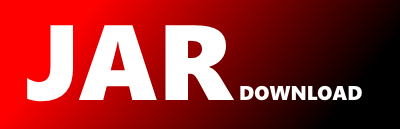
commonMain.aws.sdk.kotlin.services.lexruntimeservice.model.PostContentRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lexruntimeservice-jvm Show documentation
Show all versions of lexruntimeservice-jvm Show documentation
The AWS Kotlin client for Lex Runtime Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lexruntimeservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.content.ByteStream
public class PostContentRequest private constructor(builder: Builder) {
/**
* You pass this value as the `Accept` HTTP header.
*
* The message Amazon Lex returns in the response can be either text or speech based on the `Accept` HTTP header value in the request.
* + If the value is `text/plain; charset=utf-8`, Amazon Lex returns text in the response.
* + If the value begins with `audio/`, Amazon Lex returns speech in the response. Amazon Lex uses Amazon Polly to generate the speech (using the configuration you specified in the `Accept` header). For example, if you specify `audio/mpeg` as the value, Amazon Lex returns speech in the MPEG format.
* + If the value is `audio/pcm`, the speech returned is `audio/pcm` in 16-bit, little endian format.
* + The following are the accepted values:
* + audio/mpeg
* + audio/ogg
* + audio/pcm
* + text/plain; charset=utf-8
* + audio/* (defaults to mpeg)
*/
public val accept: kotlin.String? = builder.accept
/**
* A list of contexts active for the request. A context can be activated when a previous intent is fulfilled, or by including the context in the request,
*
* If you don't specify a list of contexts, Amazon Lex will use the current list of contexts for the session. If you specify an empty list, all contexts for the session are cleared.
*/
public val activeContexts: kotlin.String? = builder.activeContexts
/**
* Alias of the Amazon Lex bot.
*/
public val botAlias: kotlin.String? = builder.botAlias
/**
* Name of the Amazon Lex bot.
*/
public val botName: kotlin.String? = builder.botName
/**
* You pass this value as the `Content-Type` HTTP header.
*
* Indicates the audio format or text. The header value must start with one of the following prefixes:
* + PCM format, audio data must be in little-endian byte order.
* + audio/l16; rate=16000; channels=1
* + audio/x-l16; sample-rate=16000; channel-count=1
* + audio/lpcm; sample-rate=8000; sample-size-bits=16; channel-count=1; is-big-endian=false
* + Opus format
* + audio/x-cbr-opus-with-preamble; preamble-size=0; bit-rate=256000; frame-size-milliseconds=4
* + Text format
* + text/plain; charset=utf-8
*/
public val contentType: kotlin.String? = builder.contentType
/**
* User input in PCM or Opus audio format or text format as described in the `Content-Type` HTTP header.
*
* You can stream audio data to Amazon Lex or you can create a local buffer that captures all of the audio data before sending. In general, you get better performance if you stream audio data rather than buffering the data locally.
*/
public val inputStream: aws.smithy.kotlin.runtime.content.ByteStream? = builder.inputStream
/**
* You pass this value as the `x-amz-lex-request-attributes` HTTP header.
*
* Request-specific information passed between Amazon Lex and a client application. The value must be a JSON serialized and base64 encoded map with string keys and values. The total size of the `requestAttributes` and `sessionAttributes` headers is limited to 12 KB.
*
* The namespace `x-amz-lex:` is reserved for special attributes. Don't create any request attributes with the prefix `x-amz-lex:`.
*
* For more information, see [Setting Request Attributes](https://docs.aws.amazon.com/lex/latest/dg/context-mgmt.html#context-mgmt-request-attribs).
*/
public val requestAttributes: kotlin.String? = builder.requestAttributes
/**
* You pass this value as the `x-amz-lex-session-attributes` HTTP header.
*
* Application-specific information passed between Amazon Lex and a client application. The value must be a JSON serialized and base64 encoded map with string keys and values. The total size of the `sessionAttributes` and `requestAttributes` headers is limited to 12 KB.
*
* For more information, see [Setting Session Attributes](https://docs.aws.amazon.com/lex/latest/dg/context-mgmt.html#context-mgmt-session-attribs).
*/
public val sessionAttributes: kotlin.String? = builder.sessionAttributes
/**
* The ID of the client application user. Amazon Lex uses this to identify a user's conversation with your bot. At runtime, each request must contain the `userID` field.
*
* To decide the user ID to use for your application, consider the following factors.
* + The `userID` field must not contain any personally identifiable information of the user, for example, name, personal identification numbers, or other end user personal information.
* + If you want a user to start a conversation on one device and continue on another device, use a user-specific identifier.
* + If you want the same user to be able to have two independent conversations on two different devices, choose a device-specific identifier.
* + A user can't have two independent conversations with two different versions of the same bot. For example, a user can't have a conversation with the PROD and BETA versions of the same bot. If you anticipate that a user will need to have conversation with two different versions, for example, while testing, include the bot alias in the user ID to separate the two conversations.
*/
public val userId: kotlin.String? = builder.userId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lexruntimeservice.model.PostContentRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PostContentRequest(")
append("accept=$accept,")
append("activeContexts=*** Sensitive Data Redacted ***,")
append("botAlias=$botAlias,")
append("botName=$botName,")
append("contentType=$contentType,")
append("inputStream=$inputStream,")
append("requestAttributes=*** Sensitive Data Redacted ***,")
append("sessionAttributes=*** Sensitive Data Redacted ***,")
append("userId=$userId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accept?.hashCode() ?: 0
result = 31 * result + (activeContexts?.hashCode() ?: 0)
result = 31 * result + (botAlias?.hashCode() ?: 0)
result = 31 * result + (botName?.hashCode() ?: 0)
result = 31 * result + (contentType?.hashCode() ?: 0)
result = 31 * result + (inputStream?.hashCode() ?: 0)
result = 31 * result + (requestAttributes?.hashCode() ?: 0)
result = 31 * result + (sessionAttributes?.hashCode() ?: 0)
result = 31 * result + (userId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PostContentRequest
if (accept != other.accept) return false
if (activeContexts != other.activeContexts) return false
if (botAlias != other.botAlias) return false
if (botName != other.botName) return false
if (contentType != other.contentType) return false
if (inputStream != other.inputStream) return false
if (requestAttributes != other.requestAttributes) return false
if (sessionAttributes != other.sessionAttributes) return false
if (userId != other.userId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lexruntimeservice.model.PostContentRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* You pass this value as the `Accept` HTTP header.
*
* The message Amazon Lex returns in the response can be either text or speech based on the `Accept` HTTP header value in the request.
* + If the value is `text/plain; charset=utf-8`, Amazon Lex returns text in the response.
* + If the value begins with `audio/`, Amazon Lex returns speech in the response. Amazon Lex uses Amazon Polly to generate the speech (using the configuration you specified in the `Accept` header). For example, if you specify `audio/mpeg` as the value, Amazon Lex returns speech in the MPEG format.
* + If the value is `audio/pcm`, the speech returned is `audio/pcm` in 16-bit, little endian format.
* + The following are the accepted values:
* + audio/mpeg
* + audio/ogg
* + audio/pcm
* + text/plain; charset=utf-8
* + audio/* (defaults to mpeg)
*/
public var accept: kotlin.String? = null
/**
* A list of contexts active for the request. A context can be activated when a previous intent is fulfilled, or by including the context in the request,
*
* If you don't specify a list of contexts, Amazon Lex will use the current list of contexts for the session. If you specify an empty list, all contexts for the session are cleared.
*/
public var activeContexts: kotlin.String? = null
/**
* Alias of the Amazon Lex bot.
*/
public var botAlias: kotlin.String? = null
/**
* Name of the Amazon Lex bot.
*/
public var botName: kotlin.String? = null
/**
* You pass this value as the `Content-Type` HTTP header.
*
* Indicates the audio format or text. The header value must start with one of the following prefixes:
* + PCM format, audio data must be in little-endian byte order.
* + audio/l16; rate=16000; channels=1
* + audio/x-l16; sample-rate=16000; channel-count=1
* + audio/lpcm; sample-rate=8000; sample-size-bits=16; channel-count=1; is-big-endian=false
* + Opus format
* + audio/x-cbr-opus-with-preamble; preamble-size=0; bit-rate=256000; frame-size-milliseconds=4
* + Text format
* + text/plain; charset=utf-8
*/
public var contentType: kotlin.String? = null
/**
* User input in PCM or Opus audio format or text format as described in the `Content-Type` HTTP header.
*
* You can stream audio data to Amazon Lex or you can create a local buffer that captures all of the audio data before sending. In general, you get better performance if you stream audio data rather than buffering the data locally.
*/
public var inputStream: aws.smithy.kotlin.runtime.content.ByteStream? = null
/**
* You pass this value as the `x-amz-lex-request-attributes` HTTP header.
*
* Request-specific information passed between Amazon Lex and a client application. The value must be a JSON serialized and base64 encoded map with string keys and values. The total size of the `requestAttributes` and `sessionAttributes` headers is limited to 12 KB.
*
* The namespace `x-amz-lex:` is reserved for special attributes. Don't create any request attributes with the prefix `x-amz-lex:`.
*
* For more information, see [Setting Request Attributes](https://docs.aws.amazon.com/lex/latest/dg/context-mgmt.html#context-mgmt-request-attribs).
*/
public var requestAttributes: kotlin.String? = null
/**
* You pass this value as the `x-amz-lex-session-attributes` HTTP header.
*
* Application-specific information passed between Amazon Lex and a client application. The value must be a JSON serialized and base64 encoded map with string keys and values. The total size of the `sessionAttributes` and `requestAttributes` headers is limited to 12 KB.
*
* For more information, see [Setting Session Attributes](https://docs.aws.amazon.com/lex/latest/dg/context-mgmt.html#context-mgmt-session-attribs).
*/
public var sessionAttributes: kotlin.String? = null
/**
* The ID of the client application user. Amazon Lex uses this to identify a user's conversation with your bot. At runtime, each request must contain the `userID` field.
*
* To decide the user ID to use for your application, consider the following factors.
* + The `userID` field must not contain any personally identifiable information of the user, for example, name, personal identification numbers, or other end user personal information.
* + If you want a user to start a conversation on one device and continue on another device, use a user-specific identifier.
* + If you want the same user to be able to have two independent conversations on two different devices, choose a device-specific identifier.
* + A user can't have two independent conversations with two different versions of the same bot. For example, a user can't have a conversation with the PROD and BETA versions of the same bot. If you anticipate that a user will need to have conversation with two different versions, for example, while testing, include the bot alias in the user ID to separate the two conversations.
*/
public var userId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lexruntimeservice.model.PostContentRequest) : this() {
this.accept = x.accept
this.activeContexts = x.activeContexts
this.botAlias = x.botAlias
this.botName = x.botName
this.contentType = x.contentType
this.inputStream = x.inputStream
this.requestAttributes = x.requestAttributes
this.sessionAttributes = x.sessionAttributes
this.userId = x.userId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lexruntimeservice.model.PostContentRequest = PostContentRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy