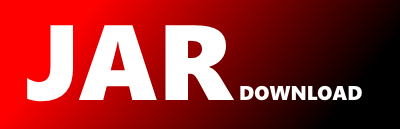
commonMain.aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lexruntimeservice-jvm Show documentation
Show all versions of lexruntimeservice-jvm Show documentation
The AWS Kotlin client for Lex Runtime Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexruntimeservice.model
/**
* Describes the next action that the bot should take in its interaction with the user and provides information about the context in which the action takes place. Use the `DialogAction` data type to set the interaction to a specific state, or to return the interaction to a previous state.
*/
public class DialogAction private constructor(builder: Builder) {
/**
* The fulfillment state of the intent. The possible values are:
* + `Failed` - The Lambda function associated with the intent failed to fulfill the intent.
* + `Fulfilled` - The intent has fulfilled by the Lambda function associated with the intent.
* + `ReadyForFulfillment` - All of the information necessary for the intent is present and the intent ready to be fulfilled by the client application.
*/
public val fulfillmentState: aws.sdk.kotlin.services.lexruntimeservice.model.FulfillmentState? = builder.fulfillmentState
/**
* The name of the intent.
*/
public val intentName: kotlin.String? = builder.intentName
/**
* The message that should be shown to the user. If you don't specify a message, Amazon Lex will use the message configured for the intent.
*/
public val message: kotlin.String? = builder.message
/**
* + `PlainText` - The message contains plain UTF-8 text.
* + `CustomPayload` - The message is a custom format for the client.
* + `SSML` - The message contains text formatted for voice output.
* + `Composite` - The message contains an escaped JSON object containing one or more messages. For more information, see [Message Groups](https://docs.aws.amazon.com/lex/latest/dg/howitworks-manage-prompts.html).
*/
public val messageFormat: aws.sdk.kotlin.services.lexruntimeservice.model.MessageFormatType? = builder.messageFormat
/**
* The name of the slot that should be elicited from the user.
*/
public val slotToElicit: kotlin.String? = builder.slotToElicit
/**
* Map of the slots that have been gathered and their values.
*/
public val slots: Map? = builder.slots
/**
* The next action that the bot should take in its interaction with the user. The possible values are:
* + `ConfirmIntent` - The next action is asking the user if the intent is complete and ready to be fulfilled. This is a yes/no question such as "Place the order?"
* + `Close` - Indicates that the there will not be a response from the user. For example, the statement "Your order has been placed" does not require a response.
* + `Delegate` - The next action is determined by Amazon Lex.
* + `ElicitIntent` - The next action is to determine the intent that the user wants to fulfill.
* + `ElicitSlot` - The next action is to elicit a slot value from the user.
*/
public val type: aws.sdk.kotlin.services.lexruntimeservice.model.DialogActionType = requireNotNull(builder.type) { "A non-null value must be provided for type" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DialogAction(")
append("fulfillmentState=$fulfillmentState,")
append("intentName=$intentName,")
append("message=*** Sensitive Data Redacted ***,")
append("messageFormat=$messageFormat,")
append("slotToElicit=$slotToElicit,")
append("slots=*** Sensitive Data Redacted ***,")
append("type=$type")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = fulfillmentState?.hashCode() ?: 0
result = 31 * result + (intentName?.hashCode() ?: 0)
result = 31 * result + (message?.hashCode() ?: 0)
result = 31 * result + (messageFormat?.hashCode() ?: 0)
result = 31 * result + (slotToElicit?.hashCode() ?: 0)
result = 31 * result + (slots?.hashCode() ?: 0)
result = 31 * result + (type.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DialogAction
if (fulfillmentState != other.fulfillmentState) return false
if (intentName != other.intentName) return false
if (message != other.message) return false
if (messageFormat != other.messageFormat) return false
if (slotToElicit != other.slotToElicit) return false
if (slots != other.slots) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction = Builder(this).apply(block).build()
public class Builder {
/**
* The fulfillment state of the intent. The possible values are:
* + `Failed` - The Lambda function associated with the intent failed to fulfill the intent.
* + `Fulfilled` - The intent has fulfilled by the Lambda function associated with the intent.
* + `ReadyForFulfillment` - All of the information necessary for the intent is present and the intent ready to be fulfilled by the client application.
*/
public var fulfillmentState: aws.sdk.kotlin.services.lexruntimeservice.model.FulfillmentState? = null
/**
* The name of the intent.
*/
public var intentName: kotlin.String? = null
/**
* The message that should be shown to the user. If you don't specify a message, Amazon Lex will use the message configured for the intent.
*/
public var message: kotlin.String? = null
/**
* + `PlainText` - The message contains plain UTF-8 text.
* + `CustomPayload` - The message is a custom format for the client.
* + `SSML` - The message contains text formatted for voice output.
* + `Composite` - The message contains an escaped JSON object containing one or more messages. For more information, see [Message Groups](https://docs.aws.amazon.com/lex/latest/dg/howitworks-manage-prompts.html).
*/
public var messageFormat: aws.sdk.kotlin.services.lexruntimeservice.model.MessageFormatType? = null
/**
* The name of the slot that should be elicited from the user.
*/
public var slotToElicit: kotlin.String? = null
/**
* Map of the slots that have been gathered and their values.
*/
public var slots: Map? = null
/**
* The next action that the bot should take in its interaction with the user. The possible values are:
* + `ConfirmIntent` - The next action is asking the user if the intent is complete and ready to be fulfilled. This is a yes/no question such as "Place the order?"
* + `Close` - Indicates that the there will not be a response from the user. For example, the statement "Your order has been placed" does not require a response.
* + `Delegate` - The next action is determined by Amazon Lex.
* + `ElicitIntent` - The next action is to determine the intent that the user wants to fulfill.
* + `ElicitSlot` - The next action is to elicit a slot value from the user.
*/
public var type: aws.sdk.kotlin.services.lexruntimeservice.model.DialogActionType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction) : this() {
this.fulfillmentState = x.fulfillmentState
this.intentName = x.intentName
this.message = x.message
this.messageFormat = x.messageFormat
this.slotToElicit = x.slotToElicit
this.slots = x.slots
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction = DialogAction(this)
internal fun correctErrors(): Builder {
if (type == null) type = DialogActionType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy