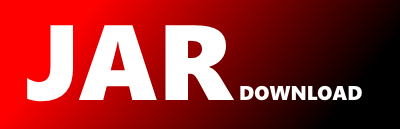
commonMain.aws.sdk.kotlin.services.lexruntimeservice.model.GetSessionResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexruntimeservice.model
import aws.smithy.kotlin.runtime.SdkDsl
public class GetSessionResponse private constructor(builder: Builder) {
/**
* A list of active contexts for the session. A context can be set when an intent is fulfilled or by calling the `PostContent`, `PostText`, or `PutSession` operation.
*
* You can use a context to control the intents that can follow up an intent, or to modify the operation of your application.
*/
public val activeContexts: List? = builder.activeContexts
/**
* Describes the current state of the bot.
*/
public val dialogAction: aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction? = builder.dialogAction
/**
* An array of information about the intents used in the session. The array can contain a maximum of three summaries. If more than three intents are used in the session, the `recentIntentSummaryView` operation contains information about the last three intents used.
*
* If you set the `checkpointLabelFilter` parameter in the request, the array contains only the intents with the specified label.
*/
public val recentIntentSummaryView: List? = builder.recentIntentSummaryView
/**
* Map of key/value pairs representing the session-specific context information. It contains application information passed between Amazon Lex and a client application.
*/
public val sessionAttributes: Map? = builder.sessionAttributes
/**
* A unique identifier for the session.
*/
public val sessionId: kotlin.String? = builder.sessionId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexruntimeservice.model.GetSessionResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetSessionResponse(")
append("activeContexts=*** Sensitive Data Redacted ***,")
append("dialogAction=$dialogAction,")
append("recentIntentSummaryView=$recentIntentSummaryView,")
append("sessionAttributes=*** Sensitive Data Redacted ***,")
append("sessionId=$sessionId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = activeContexts?.hashCode() ?: 0
result = 31 * result + (dialogAction?.hashCode() ?: 0)
result = 31 * result + (recentIntentSummaryView?.hashCode() ?: 0)
result = 31 * result + (sessionAttributes?.hashCode() ?: 0)
result = 31 * result + (sessionId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetSessionResponse
if (activeContexts != other.activeContexts) return false
if (dialogAction != other.dialogAction) return false
if (recentIntentSummaryView != other.recentIntentSummaryView) return false
if (sessionAttributes != other.sessionAttributes) return false
if (sessionId != other.sessionId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexruntimeservice.model.GetSessionResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A list of active contexts for the session. A context can be set when an intent is fulfilled or by calling the `PostContent`, `PostText`, or `PutSession` operation.
*
* You can use a context to control the intents that can follow up an intent, or to modify the operation of your application.
*/
public var activeContexts: List? = null
/**
* Describes the current state of the bot.
*/
public var dialogAction: aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction? = null
/**
* An array of information about the intents used in the session. The array can contain a maximum of three summaries. If more than three intents are used in the session, the `recentIntentSummaryView` operation contains information about the last three intents used.
*
* If you set the `checkpointLabelFilter` parameter in the request, the array contains only the intents with the specified label.
*/
public var recentIntentSummaryView: List? = null
/**
* Map of key/value pairs representing the session-specific context information. It contains application information passed between Amazon Lex and a client application.
*/
public var sessionAttributes: Map? = null
/**
* A unique identifier for the session.
*/
public var sessionId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexruntimeservice.model.GetSessionResponse) : this() {
this.activeContexts = x.activeContexts
this.dialogAction = x.dialogAction
this.recentIntentSummaryView = x.recentIntentSummaryView
this.sessionAttributes = x.sessionAttributes
this.sessionId = x.sessionId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexruntimeservice.model.GetSessionResponse = GetSessionResponse(this)
/**
* construct an [aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction] inside the given [block]
*/
public fun dialogAction(block: aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction.Builder.() -> kotlin.Unit) {
this.dialogAction = aws.sdk.kotlin.services.lexruntimeservice.model.DialogAction.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy