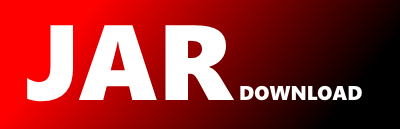
commonMain.aws.sdk.kotlin.services.iotwireless.model.GsmNmrObj.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotwireless-jvm Show documentation
Show all versions of iotwireless-jvm Show documentation
The AWS Kotlin client for IoT Wireless
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotwireless.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* GSM object for network measurement reports.
*/
public class GsmNmrObj private constructor(builder: Builder) {
/**
* GSM broadcast control channel.
*/
public val bcch: kotlin.Int = requireNotNull(builder.bcch) { "A non-null value must be provided for bcch" }
/**
* GSM base station identity code (BSIC).
*/
public val bsic: kotlin.Int = requireNotNull(builder.bsic) { "A non-null value must be provided for bsic" }
/**
* Global identity information of the GSM object.
*/
public val globalIdentity: aws.sdk.kotlin.services.iotwireless.model.GlobalIdentity? = builder.globalIdentity
/**
* Rx level, which is the received signal power, measured in dBm (decibel-milliwatts).
*/
public val rxLevel: kotlin.Int? = builder.rxLevel
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotwireless.model.GsmNmrObj = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GsmNmrObj(")
append("bcch=$bcch,")
append("bsic=$bsic,")
append("globalIdentity=$globalIdentity,")
append("rxLevel=$rxLevel")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = bcch
result = 31 * result + (bsic)
result = 31 * result + (globalIdentity?.hashCode() ?: 0)
result = 31 * result + (rxLevel ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GsmNmrObj
if (bcch != other.bcch) return false
if (bsic != other.bsic) return false
if (globalIdentity != other.globalIdentity) return false
if (rxLevel != other.rxLevel) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotwireless.model.GsmNmrObj = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* GSM broadcast control channel.
*/
public var bcch: kotlin.Int? = null
/**
* GSM base station identity code (BSIC).
*/
public var bsic: kotlin.Int? = null
/**
* Global identity information of the GSM object.
*/
public var globalIdentity: aws.sdk.kotlin.services.iotwireless.model.GlobalIdentity? = null
/**
* Rx level, which is the received signal power, measured in dBm (decibel-milliwatts).
*/
public var rxLevel: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotwireless.model.GsmNmrObj) : this() {
this.bcch = x.bcch
this.bsic = x.bsic
this.globalIdentity = x.globalIdentity
this.rxLevel = x.rxLevel
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotwireless.model.GsmNmrObj = GsmNmrObj(this)
/**
* construct an [aws.sdk.kotlin.services.iotwireless.model.GlobalIdentity] inside the given [block]
*/
public fun globalIdentity(block: aws.sdk.kotlin.services.iotwireless.model.GlobalIdentity.Builder.() -> kotlin.Unit) {
this.globalIdentity = aws.sdk.kotlin.services.iotwireless.model.GlobalIdentity.invoke(block)
}
internal fun correctErrors(): Builder {
if (bcch == null) bcch = 0
if (bsic == null) bsic = 0
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy