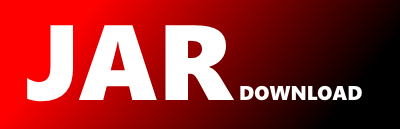
commonMain.aws.sdk.kotlin.services.iotsitewise.model.DescribeBulkImportJobResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeBulkImportJobResponse private constructor(builder: Builder) {
/**
* If set to true, ingest new data into IoT SiteWise storage. Measurements with notifications, metrics and transforms are computed. If set to false, historical data is ingested into IoT SiteWise as is.
*/
public val adaptiveIngestion: kotlin.Boolean? = builder.adaptiveIngestion
/**
* If set to true, your data files is deleted from S3, after ingestion into IoT SiteWise storage.
*/
public val deleteFilesAfterImport: kotlin.Boolean? = builder.deleteFilesAfterImport
/**
* The Amazon S3 destination where errors associated with the job creation request are saved.
*/
public val errorReportLocation: aws.sdk.kotlin.services.iotsitewise.model.ErrorReportLocation? = builder.errorReportLocation
/**
* The files in the specified Amazon S3 bucket that contain your data.
*/
public val files: List = requireNotNull(builder.files) { "A non-null value must be provided for files" }
/**
* Contains the configuration information of a job, such as the file format used to save data in Amazon S3.
*/
public val jobConfiguration: aws.sdk.kotlin.services.iotsitewise.model.JobConfiguration? = builder.jobConfiguration
/**
* The date the job was created, in Unix epoch TIME.
*/
public val jobCreationDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.jobCreationDate) { "A non-null value must be provided for jobCreationDate" }
/**
* The ID of the job.
*/
public val jobId: kotlin.String = requireNotNull(builder.jobId) { "A non-null value must be provided for jobId" }
/**
* The date the job was last updated, in Unix epoch time.
*/
public val jobLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.jobLastUpdateDate) { "A non-null value must be provided for jobLastUpdateDate" }
/**
* The unique name that helps identify the job request.
*/
public val jobName: kotlin.String = requireNotNull(builder.jobName) { "A non-null value must be provided for jobName" }
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the IAM role that allows IoT SiteWise to read Amazon S3 data.
*/
public val jobRoleArn: kotlin.String = requireNotNull(builder.jobRoleArn) { "A non-null value must be provided for jobRoleArn" }
/**
* The status of the bulk import job can be one of following values:
* + `PENDING` – IoT SiteWise is waiting for the current bulk import job to finish.
* + `CANCELLED` – The bulk import job has been canceled.
* + `RUNNING` – IoT SiteWise is processing your request to import your data from Amazon S3.
* + `COMPLETED` – IoT SiteWise successfully completed your request to import data from Amazon S3.
* + `FAILED` – IoT SiteWise couldn't process your request to import data from Amazon S3. You can use logs saved in the specified error report location in Amazon S3 to troubleshoot issues.
* + `COMPLETED_WITH_FAILURES` – IoT SiteWise completed your request to import data from Amazon S3 with errors. You can use logs saved in the specified error report location in Amazon S3 to troubleshoot issues.
*/
public val jobStatus: aws.sdk.kotlin.services.iotsitewise.model.JobStatus = requireNotNull(builder.jobStatus) { "A non-null value must be provided for jobStatus" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.DescribeBulkImportJobResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeBulkImportJobResponse(")
append("adaptiveIngestion=$adaptiveIngestion,")
append("deleteFilesAfterImport=$deleteFilesAfterImport,")
append("errorReportLocation=$errorReportLocation,")
append("files=$files,")
append("jobConfiguration=$jobConfiguration,")
append("jobCreationDate=$jobCreationDate,")
append("jobId=$jobId,")
append("jobLastUpdateDate=$jobLastUpdateDate,")
append("jobName=$jobName,")
append("jobRoleArn=$jobRoleArn,")
append("jobStatus=$jobStatus")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = adaptiveIngestion?.hashCode() ?: 0
result = 31 * result + (deleteFilesAfterImport?.hashCode() ?: 0)
result = 31 * result + (errorReportLocation?.hashCode() ?: 0)
result = 31 * result + (files.hashCode())
result = 31 * result + (jobConfiguration?.hashCode() ?: 0)
result = 31 * result + (jobCreationDate.hashCode())
result = 31 * result + (jobId.hashCode())
result = 31 * result + (jobLastUpdateDate.hashCode())
result = 31 * result + (jobName.hashCode())
result = 31 * result + (jobRoleArn.hashCode())
result = 31 * result + (jobStatus.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeBulkImportJobResponse
if (adaptiveIngestion != other.adaptiveIngestion) return false
if (deleteFilesAfterImport != other.deleteFilesAfterImport) return false
if (errorReportLocation != other.errorReportLocation) return false
if (files != other.files) return false
if (jobConfiguration != other.jobConfiguration) return false
if (jobCreationDate != other.jobCreationDate) return false
if (jobId != other.jobId) return false
if (jobLastUpdateDate != other.jobLastUpdateDate) return false
if (jobName != other.jobName) return false
if (jobRoleArn != other.jobRoleArn) return false
if (jobStatus != other.jobStatus) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.DescribeBulkImportJobResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* If set to true, ingest new data into IoT SiteWise storage. Measurements with notifications, metrics and transforms are computed. If set to false, historical data is ingested into IoT SiteWise as is.
*/
public var adaptiveIngestion: kotlin.Boolean? = null
/**
* If set to true, your data files is deleted from S3, after ingestion into IoT SiteWise storage.
*/
public var deleteFilesAfterImport: kotlin.Boolean? = null
/**
* The Amazon S3 destination where errors associated with the job creation request are saved.
*/
public var errorReportLocation: aws.sdk.kotlin.services.iotsitewise.model.ErrorReportLocation? = null
/**
* The files in the specified Amazon S3 bucket that contain your data.
*/
public var files: List? = null
/**
* Contains the configuration information of a job, such as the file format used to save data in Amazon S3.
*/
public var jobConfiguration: aws.sdk.kotlin.services.iotsitewise.model.JobConfiguration? = null
/**
* The date the job was created, in Unix epoch TIME.
*/
public var jobCreationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the job.
*/
public var jobId: kotlin.String? = null
/**
* The date the job was last updated, in Unix epoch time.
*/
public var jobLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The unique name that helps identify the job request.
*/
public var jobName: kotlin.String? = null
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the IAM role that allows IoT SiteWise to read Amazon S3 data.
*/
public var jobRoleArn: kotlin.String? = null
/**
* The status of the bulk import job can be one of following values:
* + `PENDING` – IoT SiteWise is waiting for the current bulk import job to finish.
* + `CANCELLED` – The bulk import job has been canceled.
* + `RUNNING` – IoT SiteWise is processing your request to import your data from Amazon S3.
* + `COMPLETED` – IoT SiteWise successfully completed your request to import data from Amazon S3.
* + `FAILED` – IoT SiteWise couldn't process your request to import data from Amazon S3. You can use logs saved in the specified error report location in Amazon S3 to troubleshoot issues.
* + `COMPLETED_WITH_FAILURES` – IoT SiteWise completed your request to import data from Amazon S3 with errors. You can use logs saved in the specified error report location in Amazon S3 to troubleshoot issues.
*/
public var jobStatus: aws.sdk.kotlin.services.iotsitewise.model.JobStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.DescribeBulkImportJobResponse) : this() {
this.adaptiveIngestion = x.adaptiveIngestion
this.deleteFilesAfterImport = x.deleteFilesAfterImport
this.errorReportLocation = x.errorReportLocation
this.files = x.files
this.jobConfiguration = x.jobConfiguration
this.jobCreationDate = x.jobCreationDate
this.jobId = x.jobId
this.jobLastUpdateDate = x.jobLastUpdateDate
this.jobName = x.jobName
this.jobRoleArn = x.jobRoleArn
this.jobStatus = x.jobStatus
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.DescribeBulkImportJobResponse = DescribeBulkImportJobResponse(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.ErrorReportLocation] inside the given [block]
*/
public fun errorReportLocation(block: aws.sdk.kotlin.services.iotsitewise.model.ErrorReportLocation.Builder.() -> kotlin.Unit) {
this.errorReportLocation = aws.sdk.kotlin.services.iotsitewise.model.ErrorReportLocation.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.JobConfiguration] inside the given [block]
*/
public fun jobConfiguration(block: aws.sdk.kotlin.services.iotsitewise.model.JobConfiguration.Builder.() -> kotlin.Unit) {
this.jobConfiguration = aws.sdk.kotlin.services.iotsitewise.model.JobConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
if (files == null) files = emptyList()
if (jobCreationDate == null) jobCreationDate = Instant.fromEpochSeconds(0)
if (jobId == null) jobId = ""
if (jobLastUpdateDate == null) jobLastUpdateDate = Instant.fromEpochSeconds(0)
if (jobName == null) jobName = ""
if (jobRoleArn == null) jobRoleArn = ""
if (jobStatus == null) jobStatus = JobStatus.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy