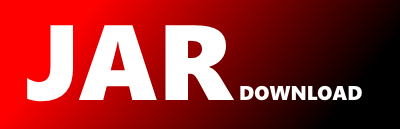
commonMain.aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexruntimeservice.model
public class PutSessionRequest private constructor(builder: Builder) {
/**
* The message that Amazon Lex returns in the response can be either text or speech based depending on the value of this field.
* + If the value is `text/plain; charset=utf-8`, Amazon Lex returns text in the response.
* + If the value begins with `audio/`, Amazon Lex returns speech in the response. Amazon Lex uses Amazon Polly to generate the speech in the configuration that you specify. For example, if you specify `audio/mpeg` as the value, Amazon Lex returns speech in the MPEG format.
* + If the value is `audio/pcm`, the speech is returned as `audio/pcm` in 16-bit, little endian format.
* + The following are the accepted values:
* + `audio/mpeg`
* + `audio/ogg`
* + `audio/pcm`
* + `audio/*` (defaults to mpeg)
* + `text/plain; charset=utf-8`
*/
public val accept: kotlin.String? = builder.accept
/**
* A list of contexts active for the request. A context can be activated when a previous intent is fulfilled, or by including the context in the request,
*
* If you don't specify a list of contexts, Amazon Lex will use the current list of contexts for the session. If you specify an empty list, all contexts for the session are cleared.
*/
public val activeContexts: List? = builder.activeContexts
/**
* The alias in use for the bot that contains the session data.
*/
public val botAlias: kotlin.String? = builder.botAlias
/**
* The name of the bot that contains the session data.
*/
public val botName: kotlin.String? = builder.botName
/**
* Sets the next action that the bot should take to fulfill the conversation.
*/
public val dialogAction: aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction? = builder.dialogAction
/**
* A summary of the recent intents for the bot. You can use the intent summary view to set a checkpoint label on an intent and modify attributes of intents. You can also use it to remove or add intent summary objects to the list.
*
* An intent that you modify or add to the list must make sense for the bot. For example, the intent name must be valid for the bot. You must provide valid values for:
* + `intentName`
* + slot names
* + `slotToElict`
*
* If you send the `recentIntentSummaryView` parameter in a `PutSession` request, the contents of the new summary view replaces the old summary view. For example, if a `GetSession` request returns three intents in the summary view and you call `PutSession` with one intent in the summary view, the next call to `GetSession` will only return one intent.
*/
public val recentIntentSummaryView: List? = builder.recentIntentSummaryView
/**
* Map of key/value pairs representing the session-specific context information. It contains application information passed between Amazon Lex and a client application.
*/
public val sessionAttributes: Map? = builder.sessionAttributes
/**
* The ID of the client application user. Amazon Lex uses this to identify a user's conversation with your bot.
*/
public val userId: kotlin.String? = builder.userId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PutSessionRequest(")
append("accept=$accept,")
append("activeContexts=*** Sensitive Data Redacted ***,")
append("botAlias=$botAlias,")
append("botName=$botName,")
append("dialogAction=$dialogAction,")
append("recentIntentSummaryView=$recentIntentSummaryView,")
append("sessionAttributes=*** Sensitive Data Redacted ***,")
append("userId=$userId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accept?.hashCode() ?: 0
result = 31 * result + (activeContexts?.hashCode() ?: 0)
result = 31 * result + (botAlias?.hashCode() ?: 0)
result = 31 * result + (botName?.hashCode() ?: 0)
result = 31 * result + (dialogAction?.hashCode() ?: 0)
result = 31 * result + (recentIntentSummaryView?.hashCode() ?: 0)
result = 31 * result + (sessionAttributes?.hashCode() ?: 0)
result = 31 * result + (userId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PutSessionRequest
if (accept != other.accept) return false
if (activeContexts != other.activeContexts) return false
if (botAlias != other.botAlias) return false
if (botName != other.botName) return false
if (dialogAction != other.dialogAction) return false
if (recentIntentSummaryView != other.recentIntentSummaryView) return false
if (sessionAttributes != other.sessionAttributes) return false
if (userId != other.userId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionRequest = Builder(this).apply(block).build()
public class Builder {
/**
* The message that Amazon Lex returns in the response can be either text or speech based depending on the value of this field.
* + If the value is `text/plain; charset=utf-8`, Amazon Lex returns text in the response.
* + If the value begins with `audio/`, Amazon Lex returns speech in the response. Amazon Lex uses Amazon Polly to generate the speech in the configuration that you specify. For example, if you specify `audio/mpeg` as the value, Amazon Lex returns speech in the MPEG format.
* + If the value is `audio/pcm`, the speech is returned as `audio/pcm` in 16-bit, little endian format.
* + The following are the accepted values:
* + `audio/mpeg`
* + `audio/ogg`
* + `audio/pcm`
* + `audio/*` (defaults to mpeg)
* + `text/plain; charset=utf-8`
*/
public var accept: kotlin.String? = null
/**
* A list of contexts active for the request. A context can be activated when a previous intent is fulfilled, or by including the context in the request,
*
* If you don't specify a list of contexts, Amazon Lex will use the current list of contexts for the session. If you specify an empty list, all contexts for the session are cleared.
*/
public var activeContexts: List? = null
/**
* The alias in use for the bot that contains the session data.
*/
public var botAlias: kotlin.String? = null
/**
* The name of the bot that contains the session data.
*/
public var botName: kotlin.String? = null
/**
* Sets the next action that the bot should take to fulfill the conversation.
*/
public var dialogAction: aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction? = null
/**
* A summary of the recent intents for the bot. You can use the intent summary view to set a checkpoint label on an intent and modify attributes of intents. You can also use it to remove or add intent summary objects to the list.
*
* An intent that you modify or add to the list must make sense for the bot. For example, the intent name must be valid for the bot. You must provide valid values for:
* + `intentName`
* + slot names
* + `slotToElict`
*
* If you send the `recentIntentSummaryView` parameter in a `PutSession` request, the contents of the new summary view replaces the old summary view. For example, if a `GetSession` request returns three intents in the summary view and you call `PutSession` with one intent in the summary view, the next call to `GetSession` will only return one intent.
*/
public var recentIntentSummaryView: List? = null
/**
* Map of key/value pairs representing the session-specific context information. It contains application information passed between Amazon Lex and a client application.
*/
public var sessionAttributes: Map? = null
/**
* The ID of the client application user. Amazon Lex uses this to identify a user's conversation with your bot.
*/
public var userId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionRequest) : this() {
this.accept = x.accept
this.activeContexts = x.activeContexts
this.botAlias = x.botAlias
this.botName = x.botName
this.dialogAction = x.dialogAction
this.recentIntentSummaryView = x.recentIntentSummaryView
this.sessionAttributes = x.sessionAttributes
this.userId = x.userId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionRequest = PutSessionRequest(this)
/**
* construct an [aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction] inside the given [block]
*/
public fun dialogAction(block: aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction.Builder.() -> kotlin.Unit) {
this.dialogAction = aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy