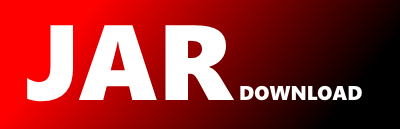
commonMain.aws.sdk.kotlin.services.iotsitewise.model.DescribePortalResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribePortalResponse private constructor(builder: Builder) {
/**
* Contains the configuration information of an alarm created in an IoT SiteWise Monitor portal.
*/
public val alarms: aws.sdk.kotlin.services.iotsitewise.model.Alarms? = builder.alarms
/**
* The email address that sends alarm notifications.
*/
public val notificationSenderEmail: kotlin.String? = builder.notificationSenderEmail
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the portal, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:portal/${PortalId}`
*/
public val portalArn: kotlin.String = requireNotNull(builder.portalArn) { "A non-null value must be provided for portalArn" }
/**
* The service to use to authenticate users to the portal.
*/
public val portalAuthMode: aws.sdk.kotlin.services.iotsitewise.model.AuthMode? = builder.portalAuthMode
/**
* The IAM Identity Center application generated client ID (used with IAM Identity Center API operations). IoT SiteWise includes `portalClientId` for only portals that use IAM Identity Center to authenticate users.
*/
public val portalClientId: kotlin.String = requireNotNull(builder.portalClientId) { "A non-null value must be provided for portalClientId" }
/**
* The Amazon Web Services administrator's contact email address.
*/
public val portalContactEmail: kotlin.String = requireNotNull(builder.portalContactEmail) { "A non-null value must be provided for portalContactEmail" }
/**
* The date the portal was created, in Unix epoch time.
*/
public val portalCreationDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.portalCreationDate) { "A non-null value must be provided for portalCreationDate" }
/**
* The portal's description.
*/
public val portalDescription: kotlin.String? = builder.portalDescription
/**
* The ID of the portal.
*/
public val portalId: kotlin.String = requireNotNull(builder.portalId) { "A non-null value must be provided for portalId" }
/**
* The date the portal was last updated, in Unix epoch time.
*/
public val portalLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.portalLastUpdateDate) { "A non-null value must be provided for portalLastUpdateDate" }
/**
* The portal's logo image, which is available at a URL.
*/
public val portalLogoImageLocation: aws.sdk.kotlin.services.iotsitewise.model.ImageLocation? = builder.portalLogoImageLocation
/**
* The name of the portal.
*/
public val portalName: kotlin.String = requireNotNull(builder.portalName) { "A non-null value must be provided for portalName" }
/**
* The URL for the IoT SiteWise Monitor portal. You can use this URL to access portals that use IAM Identity Center for authentication. For portals that use IAM for authentication, you must use the IoT SiteWise console to get a URL that you can use to access the portal.
*/
public val portalStartUrl: kotlin.String = requireNotNull(builder.portalStartUrl) { "A non-null value must be provided for portalStartUrl" }
/**
* The current status of the portal, which contains a state and any error message.
*/
public val portalStatus: aws.sdk.kotlin.services.iotsitewise.model.PortalStatus? = builder.portalStatus
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the service role that allows the portal's users to access your IoT SiteWise resources on your behalf. For more information, see [Using service roles for IoT SiteWise Monitor](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/monitor-service-role.html) in the *IoT SiteWise User Guide*.
*/
public val roleArn: kotlin.String? = builder.roleArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.DescribePortalResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribePortalResponse(")
append("alarms=$alarms,")
append("notificationSenderEmail=$notificationSenderEmail,")
append("portalArn=$portalArn,")
append("portalAuthMode=$portalAuthMode,")
append("portalClientId=$portalClientId,")
append("portalContactEmail=$portalContactEmail,")
append("portalCreationDate=$portalCreationDate,")
append("portalDescription=$portalDescription,")
append("portalId=$portalId,")
append("portalLastUpdateDate=$portalLastUpdateDate,")
append("portalLogoImageLocation=$portalLogoImageLocation,")
append("portalName=$portalName,")
append("portalStartUrl=$portalStartUrl,")
append("portalStatus=$portalStatus,")
append("roleArn=$roleArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = alarms?.hashCode() ?: 0
result = 31 * result + (notificationSenderEmail?.hashCode() ?: 0)
result = 31 * result + (portalArn.hashCode())
result = 31 * result + (portalAuthMode?.hashCode() ?: 0)
result = 31 * result + (portalClientId.hashCode())
result = 31 * result + (portalContactEmail.hashCode())
result = 31 * result + (portalCreationDate.hashCode())
result = 31 * result + (portalDescription?.hashCode() ?: 0)
result = 31 * result + (portalId.hashCode())
result = 31 * result + (portalLastUpdateDate.hashCode())
result = 31 * result + (portalLogoImageLocation?.hashCode() ?: 0)
result = 31 * result + (portalName.hashCode())
result = 31 * result + (portalStartUrl.hashCode())
result = 31 * result + (portalStatus?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribePortalResponse
if (alarms != other.alarms) return false
if (notificationSenderEmail != other.notificationSenderEmail) return false
if (portalArn != other.portalArn) return false
if (portalAuthMode != other.portalAuthMode) return false
if (portalClientId != other.portalClientId) return false
if (portalContactEmail != other.portalContactEmail) return false
if (portalCreationDate != other.portalCreationDate) return false
if (portalDescription != other.portalDescription) return false
if (portalId != other.portalId) return false
if (portalLastUpdateDate != other.portalLastUpdateDate) return false
if (portalLogoImageLocation != other.portalLogoImageLocation) return false
if (portalName != other.portalName) return false
if (portalStartUrl != other.portalStartUrl) return false
if (portalStatus != other.portalStatus) return false
if (roleArn != other.roleArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.DescribePortalResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Contains the configuration information of an alarm created in an IoT SiteWise Monitor portal.
*/
public var alarms: aws.sdk.kotlin.services.iotsitewise.model.Alarms? = null
/**
* The email address that sends alarm notifications.
*/
public var notificationSenderEmail: kotlin.String? = null
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the portal, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:portal/${PortalId}`
*/
public var portalArn: kotlin.String? = null
/**
* The service to use to authenticate users to the portal.
*/
public var portalAuthMode: aws.sdk.kotlin.services.iotsitewise.model.AuthMode? = null
/**
* The IAM Identity Center application generated client ID (used with IAM Identity Center API operations). IoT SiteWise includes `portalClientId` for only portals that use IAM Identity Center to authenticate users.
*/
public var portalClientId: kotlin.String? = null
/**
* The Amazon Web Services administrator's contact email address.
*/
public var portalContactEmail: kotlin.String? = null
/**
* The date the portal was created, in Unix epoch time.
*/
public var portalCreationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The portal's description.
*/
public var portalDescription: kotlin.String? = null
/**
* The ID of the portal.
*/
public var portalId: kotlin.String? = null
/**
* The date the portal was last updated, in Unix epoch time.
*/
public var portalLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The portal's logo image, which is available at a URL.
*/
public var portalLogoImageLocation: aws.sdk.kotlin.services.iotsitewise.model.ImageLocation? = null
/**
* The name of the portal.
*/
public var portalName: kotlin.String? = null
/**
* The URL for the IoT SiteWise Monitor portal. You can use this URL to access portals that use IAM Identity Center for authentication. For portals that use IAM for authentication, you must use the IoT SiteWise console to get a URL that you can use to access the portal.
*/
public var portalStartUrl: kotlin.String? = null
/**
* The current status of the portal, which contains a state and any error message.
*/
public var portalStatus: aws.sdk.kotlin.services.iotsitewise.model.PortalStatus? = null
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the service role that allows the portal's users to access your IoT SiteWise resources on your behalf. For more information, see [Using service roles for IoT SiteWise Monitor](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/monitor-service-role.html) in the *IoT SiteWise User Guide*.
*/
public var roleArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.DescribePortalResponse) : this() {
this.alarms = x.alarms
this.notificationSenderEmail = x.notificationSenderEmail
this.portalArn = x.portalArn
this.portalAuthMode = x.portalAuthMode
this.portalClientId = x.portalClientId
this.portalContactEmail = x.portalContactEmail
this.portalCreationDate = x.portalCreationDate
this.portalDescription = x.portalDescription
this.portalId = x.portalId
this.portalLastUpdateDate = x.portalLastUpdateDate
this.portalLogoImageLocation = x.portalLogoImageLocation
this.portalName = x.portalName
this.portalStartUrl = x.portalStartUrl
this.portalStatus = x.portalStatus
this.roleArn = x.roleArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.DescribePortalResponse = DescribePortalResponse(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.Alarms] inside the given [block]
*/
public fun alarms(block: aws.sdk.kotlin.services.iotsitewise.model.Alarms.Builder.() -> kotlin.Unit) {
this.alarms = aws.sdk.kotlin.services.iotsitewise.model.Alarms.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.ImageLocation] inside the given [block]
*/
public fun portalLogoImageLocation(block: aws.sdk.kotlin.services.iotsitewise.model.ImageLocation.Builder.() -> kotlin.Unit) {
this.portalLogoImageLocation = aws.sdk.kotlin.services.iotsitewise.model.ImageLocation.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.PortalStatus] inside the given [block]
*/
public fun portalStatus(block: aws.sdk.kotlin.services.iotsitewise.model.PortalStatus.Builder.() -> kotlin.Unit) {
this.portalStatus = aws.sdk.kotlin.services.iotsitewise.model.PortalStatus.invoke(block)
}
internal fun correctErrors(): Builder {
if (portalArn == null) portalArn = ""
if (portalClientId == null) portalClientId = ""
if (portalContactEmail == null) portalContactEmail = ""
if (portalCreationDate == null) portalCreationDate = Instant.fromEpochSeconds(0)
if (portalId == null) portalId = ""
if (portalLastUpdateDate == null) portalLastUpdateDate = Instant.fromEpochSeconds(0)
if (portalName == null) portalName = ""
if (portalStartUrl == null) portalStartUrl = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy