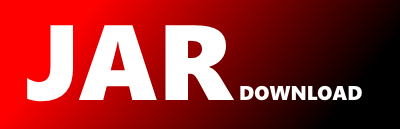
commonMain.aws.sdk.kotlin.services.iotwireless.model.UpdateNetworkAnalyzerConfigurationRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotwireless-jvm Show documentation
Show all versions of iotwireless-jvm Show documentation
The AWS Kotlin client for IoT Wireless
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.iotwireless.model
import aws.smithy.kotlin.runtime.SdkDsl
public class UpdateNetworkAnalyzerConfigurationRequest private constructor(builder: Builder) {
/**
* Name of the network analyzer configuration.
*/
public val configurationName: kotlin.String? = builder.configurationName
/**
* The description of the new resource.
*/
public val description: kotlin.String? = builder.description
/**
* Multicast group resources to add to the network analyzer configuration. Provide the `MulticastGroupId` of the resource to add in the input array.
*/
public val multicastGroupsToAdd: List? = builder.multicastGroupsToAdd
/**
* Multicast group resources to remove from the network analyzer configuration. Provide the `MulticastGroupId` of the resources to remove in the input array.
*/
public val multicastGroupsToRemove: List? = builder.multicastGroupsToRemove
/**
* Trace content for your wireless devices, gateways, and multicast groups.
*/
public val traceContent: aws.sdk.kotlin.services.iotwireless.model.TraceContent? = builder.traceContent
/**
* Wireless device resources to add to the network analyzer configuration. Provide the `WirelessDeviceId` of the resource to add in the input array.
*/
public val wirelessDevicesToAdd: List? = builder.wirelessDevicesToAdd
/**
* Wireless device resources to remove from the network analyzer configuration. Provide the `WirelessDeviceId` of the resources to remove in the input array.
*/
public val wirelessDevicesToRemove: List? = builder.wirelessDevicesToRemove
/**
* Wireless gateway resources to add to the network analyzer configuration. Provide the `WirelessGatewayId` of the resource to add in the input array.
*/
public val wirelessGatewaysToAdd: List? = builder.wirelessGatewaysToAdd
/**
* Wireless gateway resources to remove from the network analyzer configuration. Provide the `WirelessGatewayId` of the resources to remove in the input array.
*/
public val wirelessGatewaysToRemove: List? = builder.wirelessGatewaysToRemove
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.iotwireless.model.UpdateNetworkAnalyzerConfigurationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateNetworkAnalyzerConfigurationRequest(")
append("configurationName=$configurationName,")
append("description=$description,")
append("multicastGroupsToAdd=$multicastGroupsToAdd,")
append("multicastGroupsToRemove=$multicastGroupsToRemove,")
append("traceContent=$traceContent,")
append("wirelessDevicesToAdd=$wirelessDevicesToAdd,")
append("wirelessDevicesToRemove=$wirelessDevicesToRemove,")
append("wirelessGatewaysToAdd=$wirelessGatewaysToAdd,")
append("wirelessGatewaysToRemove=$wirelessGatewaysToRemove")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = configurationName?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (multicastGroupsToAdd?.hashCode() ?: 0)
result = 31 * result + (multicastGroupsToRemove?.hashCode() ?: 0)
result = 31 * result + (traceContent?.hashCode() ?: 0)
result = 31 * result + (wirelessDevicesToAdd?.hashCode() ?: 0)
result = 31 * result + (wirelessDevicesToRemove?.hashCode() ?: 0)
result = 31 * result + (wirelessGatewaysToAdd?.hashCode() ?: 0)
result = 31 * result + (wirelessGatewaysToRemove?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateNetworkAnalyzerConfigurationRequest
if (configurationName != other.configurationName) return false
if (description != other.description) return false
if (multicastGroupsToAdd != other.multicastGroupsToAdd) return false
if (multicastGroupsToRemove != other.multicastGroupsToRemove) return false
if (traceContent != other.traceContent) return false
if (wirelessDevicesToAdd != other.wirelessDevicesToAdd) return false
if (wirelessDevicesToRemove != other.wirelessDevicesToRemove) return false
if (wirelessGatewaysToAdd != other.wirelessGatewaysToAdd) return false
if (wirelessGatewaysToRemove != other.wirelessGatewaysToRemove) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.iotwireless.model.UpdateNetworkAnalyzerConfigurationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Name of the network analyzer configuration.
*/
public var configurationName: kotlin.String? = null
/**
* The description of the new resource.
*/
public var description: kotlin.String? = null
/**
* Multicast group resources to add to the network analyzer configuration. Provide the `MulticastGroupId` of the resource to add in the input array.
*/
public var multicastGroupsToAdd: List? = null
/**
* Multicast group resources to remove from the network analyzer configuration. Provide the `MulticastGroupId` of the resources to remove in the input array.
*/
public var multicastGroupsToRemove: List? = null
/**
* Trace content for your wireless devices, gateways, and multicast groups.
*/
public var traceContent: aws.sdk.kotlin.services.iotwireless.model.TraceContent? = null
/**
* Wireless device resources to add to the network analyzer configuration. Provide the `WirelessDeviceId` of the resource to add in the input array.
*/
public var wirelessDevicesToAdd: List? = null
/**
* Wireless device resources to remove from the network analyzer configuration. Provide the `WirelessDeviceId` of the resources to remove in the input array.
*/
public var wirelessDevicesToRemove: List? = null
/**
* Wireless gateway resources to add to the network analyzer configuration. Provide the `WirelessGatewayId` of the resource to add in the input array.
*/
public var wirelessGatewaysToAdd: List? = null
/**
* Wireless gateway resources to remove from the network analyzer configuration. Provide the `WirelessGatewayId` of the resources to remove in the input array.
*/
public var wirelessGatewaysToRemove: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.iotwireless.model.UpdateNetworkAnalyzerConfigurationRequest) : this() {
this.configurationName = x.configurationName
this.description = x.description
this.multicastGroupsToAdd = x.multicastGroupsToAdd
this.multicastGroupsToRemove = x.multicastGroupsToRemove
this.traceContent = x.traceContent
this.wirelessDevicesToAdd = x.wirelessDevicesToAdd
this.wirelessDevicesToRemove = x.wirelessDevicesToRemove
this.wirelessGatewaysToAdd = x.wirelessGatewaysToAdd
this.wirelessGatewaysToRemove = x.wirelessGatewaysToRemove
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.iotwireless.model.UpdateNetworkAnalyzerConfigurationRequest = UpdateNetworkAnalyzerConfigurationRequest(this)
/**
* construct an [aws.sdk.kotlin.services.iotwireless.model.TraceContent] inside the given [block]
*/
public fun traceContent(block: aws.sdk.kotlin.services.iotwireless.model.TraceContent.Builder.() -> kotlin.Unit) {
this.traceContent = aws.sdk.kotlin.services.iotwireless.model.TraceContent.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy