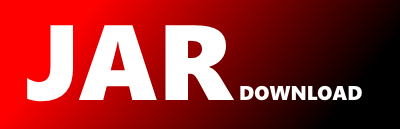
commonMain.aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexmodelbuildingservice.model
/**
* Settings used to configure delivery mode and destination for conversation logs.
*/
public class LogSettingsRequest private constructor(builder: Builder) {
/**
* Where the logs will be delivered. Text logs are delivered to a CloudWatch Logs log group. Audio logs are delivered to an S3 bucket.
*/
public val destination: aws.sdk.kotlin.services.lexmodelbuildingservice.model.Destination = requireNotNull(builder.destination) { "A non-null value must be provided for destination" }
/**
* The Amazon Resource Name (ARN) of the AWS KMS customer managed key for encrypting audio logs delivered to an S3 bucket. The key does not apply to CloudWatch Logs and is optional for S3 buckets.
*/
public val kmsKeyArn: kotlin.String? = builder.kmsKeyArn
/**
* The type of logging to enable. Text logs are delivered to a CloudWatch Logs log group. Audio logs are delivered to an S3 bucket.
*/
public val logType: aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogType = requireNotNull(builder.logType) { "A non-null value must be provided for logType" }
/**
* The Amazon Resource Name (ARN) of the CloudWatch Logs log group or S3 bucket where the logs should be delivered.
*/
public val resourceArn: kotlin.String = requireNotNull(builder.resourceArn) { "A non-null value must be provided for resourceArn" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("LogSettingsRequest(")
append("destination=$destination,")
append("kmsKeyArn=$kmsKeyArn,")
append("logType=$logType,")
append("resourceArn=$resourceArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = destination.hashCode()
result = 31 * result + (kmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (logType.hashCode())
result = 31 * result + (resourceArn.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as LogSettingsRequest
if (destination != other.destination) return false
if (kmsKeyArn != other.kmsKeyArn) return false
if (logType != other.logType) return false
if (resourceArn != other.resourceArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsRequest = Builder(this).apply(block).build()
public class Builder {
/**
* Where the logs will be delivered. Text logs are delivered to a CloudWatch Logs log group. Audio logs are delivered to an S3 bucket.
*/
public var destination: aws.sdk.kotlin.services.lexmodelbuildingservice.model.Destination? = null
/**
* The Amazon Resource Name (ARN) of the AWS KMS customer managed key for encrypting audio logs delivered to an S3 bucket. The key does not apply to CloudWatch Logs and is optional for S3 buckets.
*/
public var kmsKeyArn: kotlin.String? = null
/**
* The type of logging to enable. Text logs are delivered to a CloudWatch Logs log group. Audio logs are delivered to an S3 bucket.
*/
public var logType: aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogType? = null
/**
* The Amazon Resource Name (ARN) of the CloudWatch Logs log group or S3 bucket where the logs should be delivered.
*/
public var resourceArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsRequest) : this() {
this.destination = x.destination
this.kmsKeyArn = x.kmsKeyArn
this.logType = x.logType
this.resourceArn = x.resourceArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsRequest = LogSettingsRequest(this)
internal fun correctErrors(): Builder {
if (destination == null) destination = Destination.SdkUnknown("no value provided")
if (logType == null) logType = LogType.SdkUnknown("no value provided")
if (resourceArn == null) resourceArn = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy