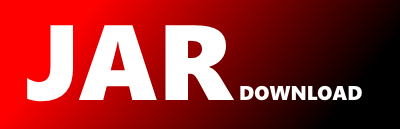
commonMain.aws.sdk.kotlin.services.iotsitewise.model.PortalSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Contains a portal summary.
*/
public class PortalSummary private constructor(builder: Builder) {
/**
* The date the portal was created, in Unix epoch time.
*/
public val creationDate: aws.smithy.kotlin.runtime.time.Instant? = builder.creationDate
/**
* The portal's description.
*/
public val description: kotlin.String? = builder.description
/**
* The ID of the portal.
*/
public val id: kotlin.String = requireNotNull(builder.id) { "A non-null value must be provided for id" }
/**
* The date the portal was last updated, in Unix epoch time.
*/
public val lastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateDate
/**
* The name of the portal.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the service role that allows the portal's users to access your IoT SiteWise resources on your behalf. For more information, see [Using service roles for IoT SiteWise Monitor](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/monitor-service-role.html) in the *IoT SiteWise User Guide*.
*/
public val roleArn: kotlin.String? = builder.roleArn
/**
* The URL for the IoT SiteWise Monitor portal. You can use this URL to access portals that use IAM Identity Center for authentication. For portals that use IAM for authentication, you must use the IoT SiteWise console to get a URL that you can use to access the portal.
*/
public val startUrl: kotlin.String = requireNotNull(builder.startUrl) { "A non-null value must be provided for startUrl" }
/**
* Contains information about the current status of a portal.
*/
public val status: aws.sdk.kotlin.services.iotsitewise.model.PortalStatus? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.PortalSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PortalSummary(")
append("creationDate=$creationDate,")
append("description=$description,")
append("id=$id,")
append("lastUpdateDate=$lastUpdateDate,")
append("name=$name,")
append("roleArn=$roleArn,")
append("startUrl=$startUrl,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = creationDate?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (id.hashCode())
result = 31 * result + (lastUpdateDate?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (startUrl.hashCode())
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PortalSummary
if (creationDate != other.creationDate) return false
if (description != other.description) return false
if (id != other.id) return false
if (lastUpdateDate != other.lastUpdateDate) return false
if (name != other.name) return false
if (roleArn != other.roleArn) return false
if (startUrl != other.startUrl) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.PortalSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The date the portal was created, in Unix epoch time.
*/
public var creationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The portal's description.
*/
public var description: kotlin.String? = null
/**
* The ID of the portal.
*/
public var id: kotlin.String? = null
/**
* The date the portal was last updated, in Unix epoch time.
*/
public var lastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the portal.
*/
public var name: kotlin.String? = null
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the service role that allows the portal's users to access your IoT SiteWise resources on your behalf. For more information, see [Using service roles for IoT SiteWise Monitor](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/monitor-service-role.html) in the *IoT SiteWise User Guide*.
*/
public var roleArn: kotlin.String? = null
/**
* The URL for the IoT SiteWise Monitor portal. You can use this URL to access portals that use IAM Identity Center for authentication. For portals that use IAM for authentication, you must use the IoT SiteWise console to get a URL that you can use to access the portal.
*/
public var startUrl: kotlin.String? = null
/**
* Contains information about the current status of a portal.
*/
public var status: aws.sdk.kotlin.services.iotsitewise.model.PortalStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.PortalSummary) : this() {
this.creationDate = x.creationDate
this.description = x.description
this.id = x.id
this.lastUpdateDate = x.lastUpdateDate
this.name = x.name
this.roleArn = x.roleArn
this.startUrl = x.startUrl
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.PortalSummary = PortalSummary(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.PortalStatus] inside the given [block]
*/
public fun status(block: aws.sdk.kotlin.services.iotsitewise.model.PortalStatus.Builder.() -> kotlin.Unit) {
this.status = aws.sdk.kotlin.services.iotsitewise.model.PortalStatus.invoke(block)
}
internal fun correctErrors(): Builder {
if (id == null) id = ""
if (name == null) name = ""
if (startUrl == null) startUrl = ""
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy