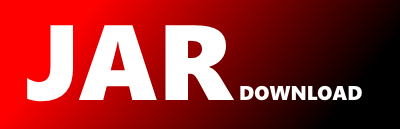
commonMain.aws.sdk.kotlin.services.iotsitewise.model.DescribeGatewayResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeGatewayResponse private constructor(builder: Builder) {
/**
* The date the gateway was created, in Unix epoch time.
*/
public val creationDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.creationDate) { "A non-null value must be provided for creationDate" }
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the gateway, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:gateway/${GatewayId}`
*/
public val gatewayArn: kotlin.String = requireNotNull(builder.gatewayArn) { "A non-null value must be provided for gatewayArn" }
/**
* A list of gateway capability summaries that each contain a namespace and status. Each gateway capability defines data sources for the gateway. To retrieve a capability configuration's definition, use [DescribeGatewayCapabilityConfiguration](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_DescribeGatewayCapabilityConfiguration.html).
*/
public val gatewayCapabilitySummaries: List = requireNotNull(builder.gatewayCapabilitySummaries) { "A non-null value must be provided for gatewayCapabilitySummaries" }
/**
* The ID of the gateway device.
*/
public val gatewayId: kotlin.String = requireNotNull(builder.gatewayId) { "A non-null value must be provided for gatewayId" }
/**
* The name of the gateway.
*/
public val gatewayName: kotlin.String = requireNotNull(builder.gatewayName) { "A non-null value must be provided for gatewayName" }
/**
* The gateway's platform.
*/
public val gatewayPlatform: aws.sdk.kotlin.services.iotsitewise.model.GatewayPlatform? = builder.gatewayPlatform
/**
* The date the gateway was last updated, in Unix epoch time.
*/
public val lastUpdateDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastUpdateDate) { "A non-null value must be provided for lastUpdateDate" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.DescribeGatewayResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeGatewayResponse(")
append("creationDate=$creationDate,")
append("gatewayArn=$gatewayArn,")
append("gatewayCapabilitySummaries=$gatewayCapabilitySummaries,")
append("gatewayId=$gatewayId,")
append("gatewayName=$gatewayName,")
append("gatewayPlatform=$gatewayPlatform,")
append("lastUpdateDate=$lastUpdateDate")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = creationDate.hashCode()
result = 31 * result + (gatewayArn.hashCode())
result = 31 * result + (gatewayCapabilitySummaries.hashCode())
result = 31 * result + (gatewayId.hashCode())
result = 31 * result + (gatewayName.hashCode())
result = 31 * result + (gatewayPlatform?.hashCode() ?: 0)
result = 31 * result + (lastUpdateDate.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeGatewayResponse
if (creationDate != other.creationDate) return false
if (gatewayArn != other.gatewayArn) return false
if (gatewayCapabilitySummaries != other.gatewayCapabilitySummaries) return false
if (gatewayId != other.gatewayId) return false
if (gatewayName != other.gatewayName) return false
if (gatewayPlatform != other.gatewayPlatform) return false
if (lastUpdateDate != other.lastUpdateDate) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.DescribeGatewayResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The date the gateway was created, in Unix epoch time.
*/
public var creationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the gateway, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:gateway/${GatewayId}`
*/
public var gatewayArn: kotlin.String? = null
/**
* A list of gateway capability summaries that each contain a namespace and status. Each gateway capability defines data sources for the gateway. To retrieve a capability configuration's definition, use [DescribeGatewayCapabilityConfiguration](https://docs.aws.amazon.com/iot-sitewise/latest/APIReference/API_DescribeGatewayCapabilityConfiguration.html).
*/
public var gatewayCapabilitySummaries: List? = null
/**
* The ID of the gateway device.
*/
public var gatewayId: kotlin.String? = null
/**
* The name of the gateway.
*/
public var gatewayName: kotlin.String? = null
/**
* The gateway's platform.
*/
public var gatewayPlatform: aws.sdk.kotlin.services.iotsitewise.model.GatewayPlatform? = null
/**
* The date the gateway was last updated, in Unix epoch time.
*/
public var lastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.DescribeGatewayResponse) : this() {
this.creationDate = x.creationDate
this.gatewayArn = x.gatewayArn
this.gatewayCapabilitySummaries = x.gatewayCapabilitySummaries
this.gatewayId = x.gatewayId
this.gatewayName = x.gatewayName
this.gatewayPlatform = x.gatewayPlatform
this.lastUpdateDate = x.lastUpdateDate
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.DescribeGatewayResponse = DescribeGatewayResponse(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.GatewayPlatform] inside the given [block]
*/
public fun gatewayPlatform(block: aws.sdk.kotlin.services.iotsitewise.model.GatewayPlatform.Builder.() -> kotlin.Unit) {
this.gatewayPlatform = aws.sdk.kotlin.services.iotsitewise.model.GatewayPlatform.invoke(block)
}
internal fun correctErrors(): Builder {
if (creationDate == null) creationDate = Instant.fromEpochSeconds(0)
if (gatewayArn == null) gatewayArn = ""
if (gatewayCapabilitySummaries == null) gatewayCapabilitySummaries = emptyList()
if (gatewayId == null) gatewayId = ""
if (gatewayName == null) gatewayName = ""
if (lastUpdateDate == null) lastUpdateDate = Instant.fromEpochSeconds(0)
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy