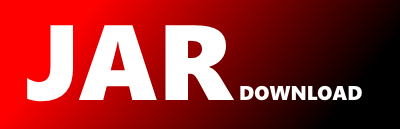
commonMain.aws.sdk.kotlin.services.iotsitewise.model.UpdateAssetModelCompositeModelRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateAssetModelCompositeModelRequest private constructor(builder: Builder) {
/**
* A description for the composite model.
*/
public val assetModelCompositeModelDescription: kotlin.String? = builder.assetModelCompositeModelDescription
/**
* An external ID to assign to the asset model. You can only set the external ID of the asset model if it wasn't set when it was created, or you're setting it to the exact same thing as when it was created.
*/
public val assetModelCompositeModelExternalId: kotlin.String? = builder.assetModelCompositeModelExternalId
/**
* The ID of a composite model on this asset model.
*/
public val assetModelCompositeModelId: kotlin.String? = builder.assetModelCompositeModelId
/**
* A unique name for the composite model.
*/
public val assetModelCompositeModelName: kotlin.String? = builder.assetModelCompositeModelName
/**
* The property definitions of the composite model. For more information, see [ Inline custom composite models](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/custom-composite-models.html#inline-composite-models) in the *IoT SiteWise User Guide*.
*
* You can specify up to 200 properties per composite model. For more information, see [Quotas](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/quotas.html) in the *IoT SiteWise User Guide*.
*/
public val assetModelCompositeModelProperties: List? = builder.assetModelCompositeModelProperties
/**
* The ID of the asset model, in UUID format.
*/
public val assetModelId: kotlin.String? = builder.assetModelId
/**
* A unique case-sensitive identifier that you can provide to ensure the idempotency of the request. Don't reuse this client token if a new idempotent request is required.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* The expected current entity tag (ETag) for the asset model’s latest or active version (specified using `matchForVersionType`). The update request is rejected if the tag does not match the latest or active version's current entity tag. See [Optimistic locking for asset model writes](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/opt-locking-for-model.html) in the *IoT SiteWise User Guide*.
*/
public val ifMatch: kotlin.String? = builder.ifMatch
/**
* Accepts ***** to reject the update request if an active version (specified using `matchForVersionType` as `ACTIVE`) already exists for the asset model.
*/
public val ifNoneMatch: kotlin.String? = builder.ifNoneMatch
/**
* Specifies the asset model version type (`LATEST` or `ACTIVE`) used in conjunction with `If-Match` or `If-None-Match` headers to determine the target ETag for the update operation.
*/
public val matchForVersionType: aws.sdk.kotlin.services.iotsitewise.model.AssetModelVersionType? = builder.matchForVersionType
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.UpdateAssetModelCompositeModelRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateAssetModelCompositeModelRequest(")
append("assetModelCompositeModelDescription=$assetModelCompositeModelDescription,")
append("assetModelCompositeModelExternalId=$assetModelCompositeModelExternalId,")
append("assetModelCompositeModelId=$assetModelCompositeModelId,")
append("assetModelCompositeModelName=$assetModelCompositeModelName,")
append("assetModelCompositeModelProperties=$assetModelCompositeModelProperties,")
append("assetModelId=$assetModelId,")
append("clientToken=$clientToken,")
append("ifMatch=$ifMatch,")
append("ifNoneMatch=$ifNoneMatch,")
append("matchForVersionType=$matchForVersionType")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = assetModelCompositeModelDescription?.hashCode() ?: 0
result = 31 * result + (assetModelCompositeModelExternalId?.hashCode() ?: 0)
result = 31 * result + (assetModelCompositeModelId?.hashCode() ?: 0)
result = 31 * result + (assetModelCompositeModelName?.hashCode() ?: 0)
result = 31 * result + (assetModelCompositeModelProperties?.hashCode() ?: 0)
result = 31 * result + (assetModelId?.hashCode() ?: 0)
result = 31 * result + (clientToken?.hashCode() ?: 0)
result = 31 * result + (ifMatch?.hashCode() ?: 0)
result = 31 * result + (ifNoneMatch?.hashCode() ?: 0)
result = 31 * result + (matchForVersionType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateAssetModelCompositeModelRequest
if (assetModelCompositeModelDescription != other.assetModelCompositeModelDescription) return false
if (assetModelCompositeModelExternalId != other.assetModelCompositeModelExternalId) return false
if (assetModelCompositeModelId != other.assetModelCompositeModelId) return false
if (assetModelCompositeModelName != other.assetModelCompositeModelName) return false
if (assetModelCompositeModelProperties != other.assetModelCompositeModelProperties) return false
if (assetModelId != other.assetModelId) return false
if (clientToken != other.clientToken) return false
if (ifMatch != other.ifMatch) return false
if (ifNoneMatch != other.ifNoneMatch) return false
if (matchForVersionType != other.matchForVersionType) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.UpdateAssetModelCompositeModelRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A description for the composite model.
*/
public var assetModelCompositeModelDescription: kotlin.String? = null
/**
* An external ID to assign to the asset model. You can only set the external ID of the asset model if it wasn't set when it was created, or you're setting it to the exact same thing as when it was created.
*/
public var assetModelCompositeModelExternalId: kotlin.String? = null
/**
* The ID of a composite model on this asset model.
*/
public var assetModelCompositeModelId: kotlin.String? = null
/**
* A unique name for the composite model.
*/
public var assetModelCompositeModelName: kotlin.String? = null
/**
* The property definitions of the composite model. For more information, see [ Inline custom composite models](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/custom-composite-models.html#inline-composite-models) in the *IoT SiteWise User Guide*.
*
* You can specify up to 200 properties per composite model. For more information, see [Quotas](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/quotas.html) in the *IoT SiteWise User Guide*.
*/
public var assetModelCompositeModelProperties: List? = null
/**
* The ID of the asset model, in UUID format.
*/
public var assetModelId: kotlin.String? = null
/**
* A unique case-sensitive identifier that you can provide to ensure the idempotency of the request. Don't reuse this client token if a new idempotent request is required.
*/
public var clientToken: kotlin.String? = null
/**
* The expected current entity tag (ETag) for the asset model’s latest or active version (specified using `matchForVersionType`). The update request is rejected if the tag does not match the latest or active version's current entity tag. See [Optimistic locking for asset model writes](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/opt-locking-for-model.html) in the *IoT SiteWise User Guide*.
*/
public var ifMatch: kotlin.String? = null
/**
* Accepts ***** to reject the update request if an active version (specified using `matchForVersionType` as `ACTIVE`) already exists for the asset model.
*/
public var ifNoneMatch: kotlin.String? = null
/**
* Specifies the asset model version type (`LATEST` or `ACTIVE`) used in conjunction with `If-Match` or `If-None-Match` headers to determine the target ETag for the update operation.
*/
public var matchForVersionType: aws.sdk.kotlin.services.iotsitewise.model.AssetModelVersionType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.UpdateAssetModelCompositeModelRequest) : this() {
this.assetModelCompositeModelDescription = x.assetModelCompositeModelDescription
this.assetModelCompositeModelExternalId = x.assetModelCompositeModelExternalId
this.assetModelCompositeModelId = x.assetModelCompositeModelId
this.assetModelCompositeModelName = x.assetModelCompositeModelName
this.assetModelCompositeModelProperties = x.assetModelCompositeModelProperties
this.assetModelId = x.assetModelId
this.clientToken = x.clientToken
this.ifMatch = x.ifMatch
this.ifNoneMatch = x.ifNoneMatch
this.matchForVersionType = x.matchForVersionType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.UpdateAssetModelCompositeModelRequest = UpdateAssetModelCompositeModelRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy