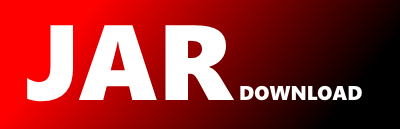
commonMain.aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lexruntimeservice-jvm Show documentation
Show all versions of lexruntimeservice-jvm Show documentation
The AWS Kotlin client for Lex Runtime Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexruntimeservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.content.ByteStream
public class PutSessionResponse private constructor(builder: Builder) {
/**
* A list of active contexts for the session.
*/
public val activeContexts: kotlin.String? = builder.activeContexts
/**
* The audio version of the message to convey to the user.
*/
public val audioStream: aws.smithy.kotlin.runtime.content.ByteStream? = builder.audioStream
/**
* Content type as specified in the `Accept` HTTP header in the request.
*/
public val contentType: kotlin.String? = builder.contentType
/**
* + `ConfirmIntent` - Amazon Lex is expecting a "yes" or "no" response to confirm the intent before fulfilling an intent.
* + `ElicitIntent` - Amazon Lex wants to elicit the user's intent.
* + `ElicitSlot` - Amazon Lex is expecting the value of a slot for the current intent.
* + `Failed` - Conveys that the conversation with the user has failed. This can happen for various reasons, including the user does not provide an appropriate response to prompts from the service, or if the Lambda function fails to fulfill the intent.
* + `Fulfilled` - Conveys that the Lambda function has sucessfully fulfilled the intent.
* + `ReadyForFulfillment` - Conveys that the client has to fulfill the intent.
*/
public val dialogState: aws.sdk.kotlin.services.lexruntimeservice.model.DialogState? = builder.dialogState
/**
* The next message that should be presented to the user.
*
* The `encodedMessage` field is base-64 encoded. You must decode the field before you can use the value.
*/
public val encodedMessage: kotlin.String? = builder.encodedMessage
/**
* The name of the current intent.
*/
public val intentName: kotlin.String? = builder.intentName
/**
* The next message that should be presented to the user.
*
* You can only use this field in the de-DE, en-AU, en-GB, en-US, es-419, es-ES, es-US, fr-CA, fr-FR, and it-IT locales. In all other locales, the `message` field is null. You should use the `encodedMessage` field instead.
*/
@Deprecated("The message field is deprecated, use the encodedMessage field instead. The message field is available only in the de-DE, en-AU, en-GB, en-US, es-419, es-ES, es-US, fr-CA, fr-FR and it-IT locales.")
public val message: kotlin.String? = builder.message
/**
* The format of the response message. One of the following values:
* + `PlainText` - The message contains plain UTF-8 text.
* + `CustomPayload` - The message is a custom format for the client.
* + `SSML` - The message contains text formatted for voice output.
* + `Composite` - The message contains an escaped JSON object containing one or more messages from the groups that messages were assigned to when the intent was created.
*/
public val messageFormat: aws.sdk.kotlin.services.lexruntimeservice.model.MessageFormatType? = builder.messageFormat
/**
* Map of key/value pairs representing session-specific context information.
*/
public val sessionAttributes: kotlin.String? = builder.sessionAttributes
/**
* A unique identifier for the session.
*/
public val sessionId: kotlin.String? = builder.sessionId
/**
* If the `dialogState` is `ElicitSlot`, returns the name of the slot for which Amazon Lex is eliciting a value.
*/
public val slotToElicit: kotlin.String? = builder.slotToElicit
/**
* Map of zero or more intent slots Amazon Lex detected from the user input during the conversation.
*
* Amazon Lex creates a resolution list containing likely values for a slot. The value that it returns is determined by the `valueSelectionStrategy` selected when the slot type was created or updated. If `valueSelectionStrategy` is set to `ORIGINAL_VALUE`, the value provided by the user is returned, if the user value is similar to the slot values. If `valueSelectionStrategy` is set to `TOP_RESOLUTION` Amazon Lex returns the first value in the resolution list or, if there is no resolution list, null. If you don't specify a `valueSelectionStrategy` the default is `ORIGINAL_VALUE`.
*/
public val slots: kotlin.String? = builder.slots
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PutSessionResponse(")
append("activeContexts=*** Sensitive Data Redacted ***,")
append("audioStream=$audioStream,")
append("contentType=$contentType,")
append("dialogState=$dialogState,")
append("encodedMessage=*** Sensitive Data Redacted ***,")
append("intentName=$intentName,")
append("message=*** Sensitive Data Redacted ***,")
append("messageFormat=$messageFormat,")
append("sessionAttributes=$sessionAttributes,")
append("sessionId=$sessionId,")
append("slotToElicit=$slotToElicit,")
append("slots=$slots")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = activeContexts?.hashCode() ?: 0
result = 31 * result + (audioStream?.hashCode() ?: 0)
result = 31 * result + (contentType?.hashCode() ?: 0)
result = 31 * result + (dialogState?.hashCode() ?: 0)
result = 31 * result + (encodedMessage?.hashCode() ?: 0)
result = 31 * result + (intentName?.hashCode() ?: 0)
result = 31 * result + (message?.hashCode() ?: 0)
result = 31 * result + (messageFormat?.hashCode() ?: 0)
result = 31 * result + (sessionAttributes?.hashCode() ?: 0)
result = 31 * result + (sessionId?.hashCode() ?: 0)
result = 31 * result + (slotToElicit?.hashCode() ?: 0)
result = 31 * result + (slots?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PutSessionResponse
if (activeContexts != other.activeContexts) return false
if (audioStream != other.audioStream) return false
if (contentType != other.contentType) return false
if (dialogState != other.dialogState) return false
if (encodedMessage != other.encodedMessage) return false
if (intentName != other.intentName) return false
if (message != other.message) return false
if (messageFormat != other.messageFormat) return false
if (sessionAttributes != other.sessionAttributes) return false
if (sessionId != other.sessionId) return false
if (slotToElicit != other.slotToElicit) return false
if (slots != other.slots) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A list of active contexts for the session.
*/
public var activeContexts: kotlin.String? = null
/**
* The audio version of the message to convey to the user.
*/
public var audioStream: aws.smithy.kotlin.runtime.content.ByteStream? = null
/**
* Content type as specified in the `Accept` HTTP header in the request.
*/
public var contentType: kotlin.String? = null
/**
* + `ConfirmIntent` - Amazon Lex is expecting a "yes" or "no" response to confirm the intent before fulfilling an intent.
* + `ElicitIntent` - Amazon Lex wants to elicit the user's intent.
* + `ElicitSlot` - Amazon Lex is expecting the value of a slot for the current intent.
* + `Failed` - Conveys that the conversation with the user has failed. This can happen for various reasons, including the user does not provide an appropriate response to prompts from the service, or if the Lambda function fails to fulfill the intent.
* + `Fulfilled` - Conveys that the Lambda function has sucessfully fulfilled the intent.
* + `ReadyForFulfillment` - Conveys that the client has to fulfill the intent.
*/
public var dialogState: aws.sdk.kotlin.services.lexruntimeservice.model.DialogState? = null
/**
* The next message that should be presented to the user.
*
* The `encodedMessage` field is base-64 encoded. You must decode the field before you can use the value.
*/
public var encodedMessage: kotlin.String? = null
/**
* The name of the current intent.
*/
public var intentName: kotlin.String? = null
/**
* The next message that should be presented to the user.
*
* You can only use this field in the de-DE, en-AU, en-GB, en-US, es-419, es-ES, es-US, fr-CA, fr-FR, and it-IT locales. In all other locales, the `message` field is null. You should use the `encodedMessage` field instead.
*/
@Deprecated("The message field is deprecated, use the encodedMessage field instead. The message field is available only in the de-DE, en-AU, en-GB, en-US, es-419, es-ES, es-US, fr-CA, fr-FR and it-IT locales.")
public var message: kotlin.String? = null
/**
* The format of the response message. One of the following values:
* + `PlainText` - The message contains plain UTF-8 text.
* + `CustomPayload` - The message is a custom format for the client.
* + `SSML` - The message contains text formatted for voice output.
* + `Composite` - The message contains an escaped JSON object containing one or more messages from the groups that messages were assigned to when the intent was created.
*/
public var messageFormat: aws.sdk.kotlin.services.lexruntimeservice.model.MessageFormatType? = null
/**
* Map of key/value pairs representing session-specific context information.
*/
public var sessionAttributes: kotlin.String? = null
/**
* A unique identifier for the session.
*/
public var sessionId: kotlin.String? = null
/**
* If the `dialogState` is `ElicitSlot`, returns the name of the slot for which Amazon Lex is eliciting a value.
*/
public var slotToElicit: kotlin.String? = null
/**
* Map of zero or more intent slots Amazon Lex detected from the user input during the conversation.
*
* Amazon Lex creates a resolution list containing likely values for a slot. The value that it returns is determined by the `valueSelectionStrategy` selected when the slot type was created or updated. If `valueSelectionStrategy` is set to `ORIGINAL_VALUE`, the value provided by the user is returned, if the user value is similar to the slot values. If `valueSelectionStrategy` is set to `TOP_RESOLUTION` Amazon Lex returns the first value in the resolution list or, if there is no resolution list, null. If you don't specify a `valueSelectionStrategy` the default is `ORIGINAL_VALUE`.
*/
public var slots: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionResponse) : this() {
this.activeContexts = x.activeContexts
this.audioStream = x.audioStream
this.contentType = x.contentType
this.dialogState = x.dialogState
this.encodedMessage = x.encodedMessage
this.intentName = x.intentName
this.message = x.message
this.messageFormat = x.messageFormat
this.sessionAttributes = x.sessionAttributes
this.sessionId = x.sessionId
this.slotToElicit = x.slotToElicit
this.slots = x.slots
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexruntimeservice.model.PutSessionResponse = PutSessionResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy