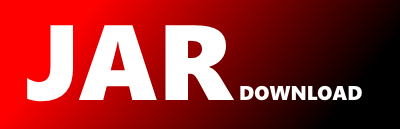
commonMain.aws.sdk.kotlin.services.iotsitewise.model.UpdatePortalRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdatePortalRequest private constructor(builder: Builder) {
/**
* Contains the configuration information of an alarm created in an IoT SiteWise Monitor portal. You can use the alarm to monitor an asset property and get notified when the asset property value is outside a specified range. For more information, see [Monitoring with alarms](https://docs.aws.amazon.com/iot-sitewise/latest/appguide/monitor-alarms.html) in the *IoT SiteWise Application Guide*.
*/
public val alarms: aws.sdk.kotlin.services.iotsitewise.model.Alarms? = builder.alarms
/**
* A unique case-sensitive identifier that you can provide to ensure the idempotency of the request. Don't reuse this client token if a new idempotent request is required.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* The email address that sends alarm notifications.
*/
public val notificationSenderEmail: kotlin.String? = builder.notificationSenderEmail
/**
* The Amazon Web Services administrator's contact email address.
*/
public val portalContactEmail: kotlin.String? = builder.portalContactEmail
/**
* A new description for the portal.
*/
public val portalDescription: kotlin.String? = builder.portalDescription
/**
* The ID of the portal to update.
*/
public val portalId: kotlin.String? = builder.portalId
/**
* Contains an image that is one of the following:
* + An image file. Choose this option to upload a new image.
* + The ID of an existing image. Choose this option to keep an existing image.
*/
public val portalLogoImage: aws.sdk.kotlin.services.iotsitewise.model.Image? = builder.portalLogoImage
/**
* A new friendly name for the portal.
*/
public val portalName: kotlin.String? = builder.portalName
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of a service role that allows the portal's users to access your IoT SiteWise resources on your behalf. For more information, see [Using service roles for IoT SiteWise Monitor](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/monitor-service-role.html) in the *IoT SiteWise User Guide*.
*/
public val roleArn: kotlin.String? = builder.roleArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.UpdatePortalRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdatePortalRequest(")
append("alarms=$alarms,")
append("clientToken=$clientToken,")
append("notificationSenderEmail=$notificationSenderEmail,")
append("portalContactEmail=$portalContactEmail,")
append("portalDescription=$portalDescription,")
append("portalId=$portalId,")
append("portalLogoImage=$portalLogoImage,")
append("portalName=$portalName,")
append("roleArn=$roleArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = alarms?.hashCode() ?: 0
result = 31 * result + (clientToken?.hashCode() ?: 0)
result = 31 * result + (notificationSenderEmail?.hashCode() ?: 0)
result = 31 * result + (portalContactEmail?.hashCode() ?: 0)
result = 31 * result + (portalDescription?.hashCode() ?: 0)
result = 31 * result + (portalId?.hashCode() ?: 0)
result = 31 * result + (portalLogoImage?.hashCode() ?: 0)
result = 31 * result + (portalName?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdatePortalRequest
if (alarms != other.alarms) return false
if (clientToken != other.clientToken) return false
if (notificationSenderEmail != other.notificationSenderEmail) return false
if (portalContactEmail != other.portalContactEmail) return false
if (portalDescription != other.portalDescription) return false
if (portalId != other.portalId) return false
if (portalLogoImage != other.portalLogoImage) return false
if (portalName != other.portalName) return false
if (roleArn != other.roleArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.UpdatePortalRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Contains the configuration information of an alarm created in an IoT SiteWise Monitor portal. You can use the alarm to monitor an asset property and get notified when the asset property value is outside a specified range. For more information, see [Monitoring with alarms](https://docs.aws.amazon.com/iot-sitewise/latest/appguide/monitor-alarms.html) in the *IoT SiteWise Application Guide*.
*/
public var alarms: aws.sdk.kotlin.services.iotsitewise.model.Alarms? = null
/**
* A unique case-sensitive identifier that you can provide to ensure the idempotency of the request. Don't reuse this client token if a new idempotent request is required.
*/
public var clientToken: kotlin.String? = null
/**
* The email address that sends alarm notifications.
*/
public var notificationSenderEmail: kotlin.String? = null
/**
* The Amazon Web Services administrator's contact email address.
*/
public var portalContactEmail: kotlin.String? = null
/**
* A new description for the portal.
*/
public var portalDescription: kotlin.String? = null
/**
* The ID of the portal to update.
*/
public var portalId: kotlin.String? = null
/**
* Contains an image that is one of the following:
* + An image file. Choose this option to upload a new image.
* + The ID of an existing image. Choose this option to keep an existing image.
*/
public var portalLogoImage: aws.sdk.kotlin.services.iotsitewise.model.Image? = null
/**
* A new friendly name for the portal.
*/
public var portalName: kotlin.String? = null
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of a service role that allows the portal's users to access your IoT SiteWise resources on your behalf. For more information, see [Using service roles for IoT SiteWise Monitor](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/monitor-service-role.html) in the *IoT SiteWise User Guide*.
*/
public var roleArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.UpdatePortalRequest) : this() {
this.alarms = x.alarms
this.clientToken = x.clientToken
this.notificationSenderEmail = x.notificationSenderEmail
this.portalContactEmail = x.portalContactEmail
this.portalDescription = x.portalDescription
this.portalId = x.portalId
this.portalLogoImage = x.portalLogoImage
this.portalName = x.portalName
this.roleArn = x.roleArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.UpdatePortalRequest = UpdatePortalRequest(this)
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.Alarms] inside the given [block]
*/
public fun alarms(block: aws.sdk.kotlin.services.iotsitewise.model.Alarms.Builder.() -> kotlin.Unit) {
this.alarms = aws.sdk.kotlin.services.iotsitewise.model.Alarms.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.iotsitewise.model.Image] inside the given [block]
*/
public fun portalLogoImage(block: aws.sdk.kotlin.services.iotsitewise.model.Image.Builder.() -> kotlin.Unit) {
this.portalLogoImage = aws.sdk.kotlin.services.iotsitewise.model.Image.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy