current status of the asset, which contains a state and any error message.
*/
public val assetStatus: aws.sdk.kotlin.services.iotsitewise.model.AssetStatus? = builder.assetStatus
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.DescribeAssetResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeAssetResponse(")
append("assetArn=$assetArn,")
append("assetCompositeModelSummaries=$assetCompositeModelSummaries,")
append("assetCompositeModels=$assetCompositeModels,")
append("assetCreationDate=$assetCreationDate,")
append("assetDescription=$assetDescription,")
append("assetExternalId=$assetExternalId,")
append("assetHierarchies=$assetHierarchies,")
append("assetId=$assetId,")
append("assetLastUpdateDate=$assetLastUpdateDate,")
append("assetModelId=$assetModelId,")
append("assetName=$assetName,")
append("assetProperties=$assetProperties,")
append("assetStatus=$assetStatus")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = assetArn.hashCode()
result = 31 * result + (assetCompositeModelSummaries?.hashCode() ?: 0)
result = 31 * result + (assetCompositeModels?.hashCode() ?: 0)
result = 31 * result + (assetCreationDate.hashCode())
result = 31 * result + (assetDescription?.hashCode() ?: 0)
result = 31 * result + (assetExternalId?.hashCode() ?: 0)
result = 31 * result + (assetHierarchies.hashCode())
result = 31 * result + (assetId.hashCode())
result = 31 * result + (assetLastUpdateDate.hashCode())
result = 31 * result + (assetModelId.hashCode())
result = 31 * result + (assetName.hashCode())
result = 31 * result + (assetProperties.hashCode())
result = 31 * result + (assetStatus?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeAssetResponse
if (assetArn != other.assetArn) return false
if (assetCompositeModelSummaries != other.assetCompositeModelSummaries) return false
if (assetCompositeModels != other.assetCompositeModels) return false
if (assetCreationDate != other.assetCreationDate) return false
if (assetDescription != other.assetDescription) return false
if (assetExternalId != other.assetExternalId) return false
if (assetHierarchies != other.assetHierarchies) return false
if (assetId != other.assetId) return false
if (assetLastUpdateDate != other.assetLastUpdateDate) return false
if (assetModelId != other.assetModelId) return false
if (assetName != other.assetName) return false
if (assetProperties != other.assetProperties) return false
if (assetStatus != other.assetStatus) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.DescribeAssetResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the asset, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:asset/${AssetId}`
*/
public var assetArn: kotlin.String? = null
/**
* The list of the immediate child custom composite model summaries for the asset.
*/
public var assetCompositeModelSummaries: List? = null
/**
* The composite models for the asset.
*/
public var assetCompositeModels: List? = null
/**
* The date the asset was created, in Unix epoch time.
*/
public var assetCreationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A description for the asset.
*/
public var assetDescription: kotlin.String? = null
/**
* The external ID of the asset, if any.
*/
public var assetExternalId: kotlin.String? = null
/**
* A list of asset hierarchies that each contain a `hierarchyId`. A hierarchy specifies allowed parent/child asset relationships.
*/
public var assetHierarchies: List? = null
/**
* The ID of the asset, in UUID format.
*/
public var assetId: kotlin.String? = null
/**
* The date the asset was last updated, in Unix epoch time.
*/
public var assetLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the asset model that was used to create the asset.
*/
public var assetModelId: kotlin.String? = null
/**
* The name of the asset.
*/
public var assetName: kotlin.String? = null
/**
* The list of asset properties for the asset.
*
* This object doesn't include properties that you define in composite models. You can find composite model properties in the `assetCompositeModels` object.
*/
public var assetProperties: List? = null
/**
* The current status of the asset, which contains a state and any error message.
*/
public var assetStatus: aws.sdk.kotlin.services.iotsitewise.model.AssetStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.DescribeAssetResponse) : this() {
this.assetArn = x.assetArn
this.assetCompositeModelSummaries = x.assetCompositeModelSummaries
this.assetCompositeModels = x.assetCompositeModels
this.assetCreationDate = x.assetCreationDate
this.assetDescription = x.assetDescription
this.assetExternalId = x.assetExternalId
this.assetHierarchies = x.assetHierarchies
this.assetId = x.assetId
this.assetLastUpdateDate = x.assetLastUpdateDate
this.assetModelId = x.assetModelId
this.assetName = x.assetName
this.assetProperties = x.assetProperties
this.assetStatus = x.assetStatus
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.DescribeAssetResponse = DescribeAssetResponse(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.AssetStatus] inside the given [block]
*/
public fun assetStatus(block: aws.sdk.kotlin.services.iotsitewise.model.AssetStatus.Builder.() -> kotlin.Unit) {
this.assetStatus = aws.sdk.kotlin.services.iotsitewise.model.AssetStatus.invoke(block)
}
internal fun correctErrors(): Builder {
if (assetArn == null) assetArn = ""
if (assetCreationDate == null) assetCreationDate = Instant.fromEpochSeconds(0)
if (assetHierarchies == null) assetHierarchies = emptyList()
if (assetId == null) assetId = ""
if (assetLastUpdateDate == null) assetLastUpdateDate = Instant.fromEpochSeconds(0)
if (assetModelId == null) assetModelId = ""
if (assetName == null) assetName = ""
if (assetProperties == null) assetProperties = emptyList()
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy