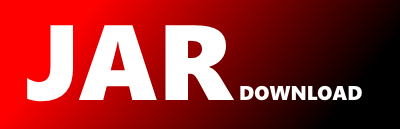
commonMain.aws.sdk.kotlin.services.lookoutmetrics.model.DescribeAnomalyDetectorResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lookoutmetrics-jvm Show documentation
Show all versions of lookoutmetrics-jvm Show documentation
The AWS SDK for Kotlin client for LookoutMetrics
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lookoutmetrics.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeAnomalyDetectorResponse private constructor(builder: Builder) {
/**
* The ARN of the detector.
*/
public val anomalyDetectorArn: kotlin.String? = builder.anomalyDetectorArn
/**
* Contains information about the detector's configuration.
*/
public val anomalyDetectorConfig: aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorConfigSummary? = builder.anomalyDetectorConfig
/**
* A description of the detector.
*/
public val anomalyDetectorDescription: kotlin.String? = builder.anomalyDetectorDescription
/**
* The name of the detector.
*/
public val anomalyDetectorName: kotlin.String? = builder.anomalyDetectorName
/**
* The time at which the detector was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The reason that the detector failed.
*/
public val failureReason: kotlin.String? = builder.failureReason
/**
* The process that caused the detector to fail.
*/
public val failureType: aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorFailureType? = builder.failureType
/**
* The ARN of the KMS key to use to encrypt your data.
*/
public val kmsKeyArn: kotlin.String? = builder.kmsKeyArn
/**
* The time at which the detector was last modified.
*/
public val lastModificationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModificationTime
/**
* The status of the detector.
*/
public val status: aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorStatus? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lookoutmetrics.model.DescribeAnomalyDetectorResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeAnomalyDetectorResponse(")
append("anomalyDetectorArn=$anomalyDetectorArn,")
append("anomalyDetectorConfig=$anomalyDetectorConfig,")
append("anomalyDetectorDescription=$anomalyDetectorDescription,")
append("anomalyDetectorName=$anomalyDetectorName,")
append("creationTime=$creationTime,")
append("failureReason=$failureReason,")
append("failureType=$failureType,")
append("kmsKeyArn=$kmsKeyArn,")
append("lastModificationTime=$lastModificationTime,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = anomalyDetectorArn?.hashCode() ?: 0
result = 31 * result + (anomalyDetectorConfig?.hashCode() ?: 0)
result = 31 * result + (anomalyDetectorDescription?.hashCode() ?: 0)
result = 31 * result + (anomalyDetectorName?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (failureType?.hashCode() ?: 0)
result = 31 * result + (kmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (lastModificationTime?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeAnomalyDetectorResponse
if (anomalyDetectorArn != other.anomalyDetectorArn) return false
if (anomalyDetectorConfig != other.anomalyDetectorConfig) return false
if (anomalyDetectorDescription != other.anomalyDetectorDescription) return false
if (anomalyDetectorName != other.anomalyDetectorName) return false
if (creationTime != other.creationTime) return false
if (failureReason != other.failureReason) return false
if (failureType != other.failureType) return false
if (kmsKeyArn != other.kmsKeyArn) return false
if (lastModificationTime != other.lastModificationTime) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lookoutmetrics.model.DescribeAnomalyDetectorResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARN of the detector.
*/
public var anomalyDetectorArn: kotlin.String? = null
/**
* Contains information about the detector's configuration.
*/
public var anomalyDetectorConfig: aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorConfigSummary? = null
/**
* A description of the detector.
*/
public var anomalyDetectorDescription: kotlin.String? = null
/**
* The name of the detector.
*/
public var anomalyDetectorName: kotlin.String? = null
/**
* The time at which the detector was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The reason that the detector failed.
*/
public var failureReason: kotlin.String? = null
/**
* The process that caused the detector to fail.
*/
public var failureType: aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorFailureType? = null
/**
* The ARN of the KMS key to use to encrypt your data.
*/
public var kmsKeyArn: kotlin.String? = null
/**
* The time at which the detector was last modified.
*/
public var lastModificationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The status of the detector.
*/
public var status: aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lookoutmetrics.model.DescribeAnomalyDetectorResponse) : this() {
this.anomalyDetectorArn = x.anomalyDetectorArn
this.anomalyDetectorConfig = x.anomalyDetectorConfig
this.anomalyDetectorDescription = x.anomalyDetectorDescription
this.anomalyDetectorName = x.anomalyDetectorName
this.creationTime = x.creationTime
this.failureReason = x.failureReason
this.failureType = x.failureType
this.kmsKeyArn = x.kmsKeyArn
this.lastModificationTime = x.lastModificationTime
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lookoutmetrics.model.DescribeAnomalyDetectorResponse = DescribeAnomalyDetectorResponse(this)
/**
* construct an [aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorConfigSummary] inside the given [block]
*/
public fun anomalyDetectorConfig(block: aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorConfigSummary.Builder.() -> kotlin.Unit) {
this.anomalyDetectorConfig = aws.sdk.kotlin.services.lookoutmetrics.model.AnomalyDetectorConfigSummary.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy