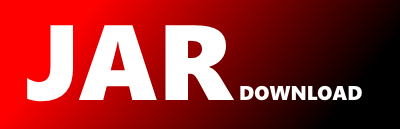
commonMain.aws.sdk.kotlin.services.iotsitewise.model.Metric.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains an asset metric property. With metrics, you can calculate aggregate functions, such as an average, maximum, or minimum, as specified through an expression. A metric maps several values to a single value (such as a sum).
*
* The maximum number of dependent/cascading variables used in any one metric calculation is 10. Therefore, a *root* metric can have up to 10 cascading metrics in its computational dependency tree. Additionally, a metric can only have a data type of `DOUBLE` and consume properties with data types of `INTEGER` or `DOUBLE`.
*
* For more information, see [Metrics](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/asset-properties.html#metrics) in the *IoT SiteWise User Guide*.
*/
public class Metric private constructor(builder: Builder) {
/**
* The mathematical expression that defines the metric aggregation function. You can specify up to 10 variables per expression. You can specify up to 10 functions per expression.
*
* For more information, see [Quotas](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/quotas.html) in the *IoT SiteWise User Guide*.
*/
public val expression: kotlin.String = requireNotNull(builder.expression) { "A non-null value must be provided for expression" }
/**
* The processing configuration for the given metric property. You can configure metrics to be computed at the edge or in the Amazon Web Services Cloud. By default, metrics are forwarded to the cloud.
*/
public val processingConfig: aws.sdk.kotlin.services.iotsitewise.model.MetricProcessingConfig? = builder.processingConfig
/**
* The list of variables used in the expression.
*/
public val variables: List = requireNotNull(builder.variables) { "A non-null value must be provided for variables" }
/**
* The window (time interval) over which IoT SiteWise computes the metric's aggregation expression. IoT SiteWise computes one data point per `window`.
*/
public val window: aws.sdk.kotlin.services.iotsitewise.model.MetricWindow? = builder.window
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.Metric = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Metric(")
append("expression=$expression,")
append("processingConfig=$processingConfig,")
append("variables=$variables,")
append("window=$window")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = expression.hashCode()
result = 31 * result + (processingConfig?.hashCode() ?: 0)
result = 31 * result + (variables.hashCode())
result = 31 * result + (window?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Metric
if (expression != other.expression) return false
if (processingConfig != other.processingConfig) return false
if (variables != other.variables) return false
if (window != other.window) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.Metric = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The mathematical expression that defines the metric aggregation function. You can specify up to 10 variables per expression. You can specify up to 10 functions per expression.
*
* For more information, see [Quotas](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/quotas.html) in the *IoT SiteWise User Guide*.
*/
public var expression: kotlin.String? = null
/**
* The processing configuration for the given metric property. You can configure metrics to be computed at the edge or in the Amazon Web Services Cloud. By default, metrics are forwarded to the cloud.
*/
public var processingConfig: aws.sdk.kotlin.services.iotsitewise.model.MetricProcessingConfig? = null
/**
* The list of variables used in the expression.
*/
public var variables: List? = null
/**
* The window (time interval) over which IoT SiteWise computes the metric's aggregation expression. IoT SiteWise computes one data point per `window`.
*/
public var window: aws.sdk.kotlin.services.iotsitewise.model.MetricWindow? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.Metric) : this() {
this.expression = x.expression
this.processingConfig = x.processingConfig
this.variables = x.variables
this.window = x.window
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.Metric = Metric(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.MetricProcessingConfig] inside the given [block]
*/
public fun processingConfig(block: aws.sdk.kotlin.services.iotsitewise.model.MetricProcessingConfig.Builder.() -> kotlin.Unit) {
this.processingConfig = aws.sdk.kotlin.services.iotsitewise.model.MetricProcessingConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.MetricWindow] inside the given [block]
*/
public fun window(block: aws.sdk.kotlin.services.iotsitewise.model.MetricWindow.Builder.() -> kotlin.Unit) {
this.window = aws.sdk.kotlin.services.iotsitewise.model.MetricWindow.invoke(block)
}
internal fun correctErrors(): Builder {
if (expression == null) expression = ""
if (variables == null) variables = emptyList()
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy