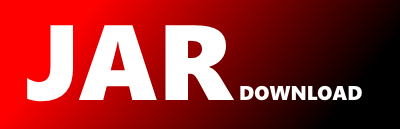
commonMain.aws.sdk.kotlin.services.iotwireless.model.LoRaWanGateway.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotwireless-jvm Show documentation
Show all versions of iotwireless-jvm Show documentation
The AWS Kotlin client for IoT Wireless
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotwireless.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* LoRaWANGateway object.
*/
public class LoRaWanGateway private constructor(builder: Builder) {
/**
* Beaconing object information, which consists of the data rate and frequency parameters.
*/
public val beaconing: aws.sdk.kotlin.services.iotwireless.model.Beaconing? = builder.beaconing
/**
* The gateway's EUI value.
*/
public val gatewayEui: kotlin.String? = builder.gatewayEui
/**
* A list of JoinEuiRange used by LoRa gateways to filter LoRa frames.
*/
public val joinEuiFilters: List>? = builder.joinEuiFilters
/**
* The MaxEIRP value.
*/
public val maxEirp: kotlin.Float? = builder.maxEirp
/**
* A list of NetId values that are used by LoRa gateways to filter the uplink frames.
*/
public val netIdFilters: List? = builder.netIdFilters
/**
* The frequency band (RFRegion) value.
*/
public val rfRegion: kotlin.String? = builder.rfRegion
/**
* A list of integer indicating which sub bands are supported by LoRa gateway.
*/
public val subBands: List? = builder.subBands
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotwireless.model.LoRaWanGateway = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("LoRaWanGateway(")
append("beaconing=$beaconing,")
append("gatewayEui=$gatewayEui,")
append("joinEuiFilters=$joinEuiFilters,")
append("maxEirp=$maxEirp,")
append("netIdFilters=$netIdFilters,")
append("rfRegion=$rfRegion,")
append("subBands=$subBands")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = beaconing?.hashCode() ?: 0
result = 31 * result + (gatewayEui?.hashCode() ?: 0)
result = 31 * result + (joinEuiFilters?.hashCode() ?: 0)
result = 31 * result + (maxEirp?.hashCode() ?: 0)
result = 31 * result + (netIdFilters?.hashCode() ?: 0)
result = 31 * result + (rfRegion?.hashCode() ?: 0)
result = 31 * result + (subBands?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as LoRaWanGateway
if (beaconing != other.beaconing) return false
if (gatewayEui != other.gatewayEui) return false
if (joinEuiFilters != other.joinEuiFilters) return false
if (!(maxEirp?.equals(other.maxEirp) ?: (other.maxEirp == null))) return false
if (netIdFilters != other.netIdFilters) return false
if (rfRegion != other.rfRegion) return false
if (subBands != other.subBands) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotwireless.model.LoRaWanGateway = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Beaconing object information, which consists of the data rate and frequency parameters.
*/
public var beaconing: aws.sdk.kotlin.services.iotwireless.model.Beaconing? = null
/**
* The gateway's EUI value.
*/
public var gatewayEui: kotlin.String? = null
/**
* A list of JoinEuiRange used by LoRa gateways to filter LoRa frames.
*/
public var joinEuiFilters: List>? = null
/**
* The MaxEIRP value.
*/
public var maxEirp: kotlin.Float? = null
/**
* A list of NetId values that are used by LoRa gateways to filter the uplink frames.
*/
public var netIdFilters: List? = null
/**
* The frequency band (RFRegion) value.
*/
public var rfRegion: kotlin.String? = null
/**
* A list of integer indicating which sub bands are supported by LoRa gateway.
*/
public var subBands: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotwireless.model.LoRaWanGateway) : this() {
this.beaconing = x.beaconing
this.gatewayEui = x.gatewayEui
this.joinEuiFilters = x.joinEuiFilters
this.maxEirp = x.maxEirp
this.netIdFilters = x.netIdFilters
this.rfRegion = x.rfRegion
this.subBands = x.subBands
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotwireless.model.LoRaWanGateway = LoRaWanGateway(this)
/**
* construct an [aws.sdk.kotlin.services.iotwireless.model.Beaconing] inside the given [block]
*/
public fun beaconing(block: aws.sdk.kotlin.services.iotwireless.model.Beaconing.Builder.() -> kotlin.Unit) {
this.beaconing = aws.sdk.kotlin.services.iotwireless.model.Beaconing.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy