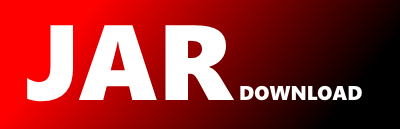
commonMain.aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationSummary.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexmodelbuildingservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides information about migrating a bot from Amazon Lex V1 to Amazon Lex V2.
*/
public class MigrationSummary private constructor(builder: Builder) {
/**
* The unique identifier that Amazon Lex assigned to the migration.
*/
public val migrationId: kotlin.String? = builder.migrationId
/**
* The status of the operation. When the status is `COMPLETE` the bot is available in Amazon Lex V2. There may be alerts and warnings that need to be resolved to complete the migration.
*/
public val migrationStatus: aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationStatus? = builder.migrationStatus
/**
* The strategy used to conduct the migration.
*/
public val migrationStrategy: aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationStrategy? = builder.migrationStrategy
/**
* The date and time that the migration started.
*/
public val migrationTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.migrationTimestamp
/**
* The locale of the Amazon Lex V1 bot that is the source of the migration.
*/
public val v1BotLocale: aws.sdk.kotlin.services.lexmodelbuildingservice.model.Locale? = builder.v1BotLocale
/**
* The name of the Amazon Lex V1 bot that is the source of the migration.
*/
public val v1BotName: kotlin.String? = builder.v1BotName
/**
* The version of the Amazon Lex V1 bot that is the source of the migration.
*/
public val v1BotVersion: kotlin.String? = builder.v1BotVersion
/**
* The unique identifier of the Amazon Lex V2 that is the destination of the migration.
*/
public val v2BotId: kotlin.String? = builder.v2BotId
/**
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*/
public val v2BotRole: kotlin.String? = builder.v2BotRole
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MigrationSummary(")
append("migrationId=$migrationId,")
append("migrationStatus=$migrationStatus,")
append("migrationStrategy=$migrationStrategy,")
append("migrationTimestamp=$migrationTimestamp,")
append("v1BotLocale=$v1BotLocale,")
append("v1BotName=$v1BotName,")
append("v1BotVersion=$v1BotVersion,")
append("v2BotId=$v2BotId,")
append("v2BotRole=$v2BotRole")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = migrationId?.hashCode() ?: 0
result = 31 * result + (migrationStatus?.hashCode() ?: 0)
result = 31 * result + (migrationStrategy?.hashCode() ?: 0)
result = 31 * result + (migrationTimestamp?.hashCode() ?: 0)
result = 31 * result + (v1BotLocale?.hashCode() ?: 0)
result = 31 * result + (v1BotName?.hashCode() ?: 0)
result = 31 * result + (v1BotVersion?.hashCode() ?: 0)
result = 31 * result + (v2BotId?.hashCode() ?: 0)
result = 31 * result + (v2BotRole?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MigrationSummary
if (migrationId != other.migrationId) return false
if (migrationStatus != other.migrationStatus) return false
if (migrationStrategy != other.migrationStrategy) return false
if (migrationTimestamp != other.migrationTimestamp) return false
if (v1BotLocale != other.v1BotLocale) return false
if (v1BotName != other.v1BotName) return false
if (v1BotVersion != other.v1BotVersion) return false
if (v2BotId != other.v2BotId) return false
if (v2BotRole != other.v2BotRole) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationSummary = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The unique identifier that Amazon Lex assigned to the migration.
*/
public var migrationId: kotlin.String? = null
/**
* The status of the operation. When the status is `COMPLETE` the bot is available in Amazon Lex V2. There may be alerts and warnings that need to be resolved to complete the migration.
*/
public var migrationStatus: aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationStatus? = null
/**
* The strategy used to conduct the migration.
*/
public var migrationStrategy: aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationStrategy? = null
/**
* The date and time that the migration started.
*/
public var migrationTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The locale of the Amazon Lex V1 bot that is the source of the migration.
*/
public var v1BotLocale: aws.sdk.kotlin.services.lexmodelbuildingservice.model.Locale? = null
/**
* The name of the Amazon Lex V1 bot that is the source of the migration.
*/
public var v1BotName: kotlin.String? = null
/**
* The version of the Amazon Lex V1 bot that is the source of the migration.
*/
public var v1BotVersion: kotlin.String? = null
/**
* The unique identifier of the Amazon Lex V2 that is the destination of the migration.
*/
public var v2BotId: kotlin.String? = null
/**
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*/
public var v2BotRole: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationSummary) : this() {
this.migrationId = x.migrationId
this.migrationStatus = x.migrationStatus
this.migrationStrategy = x.migrationStrategy
this.migrationTimestamp = x.migrationTimestamp
this.v1BotLocale = x.v1BotLocale
this.v1BotName = x.v1BotName
this.v1BotVersion = x.v1BotVersion
this.v2BotId = x.v2BotId
this.v2BotRole = x.v2BotRole
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationSummary = MigrationSummary(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy