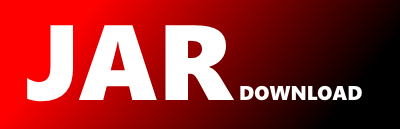
commonMain.aws.sdk.kotlin.services.lexmodelbuildingservice.model.StartMigrationRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexmodelbuildingservice.model
import aws.smithy.kotlin.runtime.SdkDsl
public class StartMigrationRequest private constructor(builder: Builder) {
/**
* The strategy used to conduct the migration.
* + `CREATE_NEW` - Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
* + `UPDATE_EXISTING` - Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated. It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is created in the Amazon Lex V2 bot.
*/
public val migrationStrategy: aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationStrategy? = builder.migrationStrategy
/**
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*/
public val v1BotName: kotlin.String? = builder.v1BotName
/**
* The version of the bot to migrate to Amazon Lex V2. You can migrate the `$LATEST` version as well as any numbered version.
*/
public val v1BotVersion: kotlin.String? = builder.v1BotVersion
/**
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
* + If the Amazon Lex V2 bot doesn't exist, you must use the `CREATE_NEW` migration strategy.
* + If the Amazon Lex V2 bot exists, you must use the `UPDATE_EXISTING` migration strategy to change the contents of the Amazon Lex V2 bot.
*/
public val v2BotName: kotlin.String? = builder.v2BotName
/**
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*/
public val v2BotRole: kotlin.String? = builder.v2BotRole
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexmodelbuildingservice.model.StartMigrationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StartMigrationRequest(")
append("migrationStrategy=$migrationStrategy,")
append("v1BotName=$v1BotName,")
append("v1BotVersion=$v1BotVersion,")
append("v2BotName=$v2BotName,")
append("v2BotRole=$v2BotRole")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = migrationStrategy?.hashCode() ?: 0
result = 31 * result + (v1BotName?.hashCode() ?: 0)
result = 31 * result + (v1BotVersion?.hashCode() ?: 0)
result = 31 * result + (v2BotName?.hashCode() ?: 0)
result = 31 * result + (v2BotRole?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StartMigrationRequest
if (migrationStrategy != other.migrationStrategy) return false
if (v1BotName != other.v1BotName) return false
if (v1BotVersion != other.v1BotVersion) return false
if (v2BotName != other.v2BotName) return false
if (v2BotRole != other.v2BotRole) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexmodelbuildingservice.model.StartMigrationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The strategy used to conduct the migration.
* + `CREATE_NEW` - Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
* + `UPDATE_EXISTING` - Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated. It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is created in the Amazon Lex V2 bot.
*/
public var migrationStrategy: aws.sdk.kotlin.services.lexmodelbuildingservice.model.MigrationStrategy? = null
/**
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*/
public var v1BotName: kotlin.String? = null
/**
* The version of the bot to migrate to Amazon Lex V2. You can migrate the `$LATEST` version as well as any numbered version.
*/
public var v1BotVersion: kotlin.String? = null
/**
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
* + If the Amazon Lex V2 bot doesn't exist, you must use the `CREATE_NEW` migration strategy.
* + If the Amazon Lex V2 bot exists, you must use the `UPDATE_EXISTING` migration strategy to change the contents of the Amazon Lex V2 bot.
*/
public var v2BotName: kotlin.String? = null
/**
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*/
public var v2BotRole: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexmodelbuildingservice.model.StartMigrationRequest) : this() {
this.migrationStrategy = x.migrationStrategy
this.v1BotName = x.v1BotName
this.v1BotVersion = x.v1BotVersion
this.v2BotName = x.v2BotName
this.v2BotRole = x.v2BotRole
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexmodelbuildingservice.model.StartMigrationRequest = StartMigrationRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy