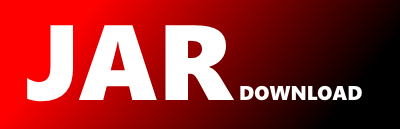
commonMain.aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsResponse.kt Maven / Gradle / Ivy
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexmodelbuildingservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The settings for conversation logs.
*/
public class LogSettingsResponse private constructor(builder: Builder) {
/**
* The destination where logs are delivered.
*/
public val destination: aws.sdk.kotlin.services.lexmodelbuildingservice.model.Destination? = builder.destination
/**
* The Amazon Resource Name (ARN) of the key used to encrypt audio logs in an S3 bucket.
*/
public val kmsKeyArn: kotlin.String? = builder.kmsKeyArn
/**
* The type of logging that is enabled.
*/
public val logType: aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogType? = builder.logType
/**
* The Amazon Resource Name (ARN) of the CloudWatch Logs log group or S3 bucket where the logs are delivered.
*/
public val resourceArn: kotlin.String? = builder.resourceArn
/**
* The resource prefix is the first part of the S3 object key within the S3 bucket that you specified to contain audio logs. For CloudWatch Logs it is the prefix of the log stream name within the log group that you specified.
*/
public val resourcePrefix: kotlin.String? = builder.resourcePrefix
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("LogSettingsResponse(")
append("destination=$destination,")
append("kmsKeyArn=$kmsKeyArn,")
append("logType=$logType,")
append("resourceArn=$resourceArn,")
append("resourcePrefix=$resourcePrefix")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = destination?.hashCode() ?: 0
result = 31 * result + (kmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (logType?.hashCode() ?: 0)
result = 31 * result + (resourceArn?.hashCode() ?: 0)
result = 31 * result + (resourcePrefix?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as LogSettingsResponse
if (destination != other.destination) return false
if (kmsKeyArn != other.kmsKeyArn) return false
if (logType != other.logType) return false
if (resourceArn != other.resourceArn) return false
if (resourcePrefix != other.resourcePrefix) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The destination where logs are delivered.
*/
public var destination: aws.sdk.kotlin.services.lexmodelbuildingservice.model.Destination? = null
/**
* The Amazon Resource Name (ARN) of the key used to encrypt audio logs in an S3 bucket.
*/
public var kmsKeyArn: kotlin.String? = null
/**
* The type of logging that is enabled.
*/
public var logType: aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogType? = null
/**
* The Amazon Resource Name (ARN) of the CloudWatch Logs log group or S3 bucket where the logs are delivered.
*/
public var resourceArn: kotlin.String? = null
/**
* The resource prefix is the first part of the S3 object key within the S3 bucket that you specified to contain audio logs. For CloudWatch Logs it is the prefix of the log stream name within the log group that you specified.
*/
public var resourcePrefix: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsResponse) : this() {
this.destination = x.destination
this.kmsKeyArn = x.kmsKeyArn
this.logType = x.logType
this.resourceArn = x.resourceArn
this.resourcePrefix = x.resourcePrefix
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexmodelbuildingservice.model.LogSettingsResponse = LogSettingsResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy