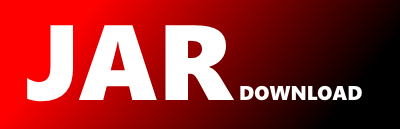
commonMain.aws.sdk.kotlin.services.lexmodelbuildingservice.model.GetBotChannelAssociationsRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexmodelbuildingservice.model
import aws.smithy.kotlin.runtime.SdkDsl
public class GetBotChannelAssociationsRequest private constructor(builder: Builder) {
/**
* An alias pointing to the specific version of the Amazon Lex bot to which this association is being made.
*/
public val botAlias: kotlin.String? = builder.botAlias
/**
* The name of the Amazon Lex bot in the association.
*/
public val botName: kotlin.String? = builder.botName
/**
* The maximum number of associations to return in the response. The default is 50.
*/
public val maxResults: kotlin.Int? = builder.maxResults
/**
* Substring to match in channel association names. An association will be returned if any part of its name matches the substring. For example, "xyz" matches both "xyzabc" and "abcxyz." To return all bot channel associations, use a hyphen ("-") as the `nameContains` parameter.
*/
public val nameContains: kotlin.String? = builder.nameContains
/**
* A pagination token for fetching the next page of associations. If the response to this call is truncated, Amazon Lex returns a pagination token in the response. To fetch the next page of associations, specify the pagination token in the next request.
*/
public val nextToken: kotlin.String? = builder.nextToken
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexmodelbuildingservice.model.GetBotChannelAssociationsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetBotChannelAssociationsRequest(")
append("botAlias=$botAlias,")
append("botName=$botName,")
append("maxResults=$maxResults,")
append("nameContains=$nameContains,")
append("nextToken=$nextToken")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = botAlias?.hashCode() ?: 0
result = 31 * result + (botName?.hashCode() ?: 0)
result = 31 * result + (maxResults ?: 0)
result = 31 * result + (nameContains?.hashCode() ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetBotChannelAssociationsRequest
if (botAlias != other.botAlias) return false
if (botName != other.botName) return false
if (maxResults != other.maxResults) return false
if (nameContains != other.nameContains) return false
if (nextToken != other.nextToken) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexmodelbuildingservice.model.GetBotChannelAssociationsRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* An alias pointing to the specific version of the Amazon Lex bot to which this association is being made.
*/
public var botAlias: kotlin.String? = null
/**
* The name of the Amazon Lex bot in the association.
*/
public var botName: kotlin.String? = null
/**
* The maximum number of associations to return in the response. The default is 50.
*/
public var maxResults: kotlin.Int? = null
/**
* Substring to match in channel association names. An association will be returned if any part of its name matches the substring. For example, "xyz" matches both "xyzabc" and "abcxyz." To return all bot channel associations, use a hyphen ("-") as the `nameContains` parameter.
*/
public var nameContains: kotlin.String? = null
/**
* A pagination token for fetching the next page of associations. If the response to this call is truncated, Amazon Lex returns a pagination token in the response. To fetch the next page of associations, specify the pagination token in the next request.
*/
public var nextToken: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexmodelbuildingservice.model.GetBotChannelAssociationsRequest) : this() {
this.botAlias = x.botAlias
this.botName = x.botName
this.maxResults = x.maxResults
this.nameContains = x.nameContains
this.nextToken = x.nextToken
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexmodelbuildingservice.model.GetBotChannelAssociationsRequest = GetBotChannelAssociationsRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy