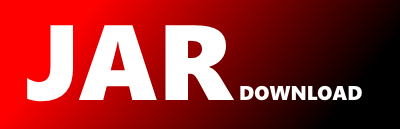
commonMain.aws.sdk.kotlin.services.iotsitewise.model.DescribeStorageConfigurationResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeStorageConfigurationResponse private constructor(builder: Builder) {
/**
* Contains current status information for the configuration.
*/
public val configurationStatus: aws.sdk.kotlin.services.iotsitewise.model.ConfigurationStatus? = builder.configurationStatus
/**
* Contains the storage configuration for time series (data streams) that aren't associated with asset properties. The `disassociatedDataStorage` can be one of the following values:
* + `ENABLED` – IoT SiteWise accepts time series that aren't associated with asset properties.After the `disassociatedDataStorage` is enabled, you can't disable it.
* + `DISABLED` – IoT SiteWise doesn't accept time series (data streams) that aren't associated with asset properties.
*
* For more information, see [Data streams](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/data-streams.html) in the *IoT SiteWise User Guide*.
*/
public val disassociatedDataStorage: aws.sdk.kotlin.services.iotsitewise.model.DisassociatedDataStorageState? = builder.disassociatedDataStorage
/**
* The date the storage configuration was last updated, in Unix epoch time.
*/
public val lastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateDate
/**
* Contains information about the storage destination.
*/
public val multiLayerStorage: aws.sdk.kotlin.services.iotsitewise.model.MultiLayerStorage? = builder.multiLayerStorage
/**
* The number of days your data is kept in the hot tier. By default, your data is kept indefinitely in the hot tier.
*/
public val retentionPeriod: aws.sdk.kotlin.services.iotsitewise.model.RetentionPeriod? = builder.retentionPeriod
/**
* The storage tier that you specified for your data. The `storageType` parameter can be one of the following values:
* + `SITEWISE_DEFAULT_STORAGE` – IoT SiteWise saves your data into the hot tier. The hot tier is a service-managed database.
* + `MULTI_LAYER_STORAGE` – IoT SiteWise saves your data in both the cold tier and the hot tier. The cold tier is a customer-managed Amazon S3 bucket.
*/
public val storageType: aws.sdk.kotlin.services.iotsitewise.model.StorageType = requireNotNull(builder.storageType) { "A non-null value must be provided for storageType" }
/**
* A service managed storage tier optimized for analytical queries. It stores periodically uploaded, buffered and historical data ingested with the CreaeBulkImportJob API.
*/
public val warmTier: aws.sdk.kotlin.services.iotsitewise.model.WarmTierState? = builder.warmTier
/**
* Set this period to specify how long your data is stored in the warm tier before it is deleted. You can set this only if cold tier is enabled.
*/
public val warmTierRetentionPeriod: aws.sdk.kotlin.services.iotsitewise.model.WarmTierRetentionPeriod? = builder.warmTierRetentionPeriod
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.DescribeStorageConfigurationResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeStorageConfigurationResponse(")
append("configurationStatus=$configurationStatus,")
append("disassociatedDataStorage=$disassociatedDataStorage,")
append("lastUpdateDate=$lastUpdateDate,")
append("multiLayerStorage=$multiLayerStorage,")
append("retentionPeriod=$retentionPeriod,")
append("storageType=$storageType,")
append("warmTier=$warmTier,")
append("warmTierRetentionPeriod=$warmTierRetentionPeriod")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = configurationStatus?.hashCode() ?: 0
result = 31 * result + (disassociatedDataStorage?.hashCode() ?: 0)
result = 31 * result + (lastUpdateDate?.hashCode() ?: 0)
result = 31 * result + (multiLayerStorage?.hashCode() ?: 0)
result = 31 * result + (retentionPeriod?.hashCode() ?: 0)
result = 31 * result + (storageType.hashCode())
result = 31 * result + (warmTier?.hashCode() ?: 0)
result = 31 * result + (warmTierRetentionPeriod?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeStorageConfigurationResponse
if (configurationStatus != other.configurationStatus) return false
if (disassociatedDataStorage != other.disassociatedDataStorage) return false
if (lastUpdateDate != other.lastUpdateDate) return false
if (multiLayerStorage != other.multiLayerStorage) return false
if (retentionPeriod != other.retentionPeriod) return false
if (storageType != other.storageType) return false
if (warmTier != other.warmTier) return false
if (warmTierRetentionPeriod != other.warmTierRetentionPeriod) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.DescribeStorageConfigurationResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Contains current status information for the configuration.
*/
public var configurationStatus: aws.sdk.kotlin.services.iotsitewise.model.ConfigurationStatus? = null
/**
* Contains the storage configuration for time series (data streams) that aren't associated with asset properties. The `disassociatedDataStorage` can be one of the following values:
* + `ENABLED` – IoT SiteWise accepts time series that aren't associated with asset properties.After the `disassociatedDataStorage` is enabled, you can't disable it.
* + `DISABLED` – IoT SiteWise doesn't accept time series (data streams) that aren't associated with asset properties.
*
* For more information, see [Data streams](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/data-streams.html) in the *IoT SiteWise User Guide*.
*/
public var disassociatedDataStorage: aws.sdk.kotlin.services.iotsitewise.model.DisassociatedDataStorageState? = null
/**
* The date the storage configuration was last updated, in Unix epoch time.
*/
public var lastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Contains information about the storage destination.
*/
public var multiLayerStorage: aws.sdk.kotlin.services.iotsitewise.model.MultiLayerStorage? = null
/**
* The number of days your data is kept in the hot tier. By default, your data is kept indefinitely in the hot tier.
*/
public var retentionPeriod: aws.sdk.kotlin.services.iotsitewise.model.RetentionPeriod? = null
/**
* The storage tier that you specified for your data. The `storageType` parameter can be one of the following values:
* + `SITEWISE_DEFAULT_STORAGE` – IoT SiteWise saves your data into the hot tier. The hot tier is a service-managed database.
* + `MULTI_LAYER_STORAGE` – IoT SiteWise saves your data in both the cold tier and the hot tier. The cold tier is a customer-managed Amazon S3 bucket.
*/
public var storageType: aws.sdk.kotlin.services.iotsitewise.model.StorageType? = null
/**
* A service managed storage tier optimized for analytical queries. It stores periodically uploaded, buffered and historical data ingested with the CreaeBulkImportJob API.
*/
public var warmTier: aws.sdk.kotlin.services.iotsitewise.model.WarmTierState? = null
/**
* Set this period to specify how long your data is stored in the warm tier before it is deleted. You can set this only if cold tier is enabled.
*/
public var warmTierRetentionPeriod: aws.sdk.kotlin.services.iotsitewise.model.WarmTierRetentionPeriod? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.DescribeStorageConfigurationResponse) : this() {
this.configurationStatus = x.configurationStatus
this.disassociatedDataStorage = x.disassociatedDataStorage
this.lastUpdateDate = x.lastUpdateDate
this.multiLayerStorage = x.multiLayerStorage
this.retentionPeriod = x.retentionPeriod
this.storageType = x.storageType
this.warmTier = x.warmTier
this.warmTierRetentionPeriod = x.warmTierRetentionPeriod
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.DescribeStorageConfigurationResponse = DescribeStorageConfigurationResponse(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.ConfigurationStatus] inside the given [block]
*/
public fun configurationStatus(block: aws.sdk.kotlin.services.iotsitewise.model.ConfigurationStatus.Builder.() -> kotlin.Unit) {
this.configurationStatus = aws.sdk.kotlin.services.iotsitewise.model.ConfigurationStatus.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.MultiLayerStorage] inside the given [block]
*/
public fun multiLayerStorage(block: aws.sdk.kotlin.services.iotsitewise.model.MultiLayerStorage.Builder.() -> kotlin.Unit) {
this.multiLayerStorage = aws.sdk.kotlin.services.iotsitewise.model.MultiLayerStorage.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.RetentionPeriod] inside the given [block]
*/
public fun retentionPeriod(block: aws.sdk.kotlin.services.iotsitewise.model.RetentionPeriod.Builder.() -> kotlin.Unit) {
this.retentionPeriod = aws.sdk.kotlin.services.iotsitewise.model.RetentionPeriod.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.WarmTierRetentionPeriod] inside the given [block]
*/
public fun warmTierRetentionPeriod(block: aws.sdk.kotlin.services.iotsitewise.model.WarmTierRetentionPeriod.Builder.() -> kotlin.Unit) {
this.warmTierRetentionPeriod = aws.sdk.kotlin.services.iotsitewise.model.WarmTierRetentionPeriod.invoke(block)
}
internal fun correctErrors(): Builder {
if (storageType == null) storageType = StorageType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy