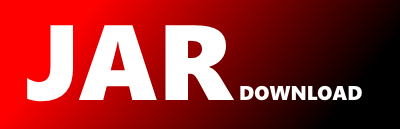
commonMain.aws.sdk.kotlin.services.iotsitewise.model.VariableValue.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotsitewise-jvm Show documentation
Show all versions of iotsitewise-jvm Show documentation
The AWS SDK for Kotlin client for IoTSiteWise
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotsitewise.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Identifies a property value used in an expression.
*/
public class VariableValue private constructor(builder: Builder) {
/**
* The ID of the hierarchy to query for the property ID. You can use the hierarchy's name instead of the hierarchy's ID. If the hierarchy has an external ID, you can specify `externalId:` followed by the external ID. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*
* You use a hierarchy ID instead of a model ID because you can have several hierarchies using the same model and therefore the same `propertyId`. For example, you might have separately grouped assets that come from the same asset model. For more information, see [Asset hierarchies](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/asset-hierarchies.html) in the *IoT SiteWise User Guide*.
*/
public val hierarchyId: kotlin.String? = builder.hierarchyId
/**
* The ID of the property to use as the variable. You can use the property `name` if it's from the same asset model. If the property has an external ID, you can specify `externalId:` followed by the external ID. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public val propertyId: kotlin.String? = builder.propertyId
/**
* The path of the property.
*/
public val propertyPath: List? = builder.propertyPath
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotsitewise.model.VariableValue = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("VariableValue(")
append("hierarchyId=$hierarchyId,")
append("propertyId=$propertyId,")
append("propertyPath=$propertyPath")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = hierarchyId?.hashCode() ?: 0
result = 31 * result + (propertyId?.hashCode() ?: 0)
result = 31 * result + (propertyPath?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as VariableValue
if (hierarchyId != other.hierarchyId) return false
if (propertyId != other.propertyId) return false
if (propertyPath != other.propertyPath) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotsitewise.model.VariableValue = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ID of the hierarchy to query for the property ID. You can use the hierarchy's name instead of the hierarchy's ID. If the hierarchy has an external ID, you can specify `externalId:` followed by the external ID. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*
* You use a hierarchy ID instead of a model ID because you can have several hierarchies using the same model and therefore the same `propertyId`. For example, you might have separately grouped assets that come from the same asset model. For more information, see [Asset hierarchies](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/asset-hierarchies.html) in the *IoT SiteWise User Guide*.
*/
public var hierarchyId: kotlin.String? = null
/**
* The ID of the property to use as the variable. You can use the property `name` if it's from the same asset model. If the property has an external ID, you can specify `externalId:` followed by the external ID. For more information, see [Using external IDs](https://docs.aws.amazon.com/iot-sitewise/latest/userguide/object-ids.html#external-ids) in the *IoT SiteWise User Guide*.
*/
public var propertyId: kotlin.String? = null
/**
* The path of the property.
*/
public var propertyPath: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotsitewise.model.VariableValue) : this() {
this.hierarchyId = x.hierarchyId
this.propertyId = x.propertyId
this.propertyPath = x.propertyPath
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotsitewise.model.VariableValue = VariableValue(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy