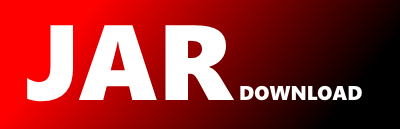
commonMain.aws.sdk.kotlin.services.iotwireless.model.MetricName.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotwireless-jvm Show documentation
Show all versions of iotwireless-jvm Show documentation
The AWS Kotlin client for IoT Wireless
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotwireless.model
import kotlin.collections.List
public sealed class MetricName {
public abstract val value: kotlin.String
public object AwsAccountActiveDeviceCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountActiveDeviceCount"
override fun toString(): kotlin.String = "AwsAccountActiveDeviceCount"
}
public object AwsAccountActiveGatewayCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountActiveGatewayCount"
override fun toString(): kotlin.String = "AwsAccountActiveGatewayCount"
}
public object AwsAccountDeviceCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountDeviceCount"
override fun toString(): kotlin.String = "AwsAccountDeviceCount"
}
public object AwsAccountDownlinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountDownlinkCount"
override fun toString(): kotlin.String = "AwsAccountDownlinkCount"
}
public object AwsAccountGatewayCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountGatewayCount"
override fun toString(): kotlin.String = "AwsAccountGatewayCount"
}
public object AwsAccountJoinAcceptCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountJoinAcceptCount"
override fun toString(): kotlin.String = "AwsAccountJoinAcceptCount"
}
public object AwsAccountJoinRequestCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountJoinRequestCount"
override fun toString(): kotlin.String = "AwsAccountJoinRequestCount"
}
public object AwsAccountRoamingDownlinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountRoamingDownlinkCount"
override fun toString(): kotlin.String = "AwsAccountRoamingDownlinkCount"
}
public object AwsAccountRoamingUplinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountRoamingUplinkCount"
override fun toString(): kotlin.String = "AwsAccountRoamingUplinkCount"
}
public object AwsAccountUplinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountUplinkCount"
override fun toString(): kotlin.String = "AwsAccountUplinkCount"
}
public object AwsAccountUplinkLostCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountUplinkLostCount"
override fun toString(): kotlin.String = "AwsAccountUplinkLostCount"
}
public object AwsAccountUplinkLostRate : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "AwsAccountUplinkLostRate"
override fun toString(): kotlin.String = "AwsAccountUplinkLostRate"
}
public object DeviceDownlinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceDownlinkCount"
override fun toString(): kotlin.String = "DeviceDownlinkCount"
}
public object DeviceJoinAcceptCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceJoinAcceptCount"
override fun toString(): kotlin.String = "DeviceJoinAcceptCount"
}
public object DeviceJoinRequestCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceJoinRequestCount"
override fun toString(): kotlin.String = "DeviceJoinRequestCount"
}
public object DeviceRssi : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceRSSI"
override fun toString(): kotlin.String = "DeviceRssi"
}
public object DeviceRoamingDownlinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceRoamingDownlinkCount"
override fun toString(): kotlin.String = "DeviceRoamingDownlinkCount"
}
public object DeviceRoamingUplinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceRoamingUplinkCount"
override fun toString(): kotlin.String = "DeviceRoamingUplinkCount"
}
public object DeviceSnr : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceSNR"
override fun toString(): kotlin.String = "DeviceSnr"
}
public object DeviceUplinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceUplinkCount"
override fun toString(): kotlin.String = "DeviceUplinkCount"
}
public object DeviceUplinkLostCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceUplinkLostCount"
override fun toString(): kotlin.String = "DeviceUplinkLostCount"
}
public object DeviceUplinkLostRate : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "DeviceUplinkLostRate"
override fun toString(): kotlin.String = "DeviceUplinkLostRate"
}
public object GatewayDownTime : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "GatewayDownTime"
override fun toString(): kotlin.String = "GatewayDownTime"
}
public object GatewayDownlinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "GatewayDownlinkCount"
override fun toString(): kotlin.String = "GatewayDownlinkCount"
}
public object GatewayJoinAcceptCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "GatewayJoinAcceptCount"
override fun toString(): kotlin.String = "GatewayJoinAcceptCount"
}
public object GatewayJoinRequestCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "GatewayJoinRequestCount"
override fun toString(): kotlin.String = "GatewayJoinRequestCount"
}
public object GatewayRssi : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "GatewayRSSI"
override fun toString(): kotlin.String = "GatewayRssi"
}
public object GatewaySnr : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "GatewaySNR"
override fun toString(): kotlin.String = "GatewaySnr"
}
public object GatewayUpTime : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "GatewayUpTime"
override fun toString(): kotlin.String = "GatewayUpTime"
}
public object GatewayUplinkCount : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override val value: kotlin.String = "GatewayUplinkCount"
override fun toString(): kotlin.String = "GatewayUplinkCount"
}
public data class SdkUnknown(override val value: kotlin.String) : aws.sdk.kotlin.services.iotwireless.model.MetricName() {
override fun toString(): kotlin.String = "SdkUnknown($value)"
}
public companion object {
/**
* Convert a raw value to one of the sealed variants or [SdkUnknown]
*/
public fun fromValue(value: kotlin.String): aws.sdk.kotlin.services.iotwireless.model.MetricName = when (value) {
"AwsAccountActiveDeviceCount" -> AwsAccountActiveDeviceCount
"AwsAccountActiveGatewayCount" -> AwsAccountActiveGatewayCount
"AwsAccountDeviceCount" -> AwsAccountDeviceCount
"AwsAccountDownlinkCount" -> AwsAccountDownlinkCount
"AwsAccountGatewayCount" -> AwsAccountGatewayCount
"AwsAccountJoinAcceptCount" -> AwsAccountJoinAcceptCount
"AwsAccountJoinRequestCount" -> AwsAccountJoinRequestCount
"AwsAccountRoamingDownlinkCount" -> AwsAccountRoamingDownlinkCount
"AwsAccountRoamingUplinkCount" -> AwsAccountRoamingUplinkCount
"AwsAccountUplinkCount" -> AwsAccountUplinkCount
"AwsAccountUplinkLostCount" -> AwsAccountUplinkLostCount
"AwsAccountUplinkLostRate" -> AwsAccountUplinkLostRate
"DeviceDownlinkCount" -> DeviceDownlinkCount
"DeviceJoinAcceptCount" -> DeviceJoinAcceptCount
"DeviceJoinRequestCount" -> DeviceJoinRequestCount
"DeviceRSSI" -> DeviceRssi
"DeviceRoamingDownlinkCount" -> DeviceRoamingDownlinkCount
"DeviceRoamingUplinkCount" -> DeviceRoamingUplinkCount
"DeviceSNR" -> DeviceSnr
"DeviceUplinkCount" -> DeviceUplinkCount
"DeviceUplinkLostCount" -> DeviceUplinkLostCount
"DeviceUplinkLostRate" -> DeviceUplinkLostRate
"GatewayDownTime" -> GatewayDownTime
"GatewayDownlinkCount" -> GatewayDownlinkCount
"GatewayJoinAcceptCount" -> GatewayJoinAcceptCount
"GatewayJoinRequestCount" -> GatewayJoinRequestCount
"GatewayRSSI" -> GatewayRssi
"GatewaySNR" -> GatewaySnr
"GatewayUpTime" -> GatewayUpTime
"GatewayUplinkCount" -> GatewayUplinkCount
else -> SdkUnknown(value)
}
/**
* Get a list of all possible variants
*/
public fun values(): kotlin.collections.List = values
private val values: kotlin.collections.List = listOf(
AwsAccountActiveDeviceCount,
AwsAccountActiveGatewayCount,
AwsAccountDeviceCount,
AwsAccountDownlinkCount,
AwsAccountGatewayCount,
AwsAccountJoinAcceptCount,
AwsAccountJoinRequestCount,
AwsAccountRoamingDownlinkCount,
AwsAccountRoamingUplinkCount,
AwsAccountUplinkCount,
AwsAccountUplinkLostCount,
AwsAccountUplinkLostRate,
DeviceDownlinkCount,
DeviceJoinAcceptCount,
DeviceJoinRequestCount,
DeviceRssi,
DeviceRoamingDownlinkCount,
DeviceRoamingUplinkCount,
DeviceSnr,
DeviceUplinkCount,
DeviceUplinkLostCount,
DeviceUplinkLostRate,
GatewayDownTime,
GatewayDownlinkCount,
GatewayJoinAcceptCount,
GatewayJoinRequestCount,
GatewayRssi,
GatewaySnr,
GatewayUpTime,
GatewayUplinkCount,
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy