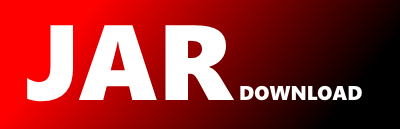
commonMain.aws.sdk.kotlin.services.lookoutmetrics.model.DescribeMetricSetResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lookoutmetrics-jvm Show documentation
Show all versions of lookoutmetrics-jvm Show documentation
The AWS SDK for Kotlin client for LookoutMetrics
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.lookoutmetrics.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeMetricSetResponse private constructor(builder: Builder) {
/**
* The ARN of the detector that contains the dataset.
*/
public val anomalyDetectorArn: kotlin.String? = builder.anomalyDetectorArn
/**
* The time at which the dataset was created.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* The dimensions and their values that were used to filter the dataset.
*/
public val dimensionFilterList: List? = builder.dimensionFilterList
/**
* A list of the dimensions chosen for analysis.
*/
public val dimensionList: List? = builder.dimensionList
/**
* The time at which the dataset was last modified.
*/
public val lastModificationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModificationTime
/**
* A list of the metrics defined by the dataset.
*/
public val metricList: List? = builder.metricList
/**
* The ARN of the dataset.
*/
public val metricSetArn: kotlin.String? = builder.metricSetArn
/**
* The dataset's description.
*/
public val metricSetDescription: kotlin.String? = builder.metricSetDescription
/**
* The interval at which the data will be analyzed for anomalies.
*/
public val metricSetFrequency: aws.sdk.kotlin.services.lookoutmetrics.model.Frequency? = builder.metricSetFrequency
/**
* The name of the dataset.
*/
public val metricSetName: kotlin.String? = builder.metricSetName
/**
* Contains information about the dataset's source data.
*/
public val metricSource: aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource? = builder.metricSource
/**
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is only supported for S3, Redshift, Athena and datasources.
*/
public val offset: kotlin.Int? = builder.offset
/**
* Contains information about the column used for tracking time in your source data.
*/
public val timestampColumn: aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn? = builder.timestampColumn
/**
* The time zone in which the dataset's data was recorded.
*/
public val timezone: kotlin.String? = builder.timezone
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.lookoutmetrics.model.DescribeMetricSetResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeMetricSetResponse(")
append("anomalyDetectorArn=$anomalyDetectorArn,")
append("creationTime=$creationTime,")
append("dimensionFilterList=$dimensionFilterList,")
append("dimensionList=$dimensionList,")
append("lastModificationTime=$lastModificationTime,")
append("metricList=$metricList,")
append("metricSetArn=$metricSetArn,")
append("metricSetDescription=$metricSetDescription,")
append("metricSetFrequency=$metricSetFrequency,")
append("metricSetName=$metricSetName,")
append("metricSource=$metricSource,")
append("offset=$offset,")
append("timestampColumn=$timestampColumn,")
append("timezone=$timezone")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = anomalyDetectorArn?.hashCode() ?: 0
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (dimensionFilterList?.hashCode() ?: 0)
result = 31 * result + (dimensionList?.hashCode() ?: 0)
result = 31 * result + (lastModificationTime?.hashCode() ?: 0)
result = 31 * result + (metricList?.hashCode() ?: 0)
result = 31 * result + (metricSetArn?.hashCode() ?: 0)
result = 31 * result + (metricSetDescription?.hashCode() ?: 0)
result = 31 * result + (metricSetFrequency?.hashCode() ?: 0)
result = 31 * result + (metricSetName?.hashCode() ?: 0)
result = 31 * result + (metricSource?.hashCode() ?: 0)
result = 31 * result + (offset ?: 0)
result = 31 * result + (timestampColumn?.hashCode() ?: 0)
result = 31 * result + (timezone?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeMetricSetResponse
if (anomalyDetectorArn != other.anomalyDetectorArn) return false
if (creationTime != other.creationTime) return false
if (dimensionFilterList != other.dimensionFilterList) return false
if (dimensionList != other.dimensionList) return false
if (lastModificationTime != other.lastModificationTime) return false
if (metricList != other.metricList) return false
if (metricSetArn != other.metricSetArn) return false
if (metricSetDescription != other.metricSetDescription) return false
if (metricSetFrequency != other.metricSetFrequency) return false
if (metricSetName != other.metricSetName) return false
if (metricSource != other.metricSource) return false
if (offset != other.offset) return false
if (timestampColumn != other.timestampColumn) return false
if (timezone != other.timezone) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.lookoutmetrics.model.DescribeMetricSetResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARN of the detector that contains the dataset.
*/
public var anomalyDetectorArn: kotlin.String? = null
/**
* The time at which the dataset was created.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The dimensions and their values that were used to filter the dataset.
*/
public var dimensionFilterList: List? = null
/**
* A list of the dimensions chosen for analysis.
*/
public var dimensionList: List? = null
/**
* The time at which the dataset was last modified.
*/
public var lastModificationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A list of the metrics defined by the dataset.
*/
public var metricList: List? = null
/**
* The ARN of the dataset.
*/
public var metricSetArn: kotlin.String? = null
/**
* The dataset's description.
*/
public var metricSetDescription: kotlin.String? = null
/**
* The interval at which the data will be analyzed for anomalies.
*/
public var metricSetFrequency: aws.sdk.kotlin.services.lookoutmetrics.model.Frequency? = null
/**
* The name of the dataset.
*/
public var metricSetName: kotlin.String? = null
/**
* Contains information about the dataset's source data.
*/
public var metricSource: aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource? = null
/**
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is only supported for S3, Redshift, Athena and datasources.
*/
public var offset: kotlin.Int? = null
/**
* Contains information about the column used for tracking time in your source data.
*/
public var timestampColumn: aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn? = null
/**
* The time zone in which the dataset's data was recorded.
*/
public var timezone: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.lookoutmetrics.model.DescribeMetricSetResponse) : this() {
this.anomalyDetectorArn = x.anomalyDetectorArn
this.creationTime = x.creationTime
this.dimensionFilterList = x.dimensionFilterList
this.dimensionList = x.dimensionList
this.lastModificationTime = x.lastModificationTime
this.metricList = x.metricList
this.metricSetArn = x.metricSetArn
this.metricSetDescription = x.metricSetDescription
this.metricSetFrequency = x.metricSetFrequency
this.metricSetName = x.metricSetName
this.metricSource = x.metricSource
this.offset = x.offset
this.timestampColumn = x.timestampColumn
this.timezone = x.timezone
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.lookoutmetrics.model.DescribeMetricSetResponse = DescribeMetricSetResponse(this)
/**
* construct an [aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource] inside the given [block]
*/
public fun metricSource(block: aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource.Builder.() -> kotlin.Unit) {
this.metricSource = aws.sdk.kotlin.services.lookoutmetrics.model.MetricSource.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn] inside the given [block]
*/
public fun timestampColumn(block: aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn.Builder.() -> kotlin.Unit) {
this.timestampColumn = aws.sdk.kotlin.services.lookoutmetrics.model.TimestampColumn.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy