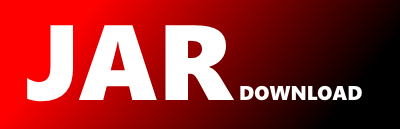
commonMain.aws.sdk.kotlin.services.lexmodelbuildingservice.model.Prompt.kt Maven / Gradle / Ivy
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexmodelbuildingservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Obtains information from the user. To define a prompt, provide one or more messages and specify the number of attempts to get information from the user. If you provide more than one message, Amazon Lex chooses one of the messages to use to prompt the user. For more information, see how-it-works.
*/
public class Prompt private constructor(builder: Builder) {
/**
* The number of times to prompt the user for information.
*/
public val maxAttempts: kotlin.Int = requireNotNull(builder.maxAttempts) { "A non-null value must be provided for maxAttempts" }
/**
* An array of objects, each of which provides a message string and its type. You can specify the message string in plain text or in Speech Synthesis Markup Language (SSML).
*/
public val messages: List = requireNotNull(builder.messages) { "A non-null value must be provided for messages" }
/**
* A response card. Amazon Lex uses this prompt at runtime, in the `PostText` API response. It substitutes session attributes and slot values for placeholders in the response card. For more information, see ex-resp-card.
*/
public val responseCard: kotlin.String? = builder.responseCard
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexmodelbuildingservice.model.Prompt = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Prompt(")
append("maxAttempts=$maxAttempts,")
append("messages=$messages,")
append("responseCard=$responseCard")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = maxAttempts
result = 31 * result + (messages.hashCode())
result = 31 * result + (responseCard?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Prompt
if (maxAttempts != other.maxAttempts) return false
if (messages != other.messages) return false
if (responseCard != other.responseCard) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexmodelbuildingservice.model.Prompt = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The number of times to prompt the user for information.
*/
public var maxAttempts: kotlin.Int? = null
/**
* An array of objects, each of which provides a message string and its type. You can specify the message string in plain text or in Speech Synthesis Markup Language (SSML).
*/
public var messages: List? = null
/**
* A response card. Amazon Lex uses this prompt at runtime, in the `PostText` API response. It substitutes session attributes and slot values for placeholders in the response card. For more information, see ex-resp-card.
*/
public var responseCard: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexmodelbuildingservice.model.Prompt) : this() {
this.maxAttempts = x.maxAttempts
this.messages = x.messages
this.responseCard = x.responseCard
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexmodelbuildingservice.model.Prompt = Prompt(this)
internal fun correctErrors(): Builder {
if (maxAttempts == null) maxAttempts = 0
if (messages == null) messages = emptyList()
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy