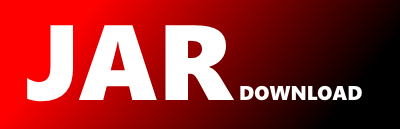
commonMain.aws.sdk.kotlin.services.iotsitewise.model.TimeSeriesSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Contains a summary of a time series (data stream).
*/
public class TimeSeriesSummary private constructor(builder: Builder) {
/**
* The alias that identifies the time series.
*/
public val alias: kotlin.String? = builder.alias
/**
* The ID of the asset in which the asset property was created.
*/
public val assetId: kotlin.String? = builder.assetId
/**
* The data type of the time series.
*
* If you specify `STRUCT`, you must also specify `dataTypeSpec` to identify the type of the structure for this time series.
*/
public val dataType: aws.sdk.kotlin.services.iotsitewise.model.PropertyDataType = requireNotNull(builder.dataType) { "A non-null value must be provided for dataType" }
/**
* The data type of the structure for this time series. This parameter is required for time series that have the `STRUCT` data type.
*
* The options for this parameter depend on the type of the composite model in which you created the asset property that is associated with your time series. Use `AWS/ALARM_STATE` for alarm state in alarm composite models.
*/
public val dataTypeSpec: kotlin.String? = builder.dataTypeSpec
/**
* The ID of the asset property, in UUID format.
*/
public val propertyId: kotlin.String? = builder.propertyId
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the time series, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:time-series/${TimeSeriesId}`
*/
public val timeSeriesArn: kotlin.String = requireNotNull(builder.timeSeriesArn) { "A non-null value must be provided for timeSeriesArn" }
/**
* The date that the time series was created, in Unix epoch time.
*/
public val timeSeriesCreationDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.timeSeriesCreationDate) { "A non-null value must be provided for timeSeriesCreationDate" }
/**
* The ID of the time series.
*/
public val timeSeriesId: kotlin.String = requireNotNull(builder.timeSeriesId) { "A non-null value must be provided for timeSeriesId" }
/**
* The date that the time series was last updated, in Unix epoch time.
*/
public val timeSeriesLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.timeSeriesLastUpdateDate) { "A non-null value must be provided for timeSeriesLastUpdateDate" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.TimeSeriesSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TimeSeriesSummary(")
append("alias=$alias,")
append("assetId=$assetId,")
append("dataType=$dataType,")
append("dataTypeSpec=$dataTypeSpec,")
append("propertyId=$propertyId,")
append("timeSeriesArn=$timeSeriesArn,")
append("timeSeriesCreationDate=$timeSeriesCreationDate,")
append("timeSeriesId=$timeSeriesId,")
append("timeSeriesLastUpdateDate=$timeSeriesLastUpdateDate")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = alias?.hashCode() ?: 0
result = 31 * result + (assetId?.hashCode() ?: 0)
result = 31 * result + (dataType.hashCode())
result = 31 * result + (dataTypeSpec?.hashCode() ?: 0)
result = 31 * result + (propertyId?.hashCode() ?: 0)
result = 31 * result + (timeSeriesArn.hashCode())
result = 31 * result + (timeSeriesCreationDate.hashCode())
result = 31 * result + (timeSeriesId.hashCode())
result = 31 * result + (timeSeriesLastUpdateDate.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TimeSeriesSummary
if (alias != other.alias) return false
if (assetId != other.assetId) return false
if (dataType != other.dataType) return false
if (dataTypeSpec != other.dataTypeSpec) return false
if (propertyId != other.propertyId) return false
if (timeSeriesArn != other.timeSeriesArn) return false
if (timeSeriesCreationDate != other.timeSeriesCreationDate) return false
if (timeSeriesId != other.timeSeriesId) return false
if (timeSeriesLastUpdateDate != other.timeSeriesLastUpdateDate) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.TimeSeriesSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The alias that identifies the time series.
*/
public var alias: kotlin.String? = null
/**
* The ID of the asset in which the asset property was created.
*/
public var assetId: kotlin.String? = null
/**
* The data type of the time series.
*
* If you specify `STRUCT`, you must also specify `dataTypeSpec` to identify the type of the structure for this time series.
*/
public var dataType: aws.sdk.kotlin.services.iotsitewise.model.PropertyDataType? = null
/**
* The data type of the structure for this time series. This parameter is required for time series that have the `STRUCT` data type.
*
* The options for this parameter depend on the type of the composite model in which you created the asset property that is associated with your time series. Use `AWS/ALARM_STATE` for alarm state in alarm composite models.
*/
public var dataTypeSpec: kotlin.String? = null
/**
* The ID of the asset property, in UUID format.
*/
public var propertyId: kotlin.String? = null
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the time series, which has the following format.
*
* `arn:${Partition}:iotsitewise:${Region}:${Account}:time-series/${TimeSeriesId}`
*/
public var timeSeriesArn: kotlin.String? = null
/**
* The date that the time series was created, in Unix epoch time.
*/
public var timeSeriesCreationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the time series.
*/
public var timeSeriesId: kotlin.String? = null
/**
* The date that the time series was last updated, in Unix epoch time.
*/
public var timeSeriesLastUpdateDate: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.TimeSeriesSummary) : this() {
this.alias = x.alias
this.assetId = x.assetId
this.dataType = x.dataType
this.dataTypeSpec = x.dataTypeSpec
this.propertyId = x.propertyId
this.timeSeriesArn = x.timeSeriesArn
this.timeSeriesCreationDate = x.timeSeriesCreationDate
this.timeSeriesId = x.timeSeriesId
this.timeSeriesLastUpdateDate = x.timeSeriesLastUpdateDate
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.TimeSeriesSummary = TimeSeriesSummary(this)
internal fun correctErrors(): Builder {
if (dataType == null) dataType = PropertyDataType.SdkUnknown("no value provided")
if (timeSeriesArn == null) timeSeriesArn = ""
if (timeSeriesCreationDate == null) timeSeriesCreationDate = Instant.fromEpochSeconds(0)
if (timeSeriesId == null) timeSeriesId = ""
if (timeSeriesLastUpdateDate == null) timeSeriesLastUpdateDate = Instant.fromEpochSeconds(0)
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy